In this flutter article, I’m excited to share an overview/baisc of what I have learnt whileworking with BLE in flutter application will provide you with flutter BLE code example with practical code simple, This flutter article on BLE might be a game changer.
Working with (Bluetooth Low Energy) BLE devices in flutter
Video Tutorial – BLE SCANNER
Flutter Blue Plus
A most popular package to work with Bluetooh Device in flutter is flutter_blue_plus package that provides Bluetooth Low Energy (BLE) functionality by using this package we can scan nearby bluetooth devices, connect with them & communicate with them.
Here is a step by step guide on how to work with BLE devices in flutter using flutter_blue_plus package.
Firstly we need to add BLE package into our flutter project follow below steps
1. Open pubspec.yaml file and under dependence section add
dependencies: flutter_blue_plus: ^1.16.2
2. Once package added you need to run below cmd to download the package as external dependencies
flutter pub get
3. To use flutter blue plue you need to import where required
import 'package:flutter_blue_plus/flutter_blue_plus.dart';
Note:- Make sure you ask app users to accept bluetooth usage permission by prompt. Once users accepts the permission, you can start using mobile bluetooth into you flutter application.
Scan from BLE devices
Below is a snippet code to scan nearby BT devices
FlutterBluePlus flutterBlue = FlutterBluePlus.instance; // Listens to BLE devices flutterBlue.scanResults.listen((results){ // Hanlde discovered ble devices here });
Connect to the devices
Once you find the available devices nearby you can connect to that particular devices using below method.
void connectToDevice(BluetoothDevice device) async{ await device.connect(); }
Discover Services list
Don you know? each BLE devices has list of service that the device can provide, by connecting to in we can discover the list of services it has in it.
To fetch all the list of services that device has use below snippet code.
final services await device.discoverServices();
BLE Characteristics
Each services in BLE devices has list of it own characteristics, which is used to communicate with devices based on how it been manufactured. Fore Example:- Generic Access Services has list of BLS characteristics such as device name, MAC address etc.
Below is snippet code to list out characteristic of a services
for(var service in services){ List<BlueToothCharacterictic> characteristics = service.characteristics; }
Read Characteristics in BLE devices
Reading charactericistic retrive data from BT devices.
Create a Bluetooth Charactericistic Object then use read() method to perform read operation make sure to use await as read() is a asynchronous nature.
Snippet
BlueToothCharacterictic characteristics; List<int> value = await characteristics.read(); //handle the value as needed print(`Read Value: ${value.toString()}`);
Write Characteristics in BLE devices
Sending data to BLE devices to done by using write characteristics, We make use of write() methods make sure you use await with write() method.
You need to prepare the data to be sent as alist of integers, After the write operation you can listen for notification just to confirm that write was successful.
List<int> dataToSend = [0x01,0x02,0x03]; //write the data to characteristic await characteristics.write(dataToSend);
Flutter BLE Scanner
Below is a source code, just an example how to implement BLE Scanner into Flutter Application
code
add dependencies in pubspec.yaml file
flutter_blue: get: ^4.6.5 permission_handler:
ble_controller.dart
The below Code have 2 main methods, One for scanning near by BLE ( Bluetooth Devices ) and another method is used for connecting to BLE devices.
import 'package:flutter_blue/flutter_blue.dart'; import 'package:get/get.dart'; import 'package:permission_handler/permission_handler.dart'; class BleController extends GetxController{ FlutterBlue ble = FlutterBlue.instance; // This Function will help users to scan near by BLE devices and get the list of Bluetooth devices. Future scanDevices() async{ if(await Permission.bluetoothScan.request().isGranted){ if(await Permission.bluetoothConnect.request().isGranted){ ble.startScan(timeout: Duration(seconds: 15)); ble.stopScan(); } } } // This function will help user to connect to BLE devices. Future<void> connectToDevice(BluetoothDevice device)async { await device?.connect(timeout: Duration(seconds: 15)); device?.state.listen((isConnected) { if(isConnected == BluetoothDeviceState.connecting){ print("Device connecting to: ${device.name}"); }else if(isConnected == BluetoothDeviceState.connected){ print("Device connected: ${device.name}"); }else{ print("Device Disconnected"); } }); } Stream<List<ScanResult>> get scanResults => ble.scanResults; }
main.dart
import 'package:ble_scanner_app/ble_controller.dart'; import 'package:flutter/material.dart'; import 'package:flutter_blue/flutter_blue.dart'; import 'package:get/get.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple), useMaterial3: true, ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({super.key}); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text("BLE SCANNER"),), body: GetBuilder<BleController>( init: BleController(), builder: (BleController controller) { return Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ StreamBuilder<List<ScanResult>>( stream: controller.scanResults, builder: (context, snapshot) { if (snapshot.hasData) { return Expanded( child: ListView.builder( shrinkWrap: true, itemCount: snapshot.data!.length, itemBuilder: (context, index) { final data = snapshot.data![index]; return Card( elevation: 2, child: ListTile( title: Text(data.device.name), subtitle: Text(data.device.id.id), trailing: Text(data.rssi.toString()), onTap: ()=> controller.connectToDevice(data.device), ), ); }), ); }else{ return Center(child: Text("No Device Found"),); } }), SizedBox(height: 10,), ElevatedButton(onPressed: () async { controller.scanDevices(); // await controller.disconnectDevice(); }, child: Text("SCAN")), ], ), ); }, ) ); } }
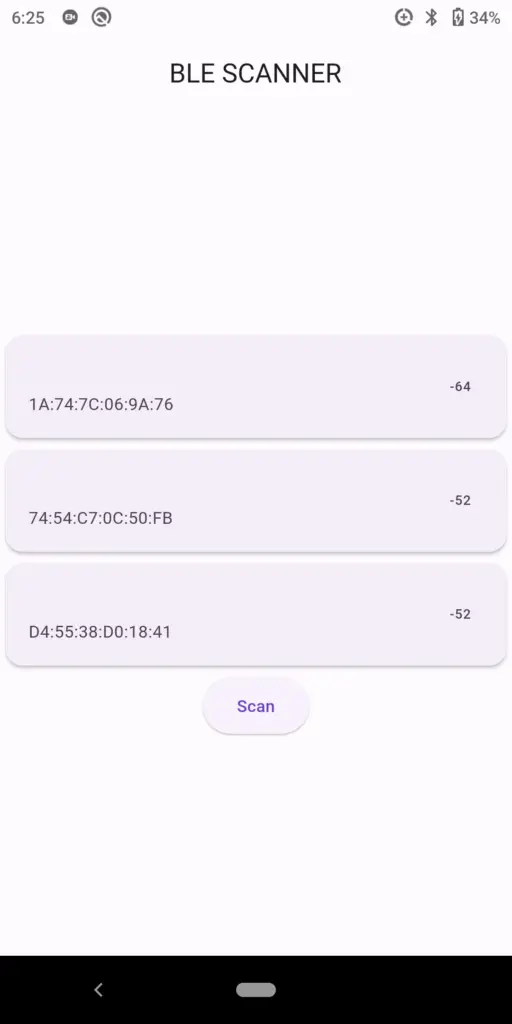
Conclusion
In this article, we learnt basic of BLE in flutter like how to discover list of BLE devices nearby, connecting to BLE Devices fetching services characteristic , reading & writing to device protocal in flutter application using flutter_blue_plus package.