Hi Guys, welcome to Proto Coders Point , In this Flutter Tutorial we will discuss and Implement Flutter Toast Message using Bot Toast Library.
Learn more about this library from Official Site
What is Toast Message?
Some TImes Toast Messages are also called as Toast Notification.
It’s a small message that pop up at the bottom of the device screen and immediately disappears on it’s own after a delay of few seconds,
This are mostly used to show a feedback on the operation that is preformed by the user.
I have Already wrote a post on another flutter toast message library which is very simple and old style to show Toast message example.
So, Let’s Begin Implementing Flutter Bot Toast Message into your Flutter project.
Flutter Bot Toast Library – message, Notification, Attached Widget pop up
Step 1 : offCourse you need new or existing flutter project
I am using Android-studio to work with Flutter Development, Check out How to add Flutter Plugin in android Studio
Create a new Flutter project
File > New > New Flutter Project
Step 2 : Add bot toast dependencies in pubspec.yaml file
Then, once you Flutter project is been created open pubspec.yaml file and you need to add Bot_Toast dependencies
dependencies:
bot_toast: ^2.2.1 //add this line
// version may update check official site for more
then click on Package Get
Step 3 : Import bot toast package
then, when you have successfully added the library into your flutter project, now you will be able to use this bot toast message package where-ever required, just you need to import the bot_toast.dart file.
import 'package:bot_toast/bot_toast.dart';
add this import statement on top of main.dart in your project.
Step 4 : Initialization of Bot Toast
Widget build(BuildContext context) {
return BotToastInit( // wrap MaterialApp with BotToastInit
child: MaterialApp(
title: 'Flutter Demo',
debugShowCheckedModeBanner: false,
navigatorObservers: [BotToastNavigatorObserver()], //Register route observer
navigatorKey: navigatorkey,
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
),
);
Then, to make user of the library you need to :
- Step 1 : Wrap MaterialApp with BotToastInit
- Step 2 : Register route Observer
As shown in above Snippet Code.
Step 5 : Different ways to show Toast message or Toast Notification
1. Simple Text Message
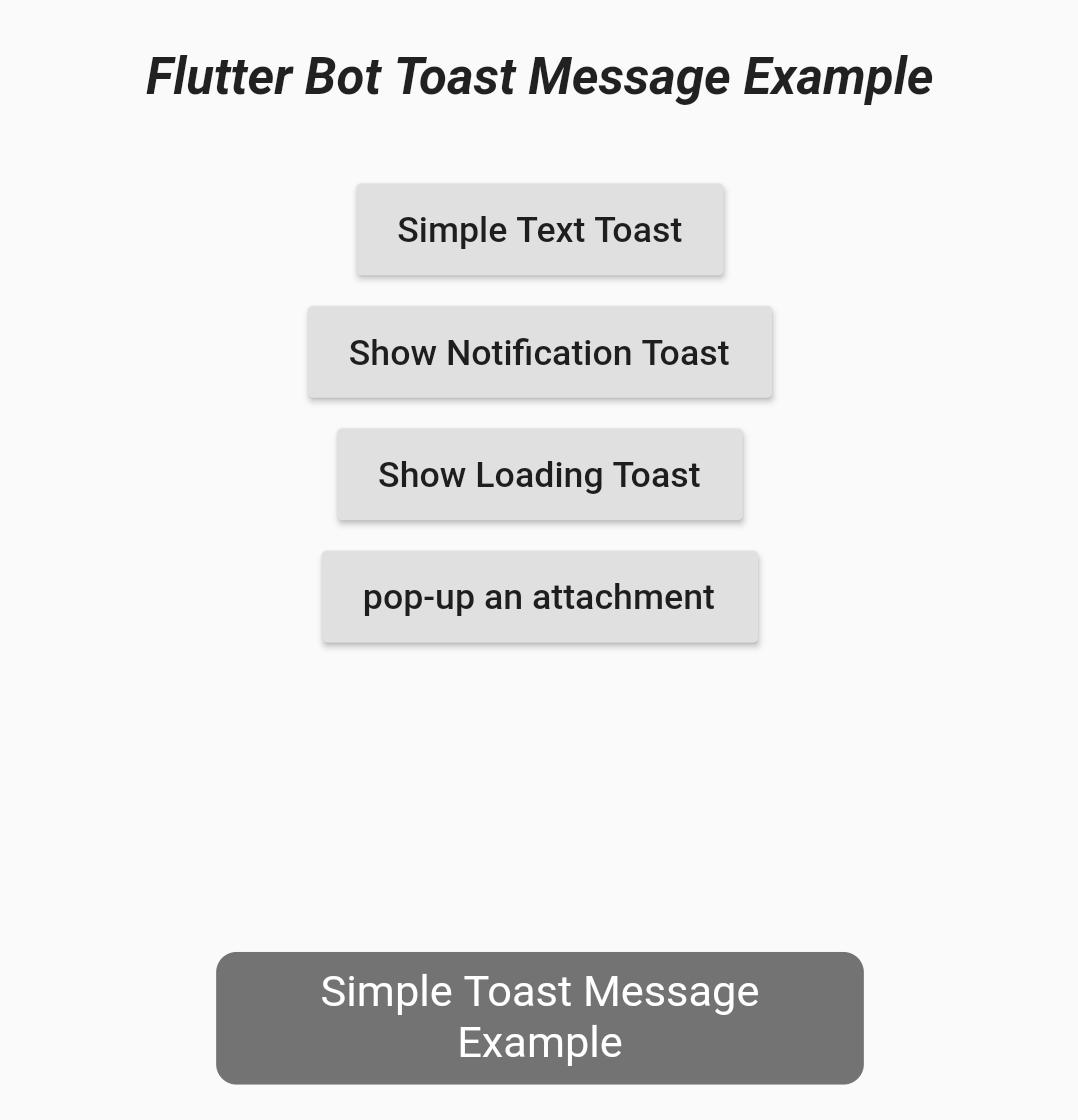
RaisedButton(
child: Text("Simple Text Toast"),
onPressed: () {
BotToast.showText(text: "Simple Toast Message Example");
},
),
2. Show a Toast Notification on top of the Screen
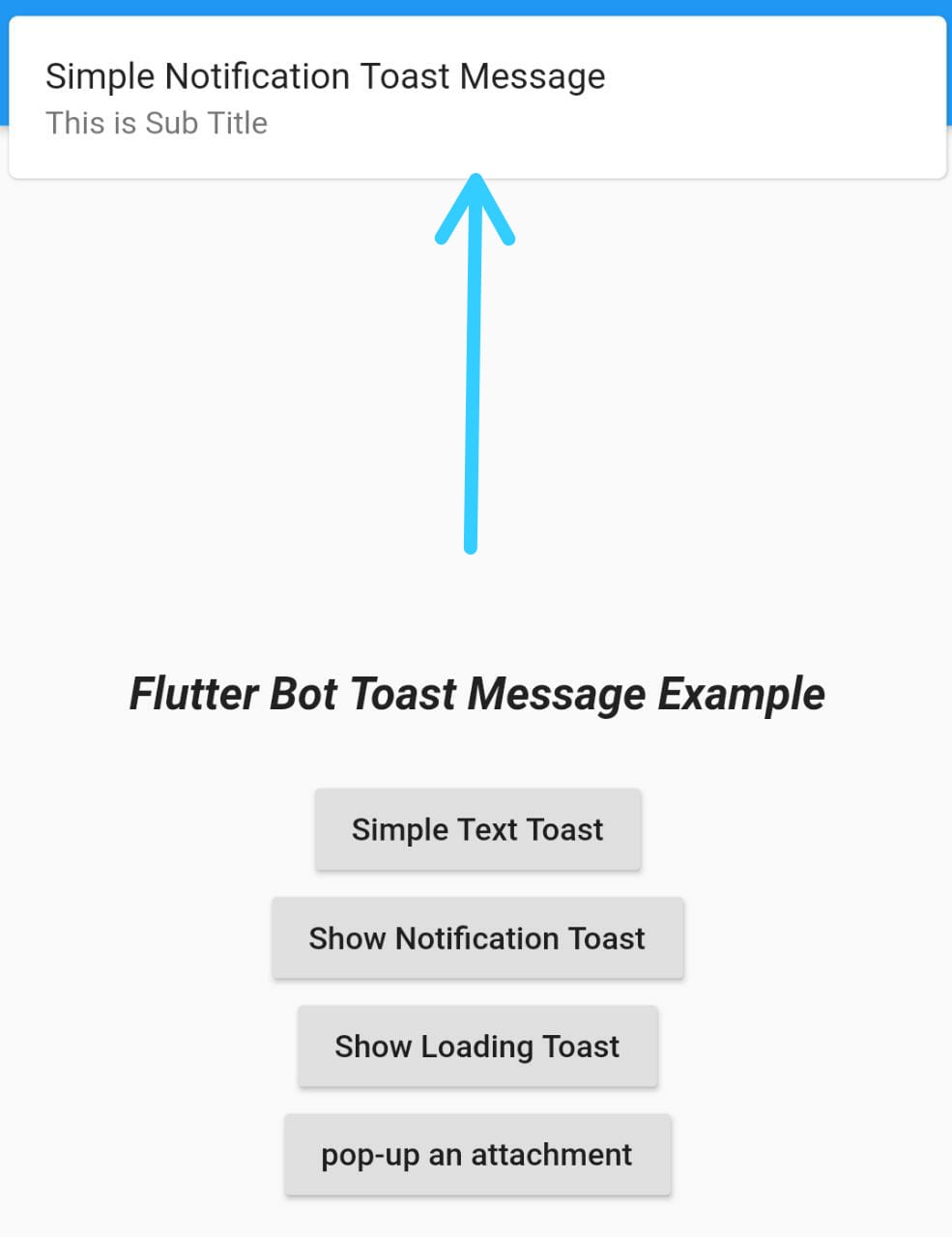
RaisedButton(
child: Text("Show Notification Toast"),
onPressed: () {
BotToast.showSimpleNotification(
title: "Simple Notification Toast Message",
subTitle: "This is Sub Title",
hideCloseButton: true,
);
},
),
This Snippet Code will show a Notification when user click on the button,
There are various property that can we used eg :
title : show text on the notification
subTitle : shows description
hideCloseButton : their is a close button on the Toast Notification using which user can close immedietly by clicking on close button, we can hide that button by setting it to true.
3. Show Loading Toast
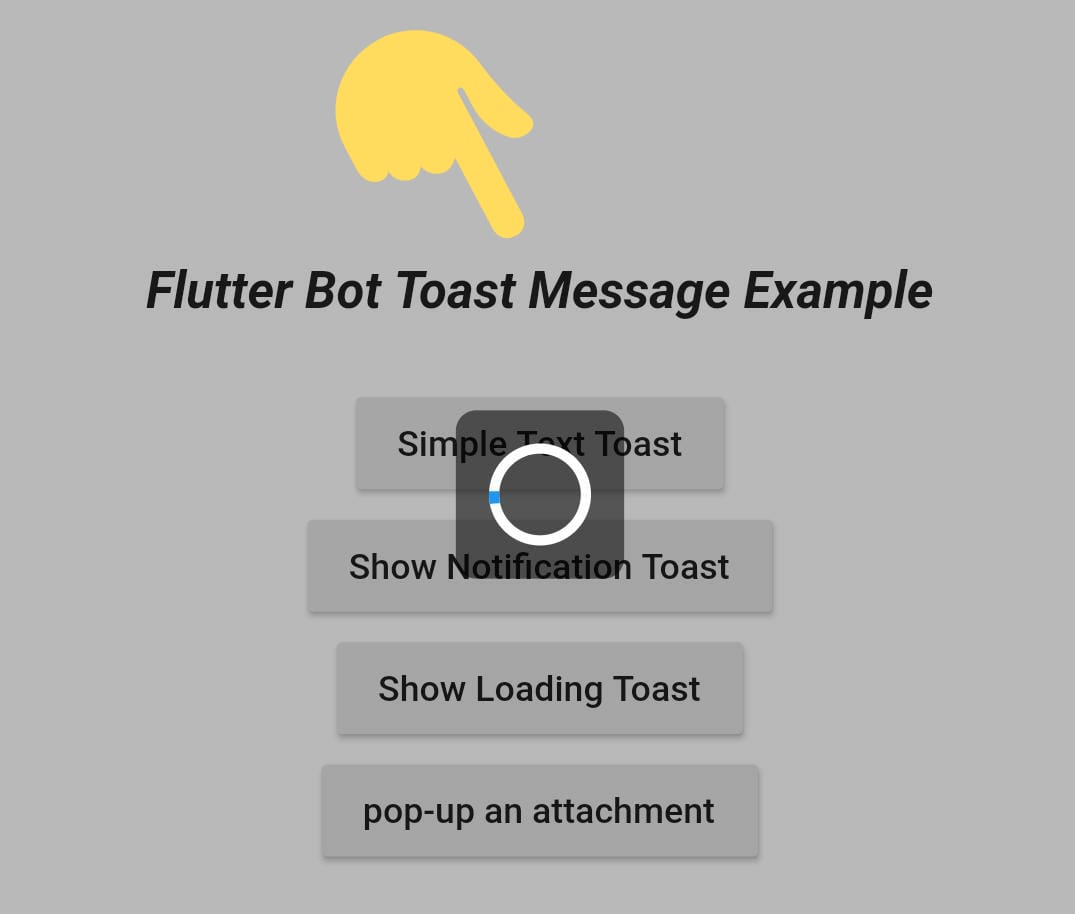
RaisedButton(
child: Text("Show Loading Toast"),
onPressed: () {
BotToast.showLoading(
clickClose: true,
); //when loading toast is clicked it closes
},
),
4. Show Attached Widget
you can use Attached Widget to show any kind of Widget in the Toast Form.
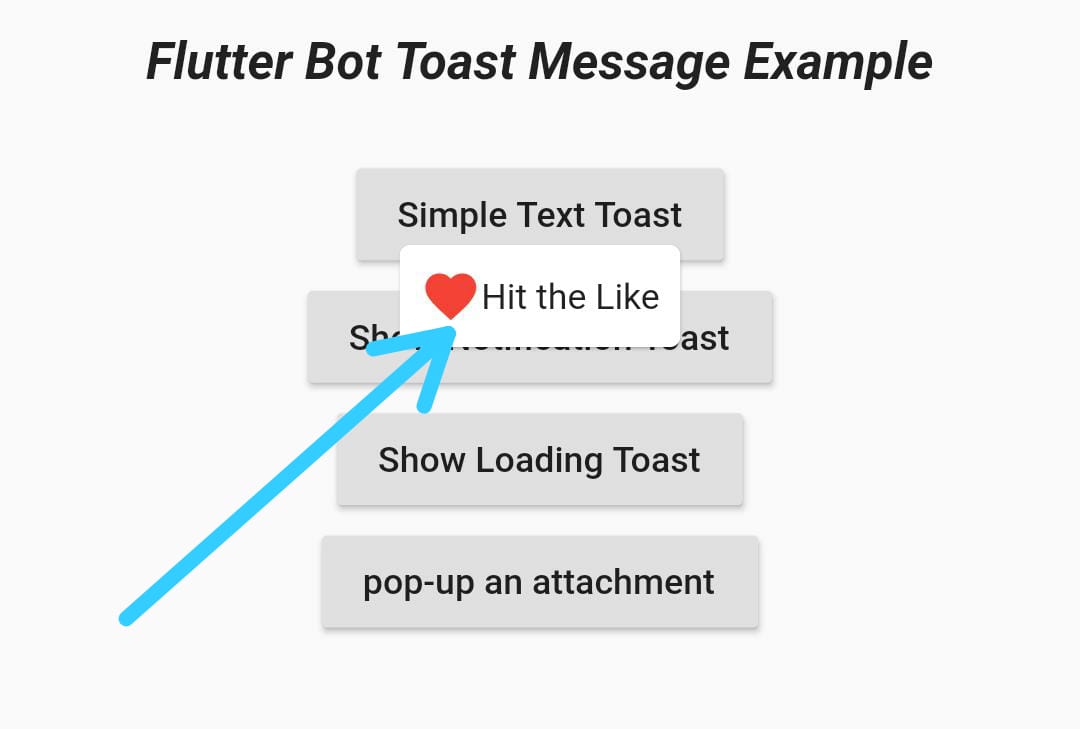
RaisedButton(
child: Text("pop-up an attachment"),
onPressed: () {
BotToast.showAttachedWidget(
allowClick: true,
attachedBuilder: (_) => Center(
child: Card(
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Row(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Icon(
Icons.favorite,
color: Colors.red,
),
Text("Hit the Like"),
],
),
),
),
),
target: Offset(520, 520),
duration: Duration(
seconds: 3)); //when loading toast is clicked it closes
},
),
In the Above Snippet code i have attached Card with child as Row where Row contain 2 children Icon and Text.
And also we need to set the duration to show the Attached Toast widgets.
Complete Code of this Flutter Tutorial on Bot Toast Message
When you know the basic of this Flutter Bot Toast message libray just Copy paste the below lines of code in main.dart file
main.dart
import 'package:flutter/material.dart';
import 'package:bot_toast/bot_toast.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
// This widget is the root of your application.
GlobalKey<NavigatorState> navigatorkey = GlobalKey<NavigatorState>();
@override
Widget build(BuildContext context) {
return BotToastInit(
child: MaterialApp(
title: 'Flutter Demo',
debugShowCheckedModeBanner: false,
navigatorObservers: [BotToastNavigatorObserver()],
navigatorKey: navigatorkey,
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(),
),
);
}
}
class MyHomePage extends StatefulWidget {
@override
_MyHomePageState createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Flutter Bot Toast"),
),
body: Center(
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: <Widget>[
Text(
"Flutter Bot Toast Message Example",
style: TextStyle(
fontSize: 20.0,
fontWeight: FontWeight.w800,
fontStyle: FontStyle.italic),
),
SizedBox(
height: 25,
),
RaisedButton(
child: Text("Simple Text Toast"),
onPressed: () {
BotToast.showText(text: "Simple Toast Message Example");
},
),
RaisedButton(
child: Text("Show Notification Toast"),
onPressed: () {
BotToast.showSimpleNotification(
title: "Simple Notification Toast Message",
subTitle: "This is Sub Title",
hideCloseButton: true,
);
},
),
RaisedButton(
child: Text("Show Loading Toast"),
onPressed: () {
BotToast.showLoading(
clickClose: true,
); //when loading toast is clicked it closes
},
),
RaisedButton(
child: Text("pop-up an attachment"),
onPressed: () {
BotToast.showAttachedWidget(
allowClick: true,
attachedBuilder: (_) => Center(
child: Card(
child: Padding(
padding: const EdgeInsets.all(8.0),
child: Row(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisSize: MainAxisSize.min,
children: <Widget>[
Icon(
Icons.favorite,
color: Colors.red,
),
Text("Hit the Like"),
],
),
),
),
),
target: Offset(520, 520),
duration: Duration(
seconds: 3)); //when loading toast is clicked it closes
},
),
],
),
),
);
}
}
And here you go your app is ready to show toast messages on both iOS and Android