Hi Guy’s Welcome to Proto Coders Point, In this Flutter tutorial let’s learn how to get tap position i.e. X & Y axis coordinates when user taps on mobile screen.
This article is a quick guide on how to get user tap position XY coordinates in flutter.
Video Tutorial
How to get touch position of user
Flutter Tap Position X & Y Coordinates
In flutter, It’s very easily to get screen tap position by using GestureDetector Widget with onTapUp or OnTapDown event function.
Example:
GestureDetector( onTapDown: (position){ final tapPosition = position.globalPosition; final x = tapPosition.dx; final y = tapPosition.dy; // do something with x & y }, child: Scaffold(/* ..Whole App UI Screen.. */) )
Flutter GestureDetector get position XY axis coordinates
Example:
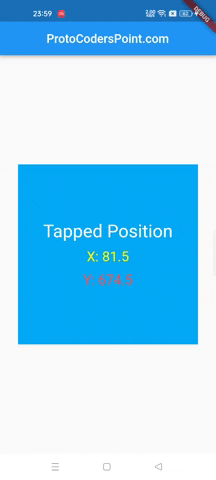
Source Code for Reference:
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { Offset? _tapPosition; @override Widget build(BuildContext context) { return GestureDetector( onTapDown: (position){ setState(() { _tapPosition = position.globalPosition; }); }, child: Scaffold( appBar: AppBar(title: Text('ProtoCodersPoint.com'),centerTitle: true,), body: Center( child: Container( color: Colors.lightBlue, width: 300, height: 300, child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text('Tapped Position',style: TextStyle(color: Colors.white,fontSize: 30),), SizedBox(height: 12,), Text('X: ${_tapPosition?.dx.toString() ?? 'Tap SomeWhere'}',style: TextStyle(color: Colors.white,fontSize: 23),), SizedBox(height: 12,), Text('Y: ${_tapPosition?.dy.toString()?? 'Tap SomeWhere'}',style: TextStyle(color: Colors.white,fontSize: 23),) ], ), ), ) ), ); } }
Recommended Flutter Article
Flutter Show Popup menu at long press position