Hi Guys, Welcome to Proto Coders Point, In this Flutter tutorial we gonna learn how to use the image picker flutter library a package that helps getimage/flutter pick images from a gallery or camera.
Image Picker flutter library
A Flutter Plugin library for both iOS and Android that is been used to pick an images from mobile phone gallery or even you can take a new photo with the camera.
let’s straight away start implement the flutter image picker in our flutter app.
find the latest version of Image picker from official site.
VIDEO TUTORIAL
Step 1: Adding image picker dependency flutter in to our flutter project
Once you have created a new Flutter project or open your existing flutter project to implement image picker flutter library.
Then you need to add image picker dependencies,
Open pubspec.yaml then add the below lines
dependencies: image_picker: ^0.6.3+4 //add this line in pubspec.yaml file
Step 2: Import image_picker.dart file in main.dart
Now, as you have added the required library in your project now you need to import the picker library wherever required.
import 'package:image_picker/image_picker.dart';
For Example : In my case i have imported the library in main.dart file whcih is my main activity screen.
Step 3: Configuration
1. ANDROID IMAGE PICKER CONFIGURATION
When it comes to android their is no configuration required – the plugin should work out of the box.
Just we need to set request Legacy External Storage to true Android > app > src > main >AndroidManifest.xml
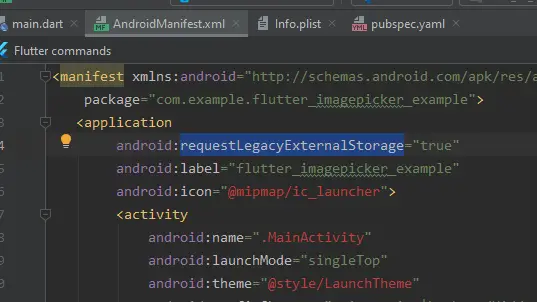
By adding the above request Legacy External Strorage the app will auto ask user to grant permission to make use of storage.
2. IOS IMAGE PICKER CONFIGURATION
Add the following keys to your Info.plist file, located in
Project > ios > Runner > Info.plist
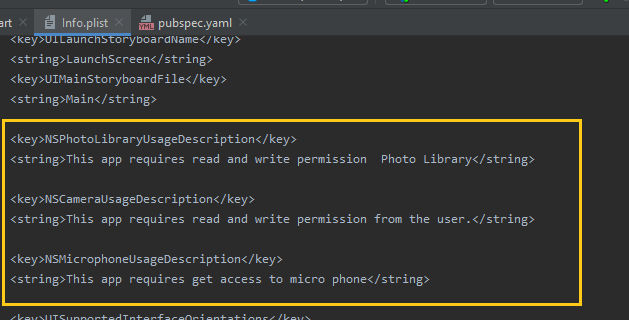
Copy paste the below permission in info.plist
<key>NSPhotoLibraryUsageDescription</key> <string>This app requires read and write permission Photo Library</string>
used to ask uses to give permission for accessing photo library. This is Called Privacy – Photo Library Usage Description.
<key>NSCameraUsageDescription</key> <string>This app requires read and write permission from the user.</string>
Used to take photo from device camera itself.
<key>NSMicrophoneUsageDescription</key> <string>This app requires get access to micro phone</string>
if you intend to record an video by using camera, video offcourse need audio to be recorded, this permission is used to record audio from microphone.
Step4 : Explaination of Image Picker snippet code
File _image; //here we gonna store our image.
var image = await ImagePicker.pickImage(source: ImageSource.gallery);
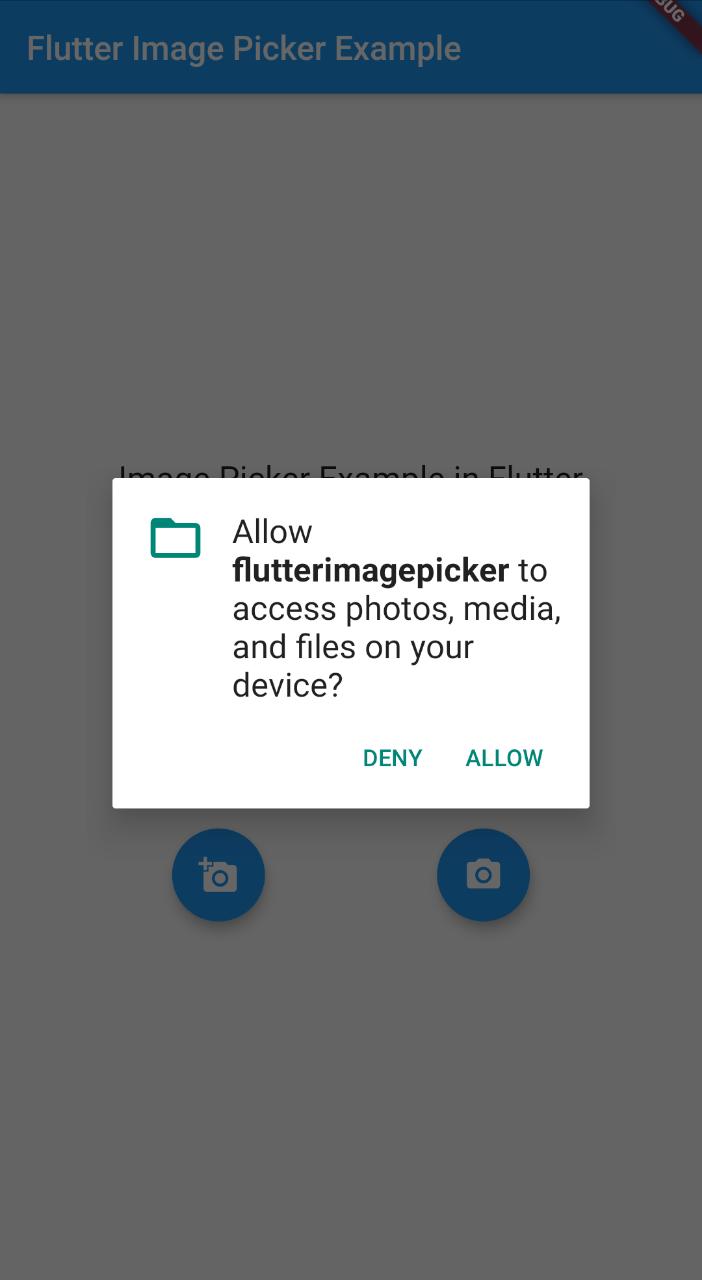
Then, Here we are using ImagePicker Class to pick images from ImageSource.
var image = await ImagePicker.pickImage(source: ImageSource.camera);
Here, ImageSource can either be camera or gallery.
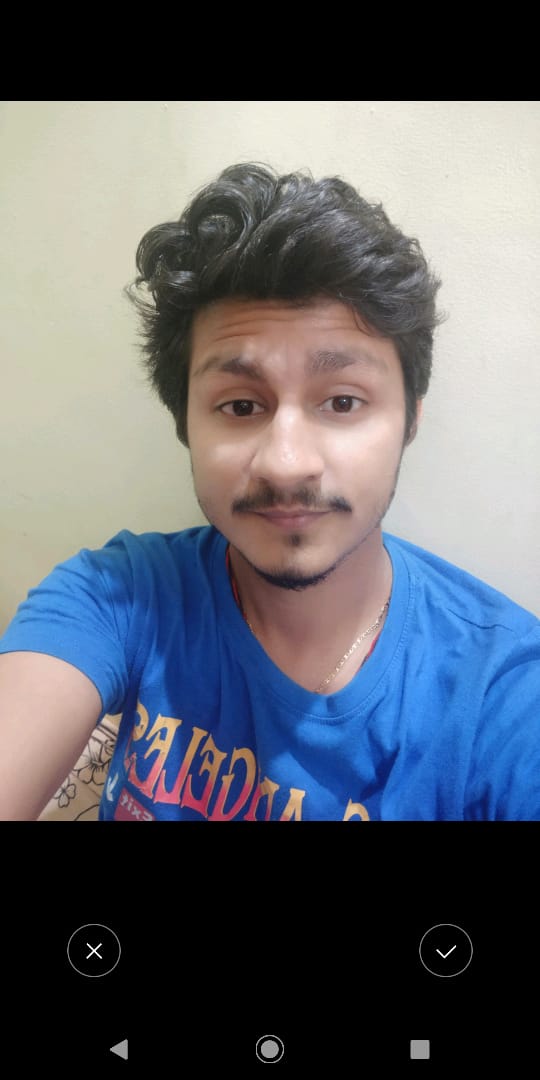
Camera is you want to click a image from device camera.
Gallery is you want to pick/select image from gallery of mobile phone.
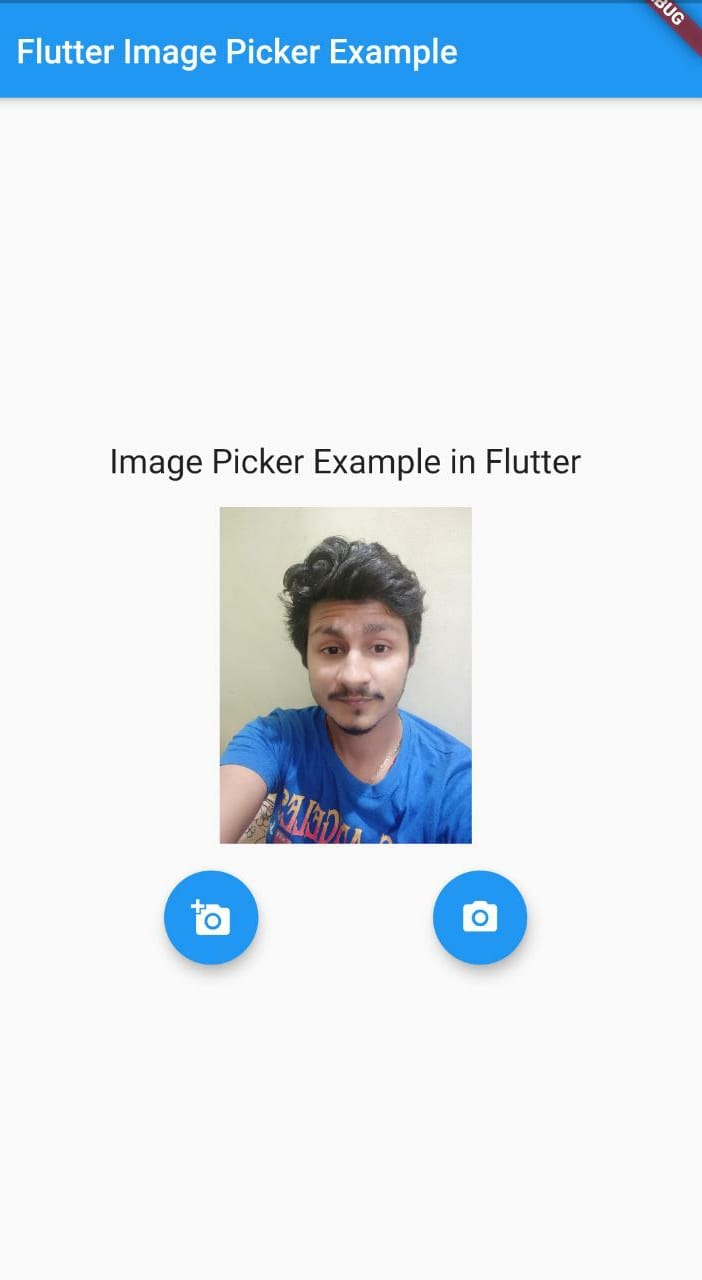
Complete Code for image picker in flutter
main.dart
import 'dart:ffi'; import 'dart:io'; import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'package:image_picker/image_picker.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { File _image; Future getImagefromcamera() async { var image = await ImagePicker.pickImage(source: ImageSource.camera); setState(() { _image = image; }); } Future getImagefromGallery() async { var image = await ImagePicker.pickImage(source: ImageSource.gallery); setState(() { _image = image; }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Flutter Image Picker Example"), ), body: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Center( child: Text( "Image Picker Example in Flutter", style: TextStyle(fontSize: 20), ), ), Padding( padding: const EdgeInsets.all(16.0), child: Container( width: MediaQuery.of(context).size.width, height: 200.0, child: Center( child: _image == null ? Text("No Image is picked") : Image.file(_image), ), ), ), Row( mainAxisAlignment: MainAxisAlignment.spaceEvenly, children: <Widget>[ FloatingActionButton( onPressed: getImagefromcamera, tooltip: "pickImage", child: Icon(Icons.add_a_photo), ), FloatingActionButton( onPressed: getImagefromGallery, tooltip: "Pick Image", child: Icon(Icons.camera_alt), ) ], ) ], ), ); } }
Here are some best Flutter Library that you can use in your flutter Project
Very useful Libraries in Flutter
Flutter image viewer : Is a simple photo View flutter library using which your app user will be easily able to zoom into the image.
Flutter time picker : A Flutter library that help you in adding date & time picker in your application.
Android Developer Image Libraries
Android Image Filter library : Image processing library is very easy to use as android image filter library.
Image Steps : Can you used as delivery progress indicator.
Flutter Image to base64 string or viceversa base64 to image: Here we have covered 3 example i.e convert image to base64 string, base64 to image, and pick image from gallery and convert it into base64 string.