Hi Guys Welcome to Proto Coders Point, In this Android Tutorial we will learn how to implement password validation regex requirements in android app.
In other words Password Validation To check a password character must be minimum 8 character length which include alphebet, numeric digits and any special character symbol.
Password Validation requirements
- Atleast 8 character in length.
- Minimum One Uppercase.
- Minimum One Number.
- and should contain 1 Special Symbol.
Password Validation regex in android
Video Tutorial
Follow the below Steps and Code to integrate this validation textfield in android.
Step 1 : Create a new android project
offcourse you need to create a new Android Project or Open any existing project in android studio.
File > New > New Project > Empty Activity
Give a name to android project as “Password Validation android” or anything else as per your choice.
hit next > finish.
Step 2 : Add Material Design Dependencies
Open build.gradle(app level) and add the below android design dependencies.
implementation 'com.android.support:design:29.0.0'
We are making use of android design library dependencies so that we can use material.textfield.TextInputLayout and androidx.cardview.widget.CardView in our android UI design.
Step 3 : Creating a Registration page UI
Open activity_main.xml file and copy page the below xml UI code.
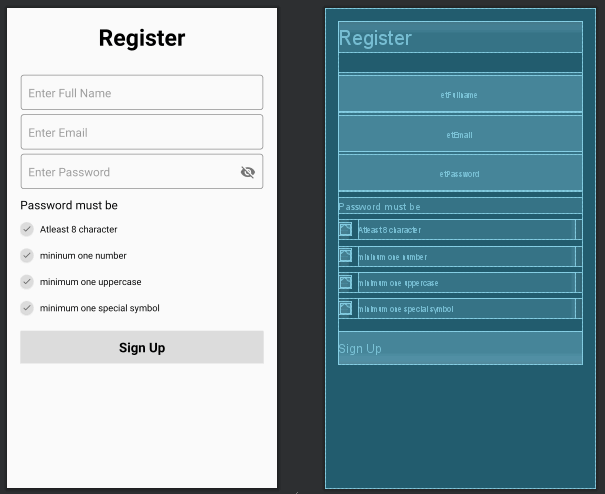
<?xml version="1.0" encoding="utf-8"?> <androidx.core.widget.NestedScrollView xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:layout_margin="20dp" android:orientation="vertical" > <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="35sp" android:textStyle="bold" android:text="Register" android:textColor="@android:color/black" android:gravity="center" android:layout_marginBottom="30dp" /> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="60dp" style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox.Dense"> <EditText android:id="@+id/etFullname" android:layout_width="match_parent" android:layout_height="match_parent" android:hint="Enter Full Name" android:inputType="text" android:textColor="@android:color/black" /> </com.google.android.material.textfield.TextInputLayout> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="60dp" style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox.Dense"> <EditText android:id="@+id/etEmail" android:layout_width="match_parent" android:layout_height="match_parent" android:hint="Enter Email" android:inputType="text" android:textColor="@android:color/black" /> </com.google.android.material.textfield.TextInputLayout> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="60dp" app:passwordToggleEnabled="true" style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox.Dense"> <EditText android:id="@+id/etPassword" android:layout_width="match_parent" android:layout_height="match_parent" android:hint="Enter Password" android:inputType="textPassword" android:textColor="@android:color/black" /> </com.google.android.material.textfield.TextInputLayout> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Password must be " android:layout_marginTop="10dp" android:textColor="@android:color/black" android:textSize="18sp" /> <LinearLayout android:layout_width="match_parent" android:layout_height="30dp" android:layout_marginTop="10dp" android:gravity="center" android:orientation="horizontal"> <androidx.cardview.widget.CardView android:id="@+id/card1" android:layout_width="20dp" android:layout_height="20dp" app:cardBackgroundColor="#dcdcdc" app:cardCornerRadius="25dp" android:layout_gravity="center"> <ImageView android:layout_gravity="center" android:layout_width="15dp" android:layout_height="15dp" android:background="@drawable/check_24" /> </androidx.cardview.widget.CardView> <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:textColor="@android:color/black" android:text="Atleast 8 character" android:gravity="center|start" android:layout_marginStart="10dp" android:layout_marginEnd="10dp" android:layout_marginLeft="10dp" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="30dp" android:layout_marginTop="10dp" android:gravity="center" android:orientation="horizontal"> <androidx.cardview.widget.CardView android:id="@+id/card2" android:layout_width="20dp" android:layout_height="20dp" app:cardBackgroundColor="#dcdcdc" app:cardCornerRadius="25dp" android:layout_gravity="center"> <ImageView android:layout_gravity="center" android:layout_width="15dp" android:layout_height="15dp" android:background="@drawable/check_24" /> </androidx.cardview.widget.CardView> <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:textColor="@android:color/black" android:text="mininum one number" android:gravity="center|start" android:layout_marginStart="10dp" android:layout_marginEnd="10dp" android:layout_marginLeft="10dp" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="30dp" android:layout_marginTop="10dp" android:gravity="center" android:orientation="horizontal"> <androidx.cardview.widget.CardView android:id="@+id/card3" android:layout_width="20dp" android:layout_height="20dp" app:cardBackgroundColor="#dcdcdc" app:cardCornerRadius="25dp" android:layout_gravity="center"> <ImageView android:layout_gravity="center" android:layout_width="15dp" android:layout_height="15dp" android:background="@drawable/check_24" /> </androidx.cardview.widget.CardView> <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:textColor="@android:color/black" android:text="minimum one uppercase" android:gravity="center|start" android:layout_marginStart="10dp" android:layout_marginEnd="10dp" android:layout_marginLeft="10dp" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="30dp" android:layout_marginTop="10dp" android:gravity="center" android:orientation="horizontal"> <androidx.cardview.widget.CardView android:id="@+id/card4" android:layout_width="20dp" android:layout_height="20dp" app:cardBackgroundColor="#dcdcdc" app:cardCornerRadius="25dp" android:layout_gravity="center"> <ImageView android:layout_gravity="center" android:layout_width="15dp" android:layout_height="15dp" android:background="@drawable/check_24" /> </androidx.cardview.widget.CardView> <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:textColor="@android:color/black" android:text="minimum one special symbol" android:gravity="center|start" android:layout_marginStart="10dp" android:layout_marginEnd="10dp" android:layout_marginLeft="10dp" /> </LinearLayout> <androidx.cardview.widget.CardView android:id="@+id/cardsignup" android:layout_width="match_parent" android:layout_height="50dp" android:layout_marginTop="20sp" app:cardBackgroundColor="#dcdcdc"> <TextView android:layout_width="match_parent" android:layout_height="match_parent" android:textSize="20sp" android:textStyle="bold" android:text="Sign Up" android:textColor="@android:color/black" android:gravity="center"/> </androidx.cardview.widget.CardView> </LinearLayout> </androidx.core.widget.NestedScrollView>
As you can see in above Ui Screenshot we have 3 EditText, 4 Cardview with a tick Icon and a Button.
Step 4 : Java Coding to Handle password Validation
In Below Java code we have 2 method one to check passwordValidation() and other to keep track of InputChanged() in TextField.
MainActivity.java
package com.example.passwordcharactervalidation; import android.annotation.SuppressLint; import android.graphics.Color; import android.os.Bundle; import android.text.Editable; import android.text.TextWatcher; import android.view.View; import android.widget.EditText; import android.widget.TextView; import androidx.annotation.Nullable; import androidx.appcompat.app.AppCompatActivity; import androidx.cardview.widget.CardView; public class MainActivity extends AppCompatActivity { EditText etfullname,etEmail,etPassword; CardView card1,card2,card3,card4,cardButtonSignUp; private boolean is8char=false, hasUpper=false, hasnum=false, hasSpecialSymbol =false, isSignupClickable = false; @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); etfullname=(EditText) findViewById(R.id.etFullname); etEmail = (EditText)findViewById(R.id.etEmail); etPassword = (EditText)findViewById(R.id.etPassword); card1 = (CardView)findViewById(R.id.card1); card2 = (CardView)findViewById(R.id.card2); card3 = (CardView)findViewById(R.id.card3); card4 = (CardView)findViewById(R.id.card4); cardButtonSignUp = (CardView)findViewById(R.id.cardsignup); cardButtonSignUp.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { } }); inputChanged(); } @SuppressLint("ResourceType") private void passwordValidate(){ String name = etfullname.getText().toString(); String email = etEmail.getText().toString(); String password = etPassword.getText().toString(); if(name.isEmpty()) { etfullname.setError("Please Enter Full name "); } if(email.isEmpty()) { etEmail.setError("Please Enter Email "); } // 8 character if(password.length()>= 8) { is8char = true; card1.setCardBackgroundColor(Color.parseColor(getString(R.color.colorAccent))); }else{ is8char = false; card1.setCardBackgroundColor(Color.parseColor(getString(R.color.colorGrey))); } //number if(password.matches("(.*[0-9].*)")) { hasnum = true; card2.setCardBackgroundColor(Color.parseColor(getString(R.color.colorAccent))); }else{ hasUpper = false; card2.setCardBackgroundColor(Color.parseColor(getString(R.color.colorGrey))); } //upper case if(password.matches("(.*[A-Z].*)")) { hasUpper = true; card3.setCardBackgroundColor(Color.parseColor(getString(R.color.colorAccent))); }else{ hasUpper = false; card3.setCardBackgroundColor(Color.parseColor(getString(R.color.colorGrey))); } //symbol if(password.matches("^(?=.*[_.()$&@]).*$")){ hasSpecialSymbol = true; card4.setCardBackgroundColor(Color.parseColor(getString(R.color.colorAccent))); }else{ hasSpecialSymbol = false; card4.setCardBackgroundColor(Color.parseColor(getString(R.color.colorGrey))); } // } private void inputChanged(){ etPassword.addTextChangedListener(new TextWatcher() { @Override public void beforeTextChanged(CharSequence s, int start, int count, int after) { } @SuppressLint("ResourceType") @Override public void onTextChanged(CharSequence s, int start, int before, int count) { passwordValidate(); if(is8char && hasnum && hasSpecialSymbol && hasUpper) { cardButtonSignUp.setCardBackgroundColor(Color.parseColor(getString(R.color.colorPrimary))); } } @Override public void afterTextChanged(Editable s) { } // }); // } }
Final Output