Hi Guys, Welcome to Proto Coders Point, In this android tutorial we will create an android login and Registration form using volley library and phpmyadmin as my database server.
How to keep user logged in android?
In this Android Project i am making used of sharedPreferences to store data of successful logged in uses so that we can keep the user logged in until the user manually logout himself.
Things required for this android mini project.
1. A server: with phpmyadmin installed for database holding.
2. A Hosting: where we will store our server code (.php codes)
3. Android Studio
In this Android Mini Project we gonna make use of Volley Library to Store and Retrieve data form database server using php codes.
Let’s begin creating login and registration form in android studio using volley library
Create a new Android project in android studio
Then, Let’s add the required dependencies in our android project.
Dependencies needed
implementation 'com.google.android.material:material:1.0.0' //material design implementation 'com.android.volley:volley:1.1.0' //volley library
Add a network configuration xml file
Create a new directory named ‘xml‘ under res directory
right click on res > New > Directory > name it as 'xml'
Now under xml folder/directory create a new XML resource file named “network_security_config.xml” and Copy Page the below lines of network confiq code.
<?xml version="1.0" encoding="utf-8"?> <network-security-config> <domain-config cleartextTrafficPermitted="true"> <domain includeSubdomains="true">protocoderspoint.com</domain> //here replace with you localhost server or your domain name </domain-config> </network-security-config>
Here you need you replace protocoderspoint.com with your localhost ip address or with your domain address.
Adding Internet Permission
Then, Open AndroidManifest.xml and add the <uses-permission INTERNET />
<uses-permission android:name="android.permission.INTERNET"/>
OK, Now all the basic requirement for this android project is been added.
Login & Registration form in android using volley library.
For this project we need total 3 Activitys and 3 xml files
1. RegistrationActivity
The RegistrationActivity is where user will be able to register himself to get access for the further feature of any android application
2. LoginActivity
After the user Successfully register himself with the app, now a the user is the part of our application services and his data is registered with us in our phpmyadmin database, now the user can easily Login through the app using his login credential.
3. MainActivity
MainActivity is somewhat like any application home page or dashboard.
UI DESIGN for Login, Registration and MainActivity
button_design.xml
create a new Drawable Resource file in drawable folder and name it as button_design.xml and add the following code.
<shape xmlns:android="http://schemas.android.com/apk/res/android"> <solid android:color="@color/colorPrimary" /> <corners android:radius="40dp" /> <stroke android:width="1dp" android:color="#FFE503" /> </shape>
activity_registration.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@drawable/background" android:orientation="vertical" tools:context=".RegistrationActivity"> <TextView android:layout_margin="10dp" android:gravity="center" android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="25sp" android:textColor="@android:color/white" android:text="Registration Page"/> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="60dp" android:layout_gravity="center" android:layout_margin="20dp" style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox" android:gravity="center" android:hint="Name" android:textColor="@android:color/white"> <com.google.android.material.textfield.TextInputEditText android:id="@+id/rname" android:layout_width="match_parent" android:layout_height="match_parent" android:inputType="text" android:textColor="@android:color/white" android:lines="1" android:maxLines="1"/> </com.google.android.material.textfield.TextInputLayout> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="60dp" android:layout_gravity="center" android:layout_marginTop="10dp" android:layout_marginRight="5dp" android:layout_marginBottom="10dp" style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox" android:gravity="center" android:hint="username" android:layout_margin="20dp" android:textColor="@android:color/white"> <com.google.android.material.textfield.TextInputEditText android:id="@+id/rusername" android:layout_width="match_parent" android:layout_height="match_parent" android:inputType="text" android:textColor="@android:color/white" android:lines="1" android:maxLines="1"/> </com.google.android.material.textfield.TextInputLayout> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="60dp" android:layout_gravity="center" android:layout_marginTop="10dp" android:layout_marginRight="5dp" android:layout_marginBottom="10dp" style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox" android:gravity="center" android:layout_margin="20dp" android:hint="Password" android:textColor="@android:color/white"> <com.google.android.material.textfield.TextInputEditText android:id="@+id/rpassword" android:layout_width="match_parent" android:layout_height="match_parent" android:textColor="@android:color/white" android:inputType="textPassword" android:lines="1" android:maxLines="1"/> </com.google.android.material.textfield.TextInputLayout> <Button android:id="@+id/rregister" android:layout_width="150dp" android:layout_height="wrap_content" android:layout_gravity="center" android:textColor="@android:color/white" android:background="@drawable/button_design" android:text="Register"/> </LinearLayout>
activity_login.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="@drawable/background" android:orientation="vertical" tools:context=".RegistrationActivity"> <TextView android:layout_margin="10dp" android:gravity="center" android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="25sp" android:textColor="@android:color/white" android:text="Login Page"/> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="60dp" android:layout_gravity="center" android:layout_marginTop="10dp" android:layout_marginRight="5dp" android:layout_marginBottom="10dp" style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox" android:gravity="center" android:hint="username" android:layout_margin="20dp" android:textColor="@android:color/white"> <com.google.android.material.textfield.TextInputEditText android:id="@+id/lusername" android:layout_width="match_parent" android:layout_height="match_parent" android:inputType="text" android:textColor="@android:color/white" android:lines="1" android:maxLines="1"/> </com.google.android.material.textfield.TextInputLayout> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="60dp" android:layout_gravity="center" android:layout_marginTop="10dp" android:layout_marginRight="5dp" android:layout_marginBottom="10dp" style="@style/Widget.MaterialComponents.TextInputLayout.OutlinedBox" android:gravity="center" android:layout_margin="20dp" android:hint="Password" android:textColor="@android:color/white"> <com.google.android.material.textfield.TextInputEditText android:id="@+id/lpassword" android:layout_width="match_parent" android:layout_height="match_parent" android:textColor="@android:color/white" android:inputType="textPassword" android:lines="1" android:maxLines="1"/> </com.google.android.material.textfield.TextInputLayout> <TextView android:id="@+id/registernow" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Register Now" android:layout_gravity="center" android:textSize="15sp" android:layout_marginBottom="20dp" android:textColor="@android:color/white" > </TextView> <Button android:id="@+id/loginbutton" android:layout_width="150dp" android:layout_height="wrap_content" android:layout_gravity="center" android:textColor="@android:color/white" android:background="@drawable/button_design" android:text="Login"/> </LinearLayout>
activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" android:background="@drawable/background" android:gravity="center" android:orientation="vertical"> <TextView android:layout_marginTop="20dp" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Home Page" android:textColor="#FFF" android:textSize="25sp" android:layout_gravity="center" /> <TextView android:layout_marginTop="20dp" android:layout_width="wrap_content" android:layout_height="wrap_content" android:gravity="center" android:text="Welcome to Proto Coders Point" android:textColor="#FFF" android:textSize="25sp" android:layout_gravity="center" /> <TextView android:id="@+id/signinusername" android:layout_marginTop="20dp" android:layout_width="wrap_content" android:layout_height="wrap_content" android:gravity="center" android:text="username" android:textColor="#FFF" android:textSize="25sp" android:layout_gravity="center" /> <LinearLayout android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="vertical" android:layout_margin="20dp" android:layout_gravity="center"> <Button android:id="@+id/logout" android:layout_width="150dp" android:layout_height="wrap_content" android:background="@drawable/button_design" android:text="LogOut" android:layout_gravity="center" android:textColor="@android:color/white" /> </LinearLayout> </LinearLayout>
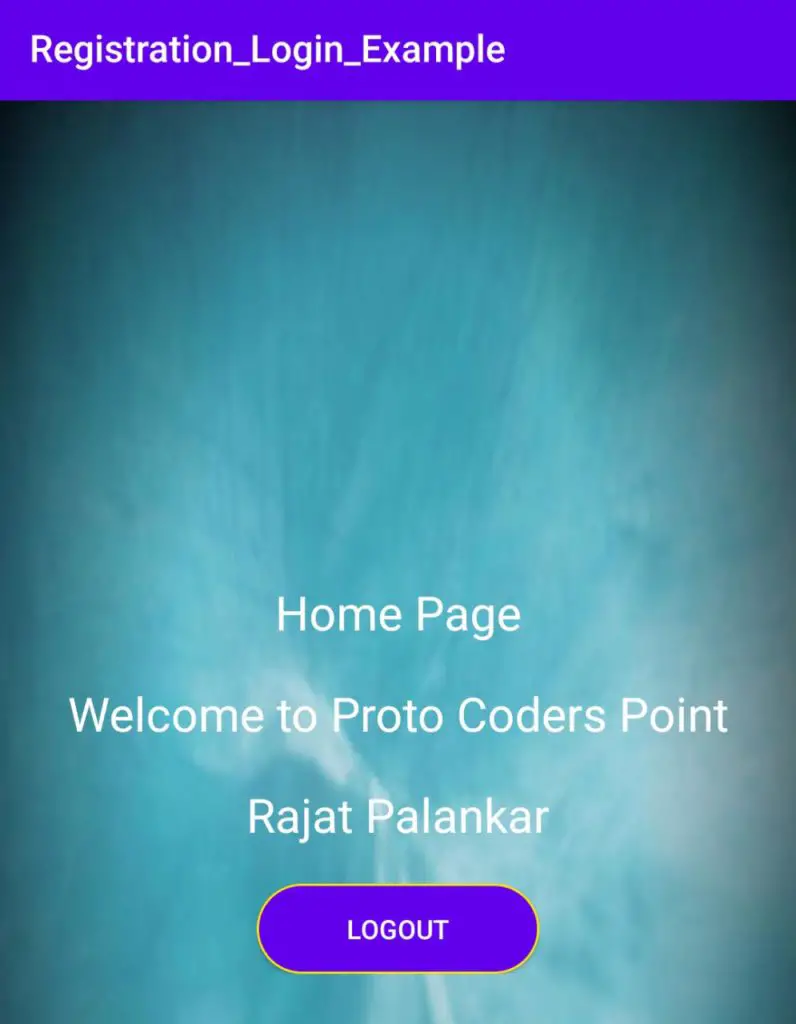
*********Done with UI Design*********
Java Volley Library Snippet
Here is java Volley Snippet code that is used to send and receive data from server.
private void Register() { StringRequest stringRequest = new StringRequest(Request.Method.POST, URL_REGISTER, new Response.Listener<String>() { @Override public void onResponse(String response) { try{ // after success response from server } catch (Exception e) { // if any exception is been cought } } }, new Response.ErrorListener() { @Override public void onErrorResponse(VolleyError error) { // if server fails to response on time } }) { @Override protected Map<String, String> getParams() { Map<String,String> params = new HashMap<>(); // params.put("name",sname); // passing parameters to server return params; } }; RequestQueue requestQueue = Volley.newRequestQueue(this); requestQueue.add(stringRequest); }
The above snippet code is how we send data from application to server and how to deal with the server response.
Here Request.Method.POST describle which Method we gonna use to store or retrieve either GET or POST.
URL_REGISTER : This is the url where out server side code is been kept, for example a url path to the server code.
JAVA CODE for Login,Registration using volley
RegistrationActivity.java
Just change the URL_REGISTER link with your file hosting server path
if you are in localhost your path may be as below :
localhost : “https://192.168.0.10/www/php/registration.php”
else if you have hosting then path you can create
domain: “https://protocoderspoint.com/php/registration.php”
package com.protocoderspoint.registration_login; import androidx.appcompat.app.AppCompatActivity; import android.app.ProgressDialog; import android.content.Intent; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.Toast; import com.android.volley.Request; import com.android.volley.RequestQueue; import com.android.volley.Response; import com.android.volley.VolleyError; import com.android.volley.toolbox.StringRequest; import com.android.volley.toolbox.Volley; import com.google.android.material.textfield.TextInputEditText; import org.json.JSONObject; import java.util.HashMap; import java.util.Map; import java.util.Random; public class RegistrationActivity extends AppCompatActivity { TextInputEditText name ,username ,password; Button rregister; String sname,susername,spassword; ProgressDialog pdDialog; String URL_REGISTER = "https://protocoderspoint.com/php/registration.php"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_registration); name=(TextInputEditText)findViewById(R.id.rname); username=(TextInputEditText)findViewById(R.id.rusername); password=(TextInputEditText)findViewById(R.id.rpassword); rregister=(Button) findViewById(R.id.rregister); pdDialog= new ProgressDialog(RegistrationActivity.this); pdDialog.setTitle("Registering please wait..."); pdDialog.setCancelable(false); rregister.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { sname=name.getText().toString().trim(); susername=username.getText().toString().trim(); spassword=password.getText().toString().trim(); if(sname.isEmpty()||susername.isEmpty()||spassword.isEmpty()) { Toast.makeText(RegistrationActivity.this,"please enter valid data",Toast.LENGTH_SHORT).show(); } else{ Register(); } } }); } private void Register() { pdDialog.show(); StringRequest stringRequest = new StringRequest(Request.Method.POST, URL_REGISTER, new Response.Listener<String>() { @Override public void onResponse(String response) { Log.e("anyText",response); try{ JSONObject jsonObject = new JSONObject(response); String success = jsonObject.getString("success"); String message = jsonObject.getString("message"); if(success.equals("1")){ Toast.makeText(getApplicationContext(),"Registration Success",Toast.LENGTH_LONG).show(); pdDialog.dismiss(); Intent login = new Intent(RegistrationActivity.this,LoginActivity.class); startActivity(login); finish(); } if(success.equals("0")){ Toast.makeText(getApplicationContext(),message,Toast.LENGTH_LONG).show(); pdDialog.dismiss(); } if(success.equals("3")){ Toast.makeText(getApplicationContext(),message,Toast.LENGTH_LONG).show(); pdDialog.dismiss(); } } catch (Exception e) { e.printStackTrace(); Toast.makeText(getApplicationContext(),"Registration Error !1"+e,Toast.LENGTH_LONG).show(); } } }, new Response.ErrorListener() { @Override public void onErrorResponse(VolleyError error) { pdDialog.dismiss(); Toast.makeText(getApplicationContext(),"Registration Error !2"+error,Toast.LENGTH_LONG).show(); } }) { @Override protected Map<String, String> getParams() { Map<String,String> params = new HashMap<>(); params.put("name",sname); params.put("username",susername); params.put("password",spassword); return params; } }; RequestQueue requestQueue = Volley.newRequestQueue(this); requestQueue.add(stringRequest); } }
LoginActivity.java
package com.protocoderspoint.registration_login; import androidx.appcompat.app.AppCompatActivity; import android.app.ProgressDialog; import android.content.Intent; import android.content.SharedPreferences; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.TextView; import android.widget.Toast; import com.android.volley.Request; import com.android.volley.RequestQueue; import com.android.volley.Response; import com.android.volley.VolleyError; import com.android.volley.toolbox.StringRequest; import com.android.volley.toolbox.Volley; import com.google.android.material.textfield.TextInputEditText; import org.json.JSONObject; import java.util.HashMap; import java.util.Map; public class LoginActivity extends AppCompatActivity { TextView Registernow; ProgressDialog pdDialog; String URL_LOGIN = "https://protocoderspoint.com/php/login.php"; String luser,lpass; TextInputEditText username,password; Button loginButton; String is_signed_in=""; SharedPreferences mPreferences; String sharedprofFile="com.protocoderspoint.registration_login"; SharedPreferences.Editor preferencesEditor; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_login); mPreferences=getSharedPreferences(sharedprofFile,MODE_PRIVATE); preferencesEditor = mPreferences.edit(); is_signed_in = mPreferences.getString("issignedin","false"); if(is_signed_in.equals("true")) { Intent i = new Intent(LoginActivity.this,MainActivity.class); startActivity(i); finish(); } Registernow =(TextView)findViewById(R.id.registernow); pdDialog= new ProgressDialog(LoginActivity.this); pdDialog.setTitle("Login please wait..."); pdDialog.setCancelable(false); mPreferences=getSharedPreferences(sharedprofFile,MODE_PRIVATE); preferencesEditor = mPreferences.edit(); username = (TextInputEditText) findViewById(R.id.lusername); password = (TextInputEditText)findViewById(R.id.lpassword); loginButton=(Button) findViewById(R.id.loginbutton); Registernow.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent register=new Intent(LoginActivity.this,RegistrationActivity.class); startActivity(register); } }); loginButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { luser=username.getText().toString().trim(); lpass=password.getText().toString().trim(); if(luser.isEmpty()||lpass.isEmpty()) { Toast.makeText(LoginActivity.this,"please enter valid data",Toast.LENGTH_SHORT).show(); }else { Login(); } } }); } private void Login() { pdDialog.show(); final StringRequest stringRequest = new StringRequest(Request.Method.POST, URL_LOGIN, new Response.Listener<String>() { @Override public void onResponse(String response) { Log.e("anyText",response); try{ JSONObject jsonObject = new JSONObject(response); String success = jsonObject.getString("success"); String message = jsonObject.getString("message"); String id= jsonObject.getString("id"); String name = jsonObject.getString("name"); String username = jsonObject.getString("username"); if(success.equals("1")){ Toast.makeText(getApplicationContext(),"Logged In Success",Toast.LENGTH_LONG).show(); pdDialog.dismiss(); preferencesEditor.putString("issignedin","true"); preferencesEditor.putString("SignedInUserID",id); preferencesEditor.putString("SignedInName",name); preferencesEditor.putString("SignedInusername",username); preferencesEditor.apply(); Intent i = new Intent(LoginActivity.this,MainActivity.class); startActivity(i); finish(); } if(success.equals("0")){ Toast.makeText(getApplicationContext(),message,Toast.LENGTH_LONG).show(); pdDialog.dismiss(); } if(success.equals("3")){ Toast.makeText(getApplicationContext(),message,Toast.LENGTH_LONG).show(); pdDialog.dismiss(); } } catch (Exception e) { e.printStackTrace(); Toast.makeText(getApplicationContext(),"Registration Error !1"+e,Toast.LENGTH_LONG).show(); } } }, new Response.ErrorListener() { @Override public void onErrorResponse(VolleyError error) { pdDialog.dismiss(); Toast.makeText(getApplicationContext(),"Registration Error !2"+error,Toast.LENGTH_LONG).show(); } }) { @Override protected Map<String, String> getParams() { Map<String,String> params = new HashMap<>(); params.put("username",luser); params.put("password",lpass); return params; } }; RequestQueue requestQueue = Volley.newRequestQueue(this); requestQueue.add(stringRequest); } }
MainActivity.java
MainActivity is the page where only successful logged in user can reach.
package com.protocoderspoint.registration_login; import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.content.SharedPreferences; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.TextView; public class MainActivity extends AppCompatActivity { SharedPreferences mPreferences; String sharedprofFile="com.protocoderspoint.registration_login"; SharedPreferences.Editor preferencesEditor; String id, name, username; Button logout; TextView Signedinusername; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mPreferences=getSharedPreferences(sharedprofFile,MODE_PRIVATE); preferencesEditor = mPreferences.edit(); logout = (Button)findViewById(R.id.logout); Signedinusername = (TextView)findViewById(R.id.signinusername); id=mPreferences.getString("SignedInUserID","null"); name=mPreferences.getString("SignedInName","null"); username = mPreferences.getString("SignedInusername","null"); Signedinusername.setText(name); logout.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { preferencesEditor.putString("issignedin","false"); preferencesEditor.apply(); Intent loginscreen = new Intent(MainActivity.this, LoginActivity.class); startActivity(loginscreen); } }); } }
********* Done with JAVA CODE *********
php server side code
Create a folder in your hosting name it as “php” and add all below 3 php code in that folder
connect.php
<?php $conn = mysqli_connect("localhost", "phpmyadmin username", "your php my admin password", "database name"); if($conn) { //echo "Connection Success"; } else { //echo "Connection Failed"; } ?>
registration.php
<?php $name = $_POST["name"]; $username= $_POST["username"]; $password = $_POST["password"]; require_once 'connect.php'; $findexist="select * from registered where username='$username'"; $resultsearch=mysqli_query($conn,$findexist); if(mysqli_num_rows($resultsearch)>0) { while($row=mysqli_fetch_array($resultsearch)) { $result["success"] = "3"; $result["message"] = "user Already exist"; echo json_encode($result); mysqli_close($conn); } } else{ $sql = "INSERT INTO registered (name,username,password) VALUES ('$name','$username','$password');"; if ( mysqli_query($conn, $sql) ) { $result["success"] = "1"; $result["message"] = "Registration success"; echo json_encode($result); mysqli_close($conn); } else { $result["success"] = "0"; $result["message"] = "error in Registration"; echo json_encode($result); mysqli_close($conn); } } ?>
login.php
<?php ini_set('display_errors', 1); ini_set('display_startup_errors', 1); error_reporting(E_ALL); $username= $_POST["username"]; $password = $_POST["password"]; require_once 'connect.php'; $list=""; $query="select * from registered where username='$username' AND password='$password'"; $result=mysqli_query($conn,$query); if(mysqli_num_rows($result)>0) { while($row=mysqli_fetch_array($result)) { if($list=="") { $id=$row['id']; $name=$row['name']; $username=$row['username']; } } } if(mysqli_num_rows($result)==0) { $response["success"] = "0"; $response["message"]="user is not Registered, Please Register"; echo json_encode($response); mysqli_close($conn); } else { //response to android app $response["success"]="1"; $response["message"]="Logged in successful"; $response["id"]=$id; $response["name"]=$name; $response["username"]=$username; //converting response data into json format echo json_encode($response); mysqli_close($conn); } ?>
********* Done with PHP CODE*********
Database in phpmyadmin server
login into your phpmyadmin and create a new database > give a name to your database of your choose in my case its u766503104_protoserver
Then, create new table named “registred” with 4 column id,name,username,password as shown in above screenshot.
All set now your android app is ready to play with server
- it can register user using phpmyadmin.
- it can make registered user to login to the app.
- even the android app keep login session of the user
Download complete login registration source project from google drive
Download from Google Drive