Hi Guys, Welcome to Proto Coders Point. This Article is on How to Validate email TextField in flutter.
We will use RegExp to validate email textfield, This will be a real time email validation form as user is typing in the form we will check the validation for every string the user enter.
email regex pattern for email validation
r'\S+@\S+\.\S+'
Source Code – Form Email Validation Flutter
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { final GlobalKey<FormState> _formkey = GlobalKey<FormState>(); //TextController to read text entered in text field TextEditingController textEditingControllerEmail = TextEditingController(); @override Widget build(BuildContext context) { return Scaffold( body: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Form( key: _formkey, child: Padding( padding: const EdgeInsets.only(bottom: 15,left: 10,right: 10), child: TextFormField( controller: textEditingControllerEmail, onChanged: (val){ _formkey.currentState?.validate(); }, keyboardType: TextInputType.text, decoration:buildInputDecoration(Icons.email,"Email"), validator: (value){ print(value); if(value!.isEmpty){ return "Please Enter Email"; }else if(!RegExp(r'\S+@\S+\.\S+').hasMatch(value)) { return "Please Enter a Valid Email"; } return null; }, ), ), ), ], ), ); } } // TextFormField Border Decoration InputDecoration buildInputDecoration(IconData icons,String hinttext) { return InputDecoration( hintText: hinttext, prefixIcon: Icon(icons), focusedBorder: OutlineInputBorder( borderRadius: BorderRadius.circular(25.0), borderSide: BorderSide( color: Colors.green, width: 1.5 ), ), border: OutlineInputBorder( borderRadius: BorderRadius.circular(25.0), borderSide: BorderSide( color: Colors.blue, width: 1.5, ), ), enabledBorder:OutlineInputBorder( borderRadius: BorderRadius.circular(25.0), borderSide: BorderSide( color: Colors.blue, width: 1.5, ), ), ); }
I above code, A have created GlobalKey with formState and a TextEditingController using which we can get the text been entered in TextField.
Then In UI I have A Form widget assigned a key to it. The form Widget has children i.e. TextFormField where the user will enter his email address.
In TextFormField will use validator property where will check if the enter text in textField matches with email validate regular expression.
Then will use TextFormField onChanged() and call form.currentState.validate() function to keep checking the text entered by the user.
Result
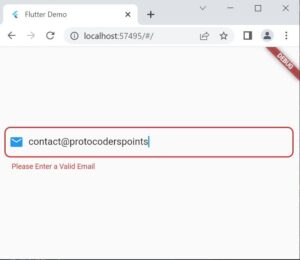
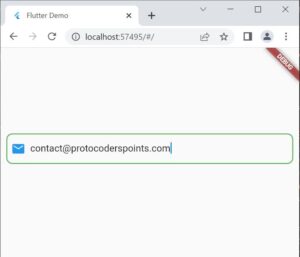
Check out Similar Articles on Validation
Flutter Email Validation using plugin