Hi Guy’s Welcome to Proto Coders Point. In this article let’s learn about Event Loop in JavaScript with code example.
What is Event Loop?
As you know that JavaScript works on a single threads, It can execute only one task at a time, But when building application using JS at backend, We can execute multiple tasks at a same time, like instance, Listening for events, and timeout etc.
To handle multiple event or calculation JavaScript make use of event loop. It is a concurrency model that has four major component. i.e. Call Stack, Web API’s, Task Queue and Micro Task Queue.
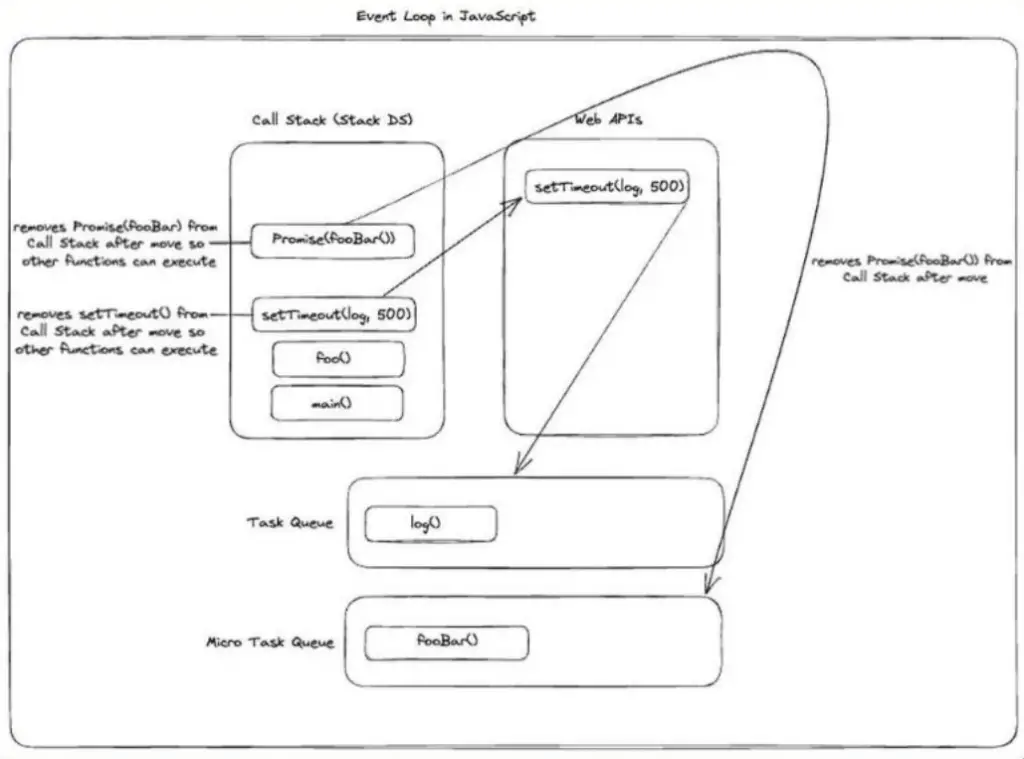
Component’s of JavaScript Event Loops
1. Call Stack: A Stack Data Structure (LIFO) Last In First Out Stack Model, that is used for keeping track of current execution function calls.
2. Web API’s: When any API event like setTimeout or DOM manipulation function gets into the call Stack, They are moved to separate bucket known as Web API’s. Once the API execution is completed is is poped from the Call Stack so that Call Stack can execute next.
3. Task Queue: When the setTimeout() delay finishes, The Web API moves the timeout to the Task Queue. Then the Task Queue will wait for the Call Stack to get empty. Once it gets empty, It will remove the first task from the queue & moves it on the Call Stack to execute that code.
4. Micro Task Queue: This is Another Queueing system, Micro Task Queue has Higher Priority than Task Queue but it holds promises instead of Web API’s. It Follow the same principle of pushing task into Call Stack when Call Stack is empty.
Event loop in javascript example or In NodeJS
Here is a example on event loop in JavaScript:
Code:
// Define a function that simulates an asynchronous task function simulateAsyncTask(taskName, duration) { console.log(`${taskName} started`); setTimeout(() => { console.log(`${taskName} completed after ${duration} milliseconds`); }, duration); } // Simulate two asynchronous tasks simulateAsyncTask("Task 1", 2000); simulateAsyncTask("Task 2", 1000); console.log("Main program continues executing...");
Event Loop Code Explanation:
We have created a function by name simulateAsyncTask
which accepts two parameters: taskName
(a string representing the name of the task) and duration
(the duration of the task in milliseconds).
Then simulateAsyncTask
, we firstly print/log that the task has started, then use setTimeout
to simulate an asynchronous operation and then pass the timeout duration
to complete it. Once the timeout elapses, we print/ log that the task has completed.
We simulate two asynchronous tasks: “Task 1” with a duration of 2000 milliseconds i.e. 2 seconds & “Task 2” with a duration of 1000 milliseconds i.e. 1 second.
After starting the asynchronous tasks, we have simply made use of console.log that print msg on the screen “Main program continues executing…”.
When you run this code, you’ll notice that “Main program continues executing…” is logged immediately, before the asynchronous tasks complete. This demonstrates the non-blocking nature of asynchronous JavaScript code and the event loop in action. The event loop manages the execution of asynchronous tasks, allowing the main program to continue executing while waiting for tasks to complete.