As you all know the Flutter is a powerful framework using which we can build cross-platform applications for Mobile/Web. When a popular frameworks comes to the Technical market the ability to integrate third-party packages or dependencies becomes easily & al to enhance functionality.
Hi Guy’s Welcome to Proto Coders Point. In this Article, Let’s us talk and make you walk through the process how easily it is adding dependencies in Flutter, managing versions, and updating them properly.
What are Dependencies in Flutter?
So Dependencies in Flutter are basically a pre-defined code of code or an external packages also called as libraries that a developer can freely use and add functionalities to an up coming app. These packages are freely available on pub.dev website, which is the official package manager for Dart and Flutter.
Just by download and using dependencies, you can easily make use of freely features like:
- Networking (e.g., HTTP requests)
- State management (e.g., Provider, Riverpod, Bloc)
- UI components (e.g., animations, charts)
- Database and storage (e.g., SQLite, Firebase)
This is just an example, In pub.dev there are lots of package that published by just like us developer.
Steps to Add a Dependency in Flutter
1. Open pubspec.yaml
In root directory of your flutter project there should be a file by name: pubspec.yaml
that is metadata or information of flutter app it also contain dependencies that are used by the project. You need to add your dependencies here.
2. Search for a Package
Visit pub.dev & search for the package you are interested in. For example, let say I want to make a HTTP call for that I need http dependencies for making API requests, in pub.dev search for http
.
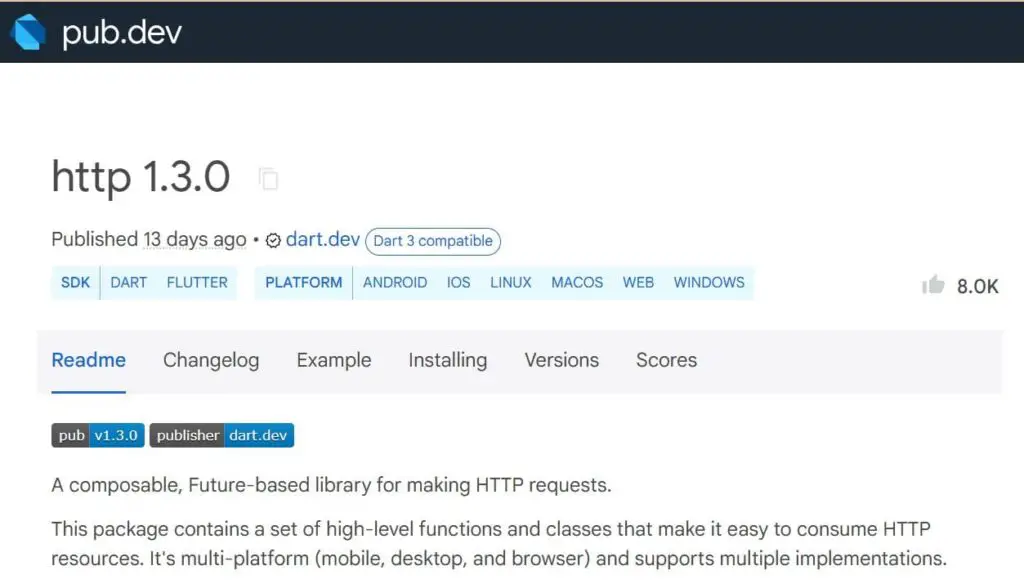
Here go to Installing tab.
3. Add the Dependency
Once you find the package in our case it http, copy the dependency line (http: ^0.13.6 ) from the installation section & paste it in your flutter project under the dependencies:
section in pubspec.yaml
just like shown below.
Example:
dependencies: flutter: sdk: flutter http: ^0.13.6
4. Run flutter pub get
Now once you added the required dependency you need to run a command in the terminal to fetch/download the package make sure you are connected to internet:
flutter pub get
by running above command it will downloads all the listed dependencies in our case it only one i.e. http and then makes it available for use in your project.
5. Import & use the Package
Then Now, once your required dependencies is successfully downloaded, you can use it by just importing the package in your Dart file where required.
Example:
import 'package:http/http.dart' as http; void fetchData() async { var response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/posts')); print(response.body); }
Managing Dependency Versions
In some situation you might need a specify dependency versions:
- Specific Version:
http: 0.13.6
This installs the exact version. - Caret Syntax (
^
):http: ^0.13.6
This installs the latest compatible version within0.x.y
. - Latest Version (
any
):http: any
This installs the latest available version. - Git Dependencies:
dependencies: my_package: git: url: https://github.com/username/repository.git
This fetches a package from a Git repository. - Path Dependencies:
dependencies: my_local_package: path: ../my_local_package
This is useful for local packages.
How to update the flutter Dependencies
It’s very easily to update the flutter dependencies, just run upgrade command as below:
flutter pub upgrade
To check outdated dependencies, run command outdated:
flutter pub outdated
To update a specific dependency, just check the dependencies version from pub.dev and then modify pubspec.yaml
then run:
flutter pub get
Removing Dependencies
If you no longer need a package and want to completely delete it from the flutter project, follow below steps:
- Remove the dependency from
pubspec.yaml
. - Run:
flutter pub get
This removes the package from the project.