Hi Guys, Welcome to Proto Coders Point.
Sometime, you not only want to navigate user to next screen page, but also want to pass data from screen 1 to screen 2.
For Example: A Screen 1 in your flutter app has a TextField & user enter the data, same data you want on screen 2 for further action.
In this flutter tutorial example, we will learn how to pass data between screens – In other words flutter send data to another page.
Flutter pass data between screens – Complete Video tutorial
Here we will have 2 Screens.
1. main.dart(Screen 1): Has TextField ( Name, Email, Phone ) where user can enter data & a button when pressed sends data from screen 1 to screen 2 using Navigator.push() to screen 2 with data passed through a constructor.
2. WelcomePage.dart(Screen 2): Will have a constructor that received data from screen 1 and then display the data in Text Widget in Screen 2.
So let’s begin with Code
1. Create a dart file – WelcomePage.dart
In Welcome page, we will just show the data received from main.dart page in a Text Widget.
As i said above, WelcomePage.dart will have a Constructor that accepts some Required data String parameter such as Name, Email, Phone.
#constructor Snippet Code String name,email,phone; WelcomePage({Key? key,required this.name,required this.email,required this.phone}) : super(key: key);
Then use the String data to print in Text Widget.
Text('Name: $name'),
Note: If you are using Stateful Widget, then you cannot directly use the data, for that you need to use widget.data as below.
Text('Name: ${widget.name}'),
WelcomePage.dart Complete Code
import 'package:flutter/material.dart'; class WelcomePage extends StatelessWidget { String name,email,phone; WelcomePage({Key? key,required this.name,required this.email,required this.phone}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Center( child: Column( crossAxisAlignment: CrossAxisAlignment.start, children: [ Text('Name: $name'), Text('Email: $email'), Text('Phone: $phone'), ], ), ), ), ); } }
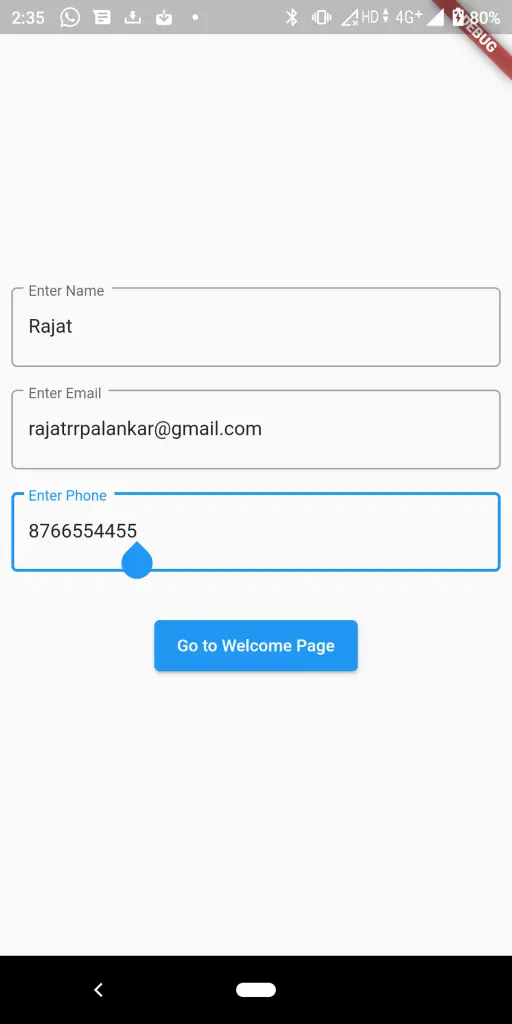
2. main.dart – Where user will Enter The Data in TextField.
In main.dart, we will have 3 TextField where user can enter his Name, Email & Phone No and a button when pressed will send the entered data to Page 2(WelcomePage.dart) using Navigator.push() & pass the data through WelcomePage Constructor.
In each TextField we have attached TextEditingController that will hold the data entered by the user in textField.
Navigator to pass data to screen 2 ( Welcomepage)
Navigator.of(context).push(MaterialPageRoute( builder: (context) => WelcomePage( name: _name.text, email: _email.text, phone: _phone.text) ) );
main.dart complete code
import 'package:flutter/material.dart'; import 'package:passing_data/ToDoDetail.dart'; import 'package:passing_data/Todo.dart'; import 'package:passing_data/WelcomePage.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: Form(), ); } } class Form extends StatefulWidget { const Form({Key? key}) : super(key: key); @override _FormState createState() => _FormState(); } class _FormState extends State<Form> { TextEditingController _name = TextEditingController(); TextEditingController _email = TextEditingController(); TextEditingController _phone = TextEditingController(); @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Padding( padding: const EdgeInsets.all(8.0), child: TextField( controller: _name, decoration: InputDecoration( border: OutlineInputBorder(), labelText: 'Enter your Name' ), ), ), Padding( padding: const EdgeInsets.all(8.0), child: TextField( controller: _email, decoration: InputDecoration( border: OutlineInputBorder(), labelText: 'Enter your Email' ), ), ), Padding( padding: const EdgeInsets.all(8.0), child: TextField( controller: _phone, decoration: InputDecoration( border: OutlineInputBorder(), labelText: 'Enter your Phone No' ), ), ), ElevatedButton(onPressed: (){ Navigator.of(context).push(MaterialPageRoute(builder: (context)=>WelcomePage(name: _name.text, email: _email.text, phone: _phone.text))); }, child: Text('Go Next Page')) ], ), ), ); } }
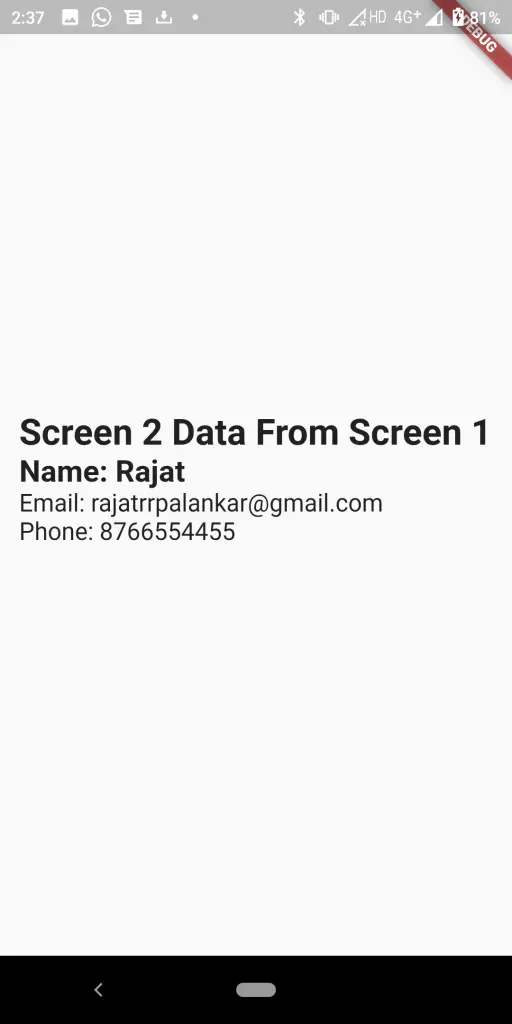