In today’s digital world, API’s (Application Programming Interfaces) are very mush helpful for developers out their, That Enables them to add new functionalities & a data sources into the application developer is building. Most of the time Free-to-use API’s provides fresher developer to practice their skill and build a project using the Free API’s. Here in this articles let’s explore 7 Free to use API that can be used in various project whether you are building websites. Mobile Apps or other Applications.
7 Free-to-Use APIs for Developers
1. Coinbase API
This API Service can be used to easily integrate bitcoin, bitcoin cash, litecoin & ethereum real-time cryptocurrency in your application.
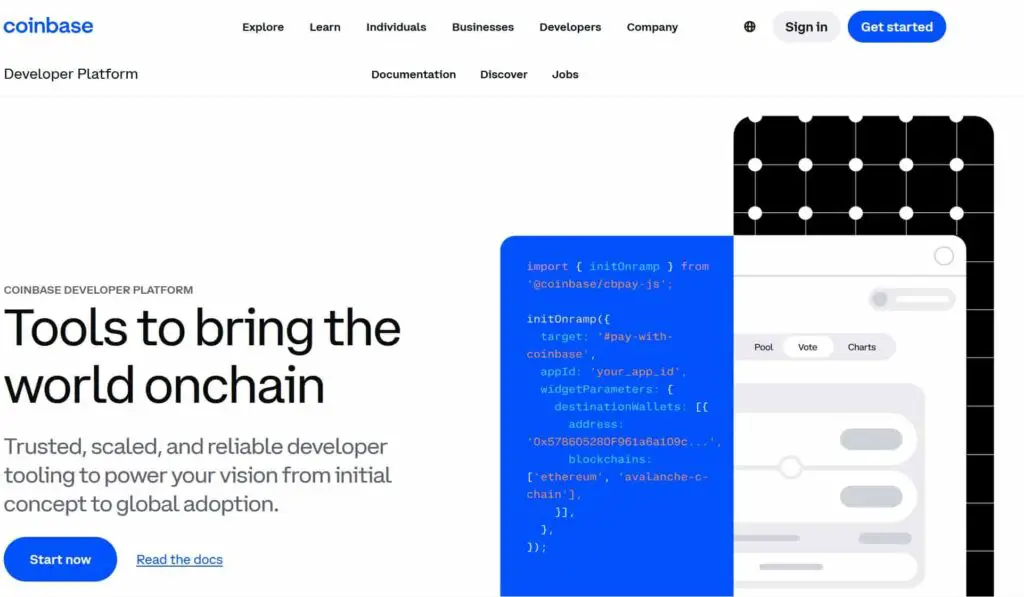
2. Hotels
This API Helps to Query rooms, price & other info from many hotels around the world, This API can be used by developers who are working on building traveling apps.
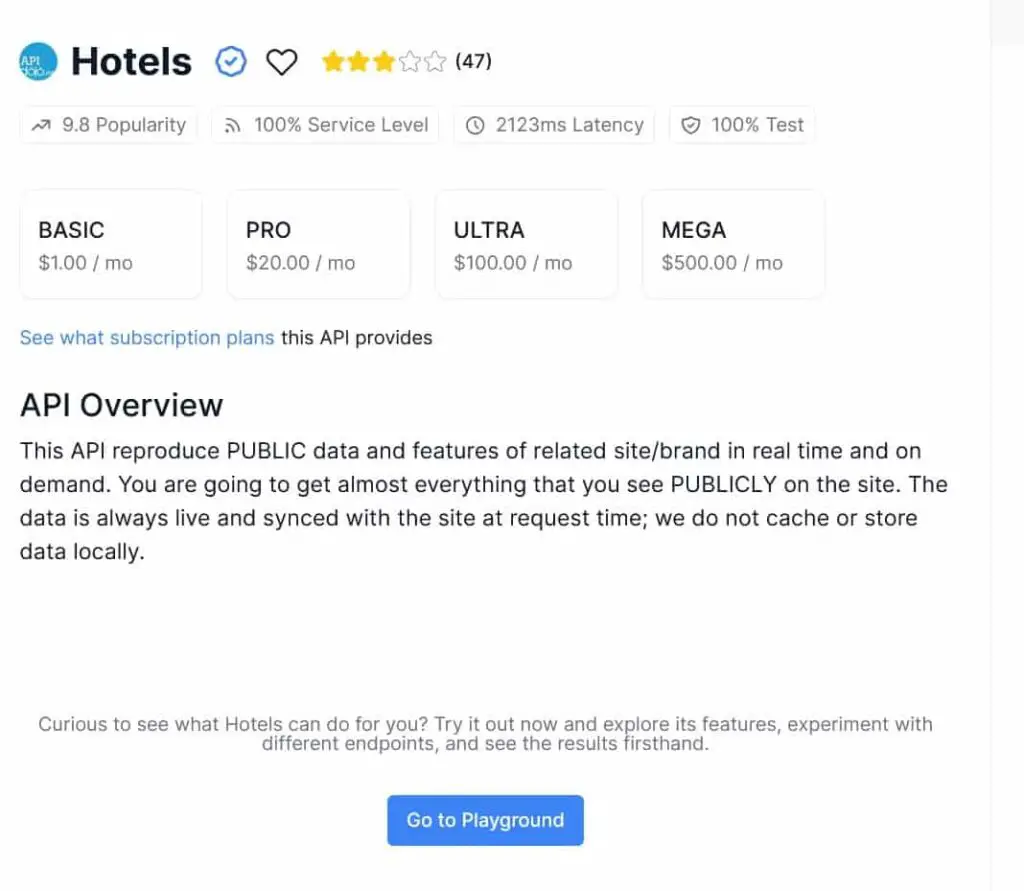
3. Data USA
Allows developer to explore the entire database to get public US data (Eg: Population Data).
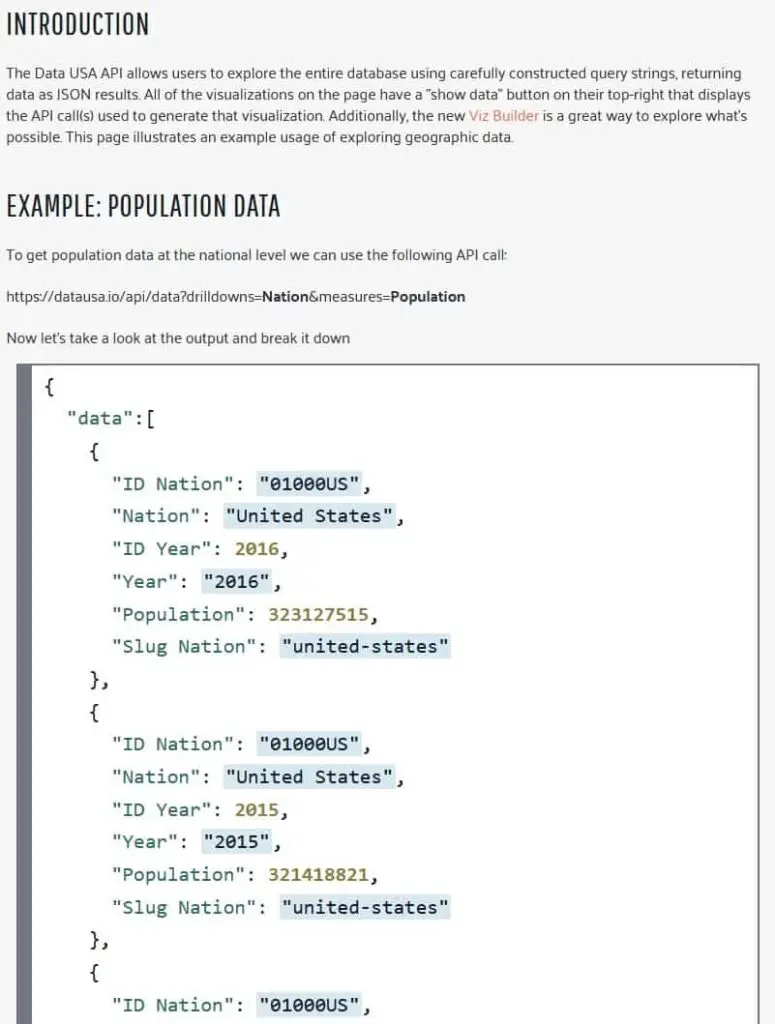
4. IPinfo
This app is useful when you are working on IP address, This gets you information about specific IP address, such as geological info, company name & carrier name etc.
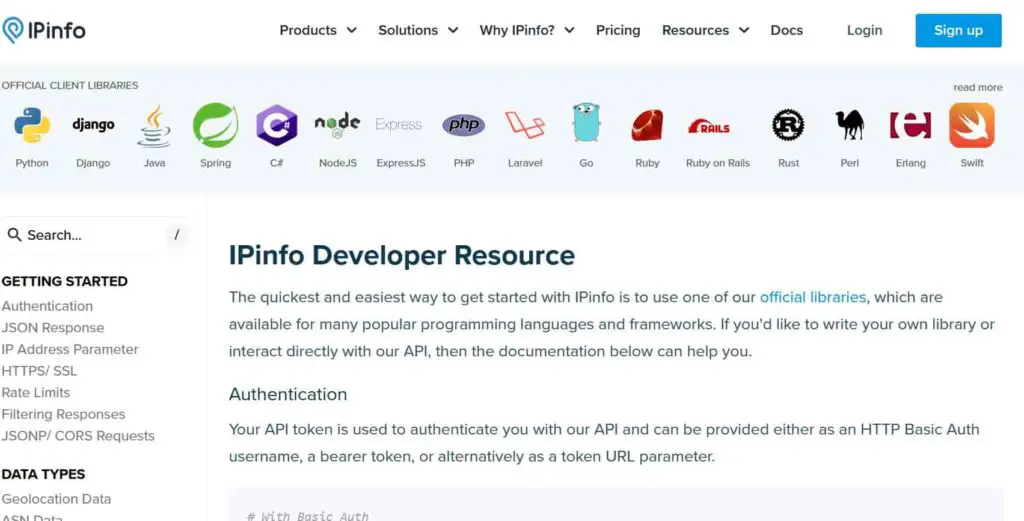
5. Weather
Using this API makes it easy to access accurate weather forecasts & get historical weather data.
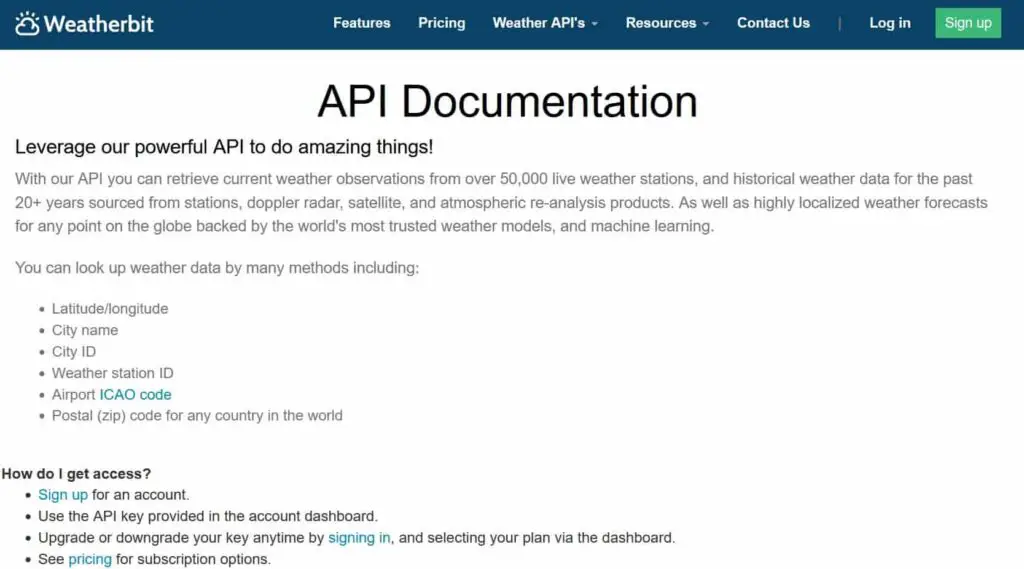
6. Google Translate
Allows you to dynamically & easily translate an arbitrary string into any supported langauge.
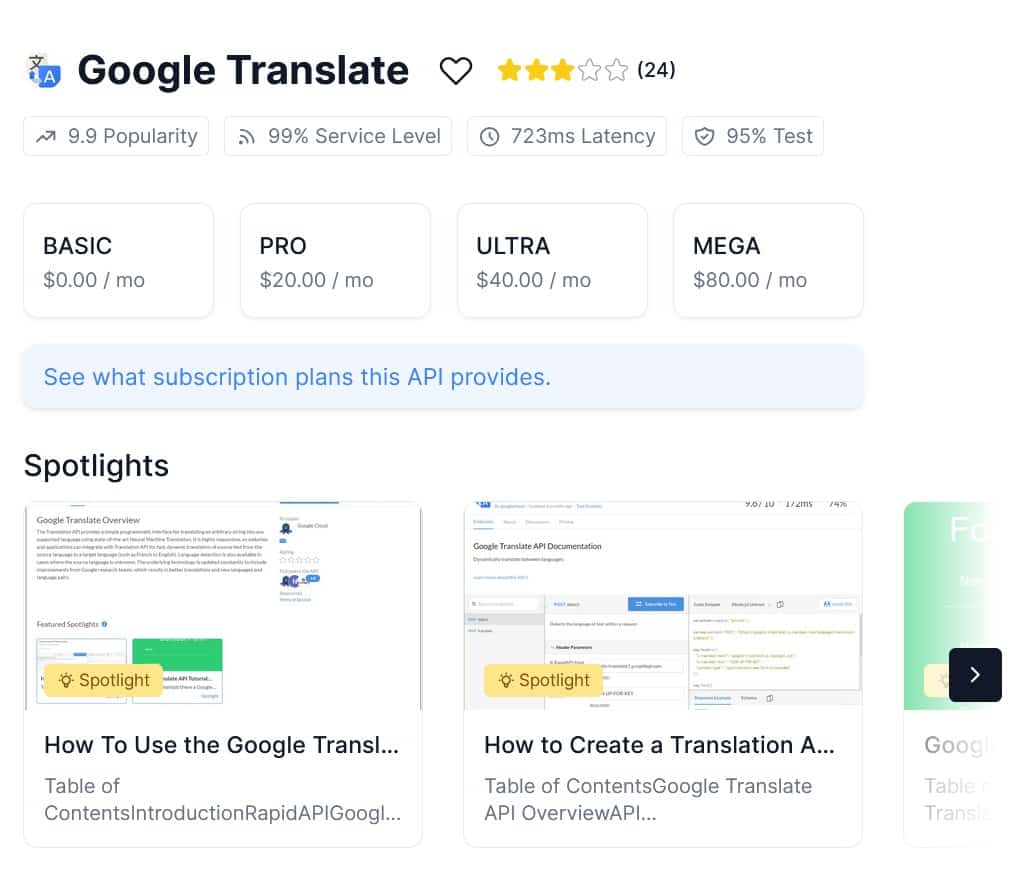
7. Alpha Vantage
Alpha Vantage is a simple & effective API using which we can access financial data like stock, ETF, forex, Technical indicators & cryptocurrency data. This API offer real-time and also previous data. Alpha Vantage is the valuable free to use api for developer who are building application related to finance.
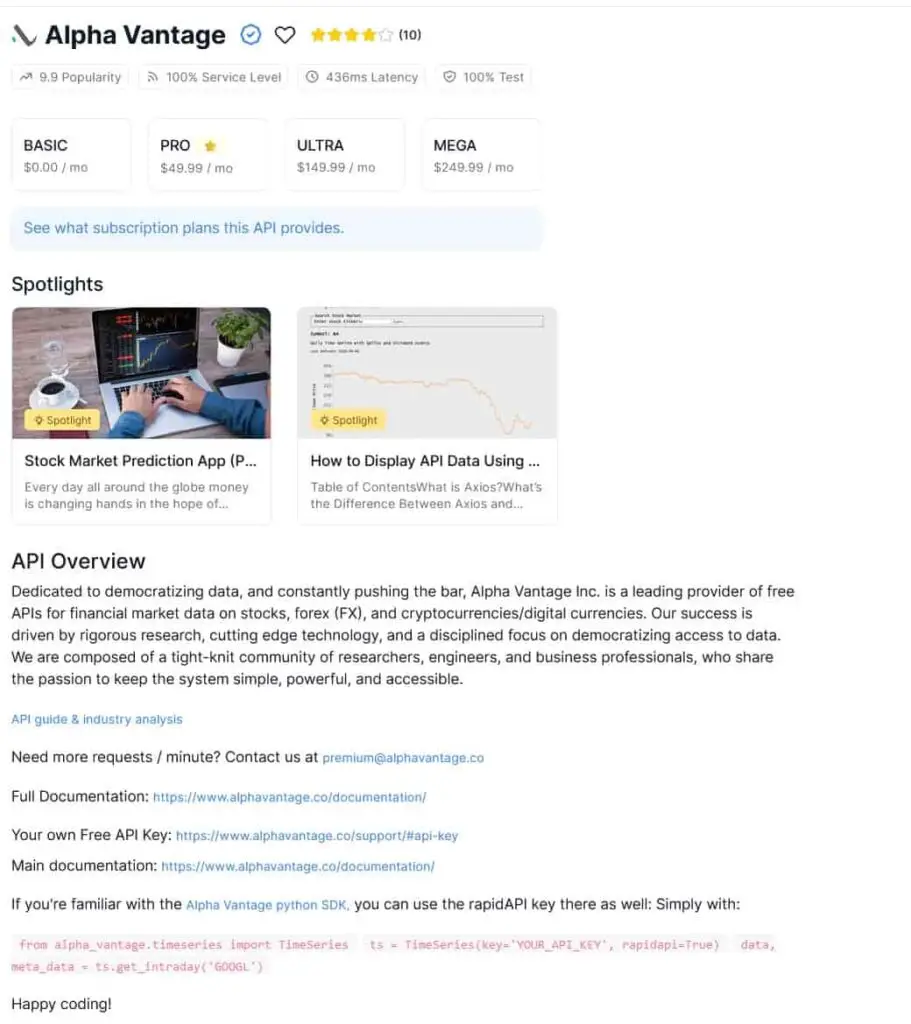