Hi Guys, Welcome to Proto Coders Point. This Article is on different ways to create a full width button in flutter, Basically How to make button acquire available screen width and make button responsive in flutter.
Video Tutorial
1. Elevated Button Full width – using Container / SizedBox
For any button to acquire complete available space (width), you need to wrap button widget i.e. (ElevatedButton, TextButton, OutlineButton) with Container widget / SizedBox the use width property with double.infinity so that it child will acquire the complete width of screen & make the button responsive in flutter.
Code Example:-
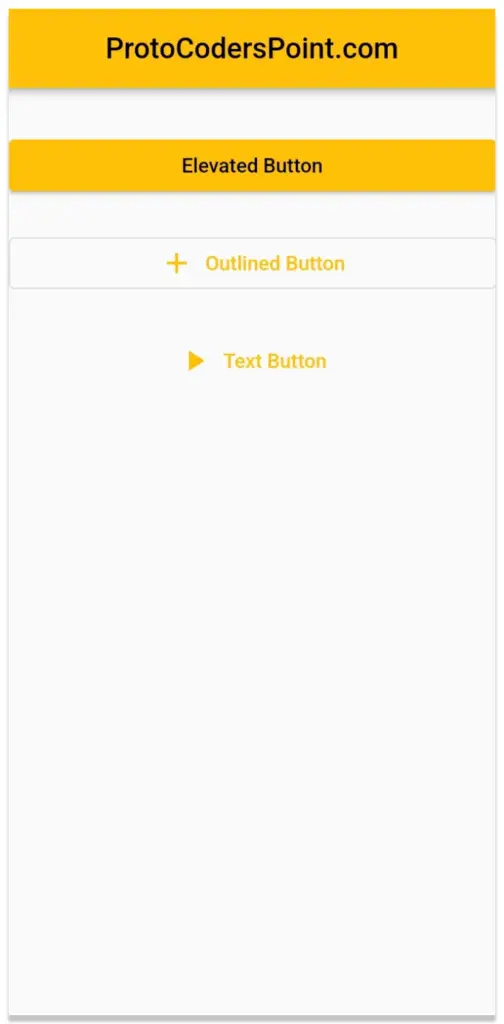
Scaffold( appBar: AppBar(title: const Text('ProtoCodersPoint.com'),centerTitle: true,), body: Padding( padding: const EdgeInsets.symmetric(vertical: 30, horizontal: 10), child: Column( children: [ // Full width button Example 1 SizedBox( width: double.infinity, child: ElevatedButton( onPressed: () {}, child: const Text('Elevated Button'))), const SizedBox( height: 20, ), // Full width button Example 2 Container( width: double.infinity, color: Colors.transparent, child: OutlinedButton.icon( onPressed: () {}, icon: const Icon(Icons.add), label: const Text('Outlined Button')), ), const SizedBox( height: 20, ), // Full width button 3 SizedBox( width: double.infinity, child: TextButton.icon( onPressed: () {}, icon: const Icon(Icons.play_arrow), label: const Text('Text Button')), ), ], ), ), );
2.ConstrainedBox to give Full Width to Button widget
The another way to give full width to button widget is to wrap Elevated Button or any other button with ConstrainedBox & use it’s constraints property with BoxConstraints & set minWidth to double.infinity.
Code:-
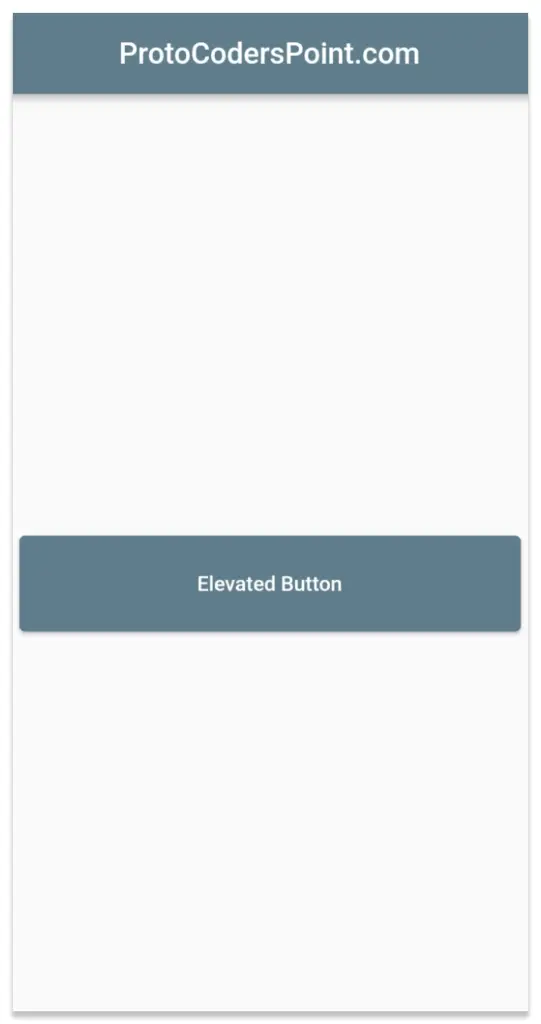
Scaffold( appBar: AppBar(title: const Text('ProtoCodersPoint.com'),centerTitle: true,), body: Padding( padding: const EdgeInsets.symmetric(vertical: 30, horizontal: 10), child: Center( child: ConstrainedBox( constraints: const BoxConstraints( minWidth: double.infinity, minHeight: 65), child: ElevatedButton( onPressed: () {}, child: const Text('Elevated Button'), )), ), ), );
3. Material Button Full Width Flutter
In Flutter material button, We can make it responsive & acquire full width by setting material button property minWidth to double.infinity.
Code:-
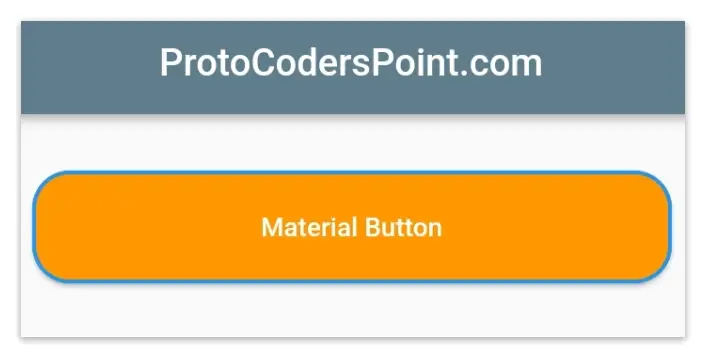
Scaffold( appBar: AppBar(title: const Text('ProtoCodersPoint.com'),centerTitle: true,), body: Padding( padding: const EdgeInsets.symmetric(vertical: 30, horizontal: 10), child: Column( children: [ MaterialButton( shape: RoundedRectangleBorder ( borderRadius: BorderRadius.circular(20.0), side: BorderSide( width: 2, color: Colors.blue ) ), minWidth: double.infinity, height: 60, color: Colors.orange, textColor: Colors.white, onPressed: () {}, child: const Text('Material Button')), ], ), ), );
4. Elevated Button / TextButton set minimumSize
In ElevatedButton or any other button in flutter like (TextButton / OutlineButton), if you use style property with styleForm & set minimumSize parameter to Size.fromHeight (value), Here value is button height, then the button will acquire full width match to it’s parent.
Code:-
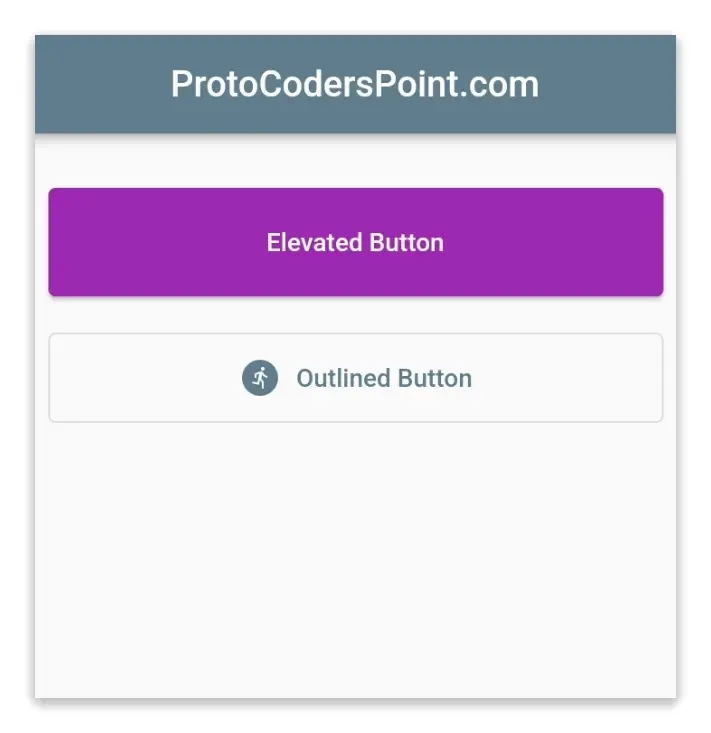
Scaffold( appBar: AppBar(title: const Text('ProtoCodersPoint.com'),centerTitle: true,), body: Padding( padding: const EdgeInsets.symmetric(vertical: 30, horizontal: 10), child: Column( children: [ ElevatedButton( style: ElevatedButton.styleFrom( // The width will be 100% of the parent widget // The height will be 60 minimumSize: const Size.fromHeight(60), backgroundColor: Colors.purple,), onPressed: () {}, child: const Text('Elevated Button')), const SizedBox(height: 20), OutlinedButton.icon( style: OutlinedButton.styleFrom( // the height is 50, the width is full minimumSize: const Size.fromHeight(50), ), onPressed: () {}, icon: const Icon(Icons.run_circle), label: const Text('Outlined Button')), ], ), ), );