Cupertino Timer Picker is IOS style timer picker in flutter, that allows user to search a duration of time or a time interval. In this Flutter Article let’s learn how to implement CupertinoTimerPicker in flutter with complete source code.
If you’re looking for a Cupertino-style timer picker i.e ios style time picker, then flutter provide a Timer Picker widget in Material Design class named CupertinoTimerPicker Here’s a basic example of how you can create a Cupertino-style timer picker:
We can make use of showCupertinoModalPopup widget to show a popup from the bottom of the screen.
Video Tutorial
Creating the Timer Picker Widget:
We have a function _showTimerPicker
that open a popup at the bottom of the screen and displays the Timer Picker when a user press on a button to select a time duration user can select time in hours, minutes and seconds, To show type of mode that i.e. hms we can make use of mode property from CupertinoTimerPicker widget.
snippet – CupertinoTimerPacker Widget constructor
CupertinoTimerPicker( { Key? key, CupertinoTimerPickerMode mode = CupertinoTimerPickerMode.hms, Duration initialTimerDuration = Duration.zero, int minuteInterval = 1, int secondInterval = 1, AlignmentGeometry alignment = Alignment.center, Color? backgroundColor, double itemExtent = _kItemExtent, required ValueChanged<Duration> onTimerDurationChanged } )
Handling Timer Selection and displaying selected time in Text Widget
The selected duration is stored in the selectedDuration
variable initially it will be 0 for hours,minutes and seconds duration, When the user selects a new duration from Cupertino Timer Picker, the onTimerDurationChanged
callback is triggered which has callback duration value selected using which we can update the selectedDuration
using setState
to reflect the user’s selection.
Source Code – CupertinoTimerPicker Flutter
Output
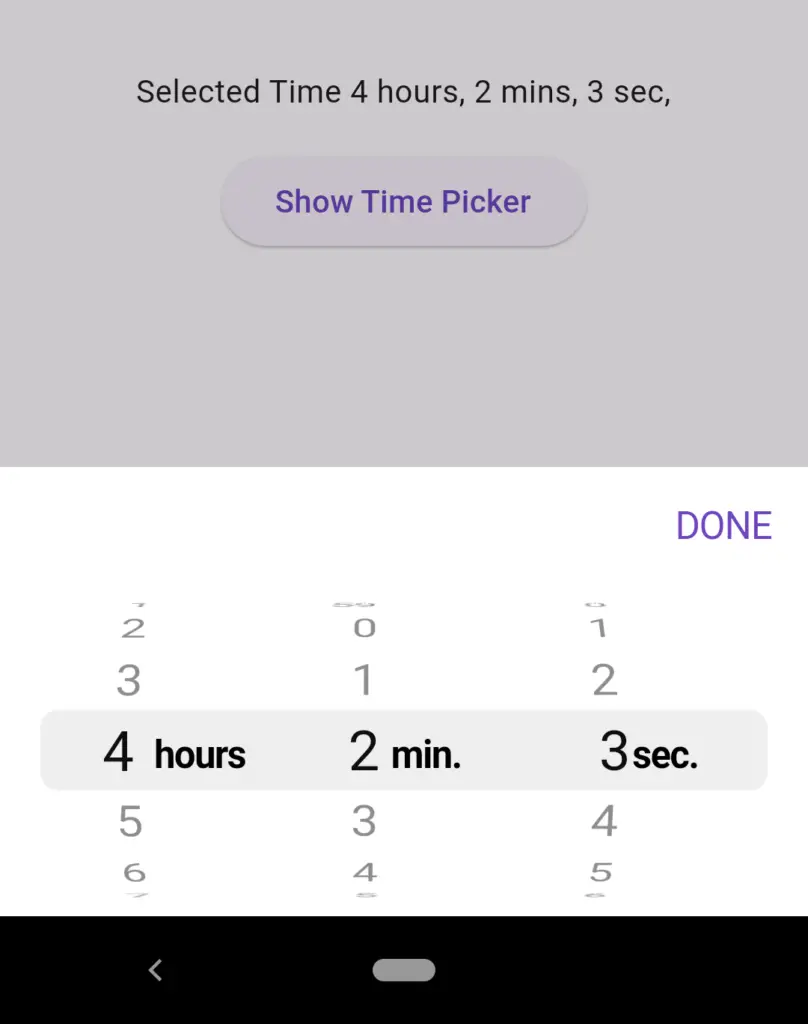
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', debugShowCheckedModeBanner: false, theme: ThemeData( colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple), useMaterial3: true, ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({super.key}); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { Duration selectedDuration = Duration(hours: 0,minutes: 0,seconds: 0); void _showTimerPicker(BuildContext context){ showCupertinoModalPopup( context: context, builder: (BuildContext context){ return Container( height: 200, color: CupertinoColors.white, child: Column( children: [ Row( mainAxisAlignment: MainAxisAlignment.end, children: [CupertinoButton(child: Text("DONE"), onPressed: (){ Navigator.of(context).pop(); })], ), Expanded( child: CupertinoTimerPicker( mode: CupertinoTimerPickerMode.hms, initialTimerDuration: selectedDuration, onTimerDurationChanged: (Duration duration){ setState(() { selectedDuration = duration; }); } ), ) ], ), ); } ); } @override Widget build(BuildContext context) { return Scaffold( body: Center(child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text('Selected Time ${selectedDuration.inHours} hours, ${selectedDuration.inMinutes % 60} mins, ${selectedDuration.inSeconds % 60} sec,'), SizedBox(height: 15,), ElevatedButton(onPressed: (){_showTimerPicker(context);}, child: Text("Show Time Picker")) ], ),), ); } }
Conclusion: This article introduced you to the Flutter Cupertino Timer Picker and showed you how to implement Time Picker in your flutter app in IOS style UI. You can further customize it to match the style of your app and use it to collect time-related input from your users.
References and Resources:
- Official Flutter documentation: CupertinoTimerPicker
- Flutter’s official website: https://flutter.dev/
Related Article
Bottom Popup Cupertino action sheet flutter
easiest way to implement image picker in flutter