Hi Guy’s Welcome to Proto Coders Point, In this Flutter Tutorial Will 🚀 Learn to build a Flutter AI Chatbot app using Google’s Gemini AI API, For this we gonna make use of the flutter pacakge named “google_generative_ai“. Explore the magic of gemini ai as we create intelligent chat features in our flutter application . Elevate your Flutter skills and enjoy a step-by-step guide to build an AI-powered chat app! 🤖📱.
Flutter Google Generative AI
To build Flutter AI-Powered chatbot we will make use of a package called “google_generative_ai”, and to communicate with google gemini ai we need a gemini API Key.
Check out the complete video tutorial on how to build AI Chatbot in flutter using gemini AI
How to get Gemini AI API key
Simply check out the above video to get gemini AI API key else click on button below:
Build ChatBot Flutter App
1. Create or open a Flutter project
2. Add dependencies
Open pubspec.yaml file from your flutter project and under depencencies section add below dependencies
dependencies: google_generative_ai: intl:
google generative ai: used to communicate with gemini AI.
intl: used for DataTime Formating.
Basic Information on how to use google generative ai package (code explanation)
This is Snippet Code- Not Complete Code- Find Complete code below with UI design Chat App
final model = GenerativeModel(model: 'gemini-pro', apiKey: apiKey); final content = [Content.text(message)]; final response = await model.generateContent(content);
This above snippet code initializes a GenerativeModel object with the specified model (‘gemini-pro’) and API key. Then, it prepares the content to be sent to the AI model (Basically a Text/prompt message), which consists of a list containing a single text content (message). Finally, it sends this content to the Gemini AI model and awaits the response, which will contain the AI-generated content based on the input message user provide.
Complete Source Code – Building an AI Chatbot in Flutter Using Google’s Generative AI Package
Result/Output of below Code
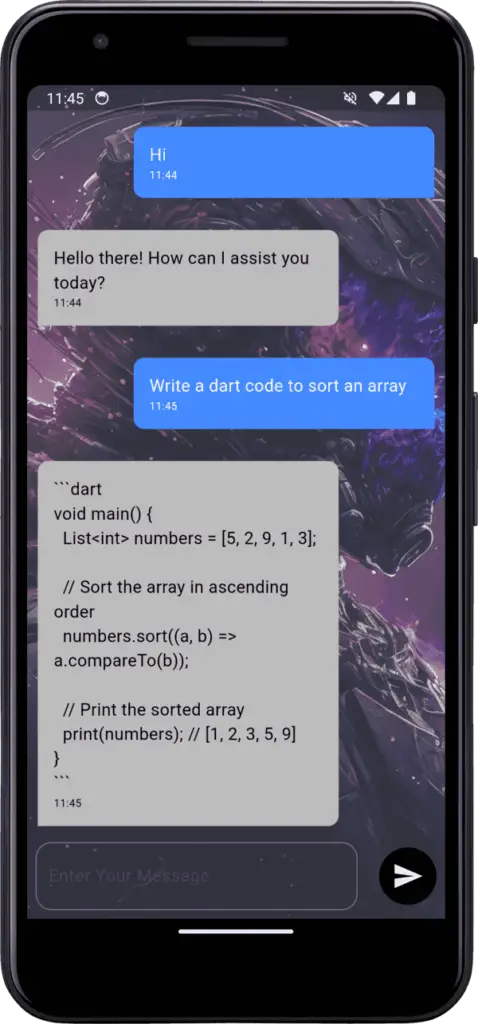
main.dart
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'package:google_generative_ai/google_generative_ai.dart'; import 'package:intl/intl.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', debugShowCheckedModeBanner: false, theme: ThemeData( colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple), useMaterial3: true, ), home: const ChatScreen(), ); } } class ChatScreen extends StatefulWidget { const ChatScreen({super.key}); @override State<ChatScreen> createState() => _ChatScreenState(); } class _ChatScreenState extends State<ChatScreen> { TextEditingController _userInput =TextEditingController(); static const apiKey = "<Replace You Gemini API Key Here>"; final model = GenerativeModel(model: 'gemini-pro', apiKey: apiKey); final List<Message> _messages = []; Future<void> sendMessage() async{ final message = _userInput.text; setState(() { _messages.add(Message(isUser: true, message: message, date: DateTime.now())); }); final content = [Content.text(message)]; final response = await model.generateContent(content); setState(() { _messages.add(Message(isUser: false, message: response.text?? "", date: DateTime.now())); }); } @override Widget build(BuildContext context) { return Scaffold( body: Container( decoration: BoxDecoration( image: DecorationImage( colorFilter: new ColorFilter.mode(Colors.black.withOpacity(0.8), BlendMode.dstATop), image: NetworkImage('https://blogger.googleusercontent.com/img/b/R29vZ2xl/AVvXsEigDbiBM6I5Fx1Jbz-hj_mqL_KtAPlv9UsQwpthZIfFLjL-hvCmst09I-RbQsbVt5Z0QzYI_Xj1l8vkS8JrP6eUlgK89GJzbb_P-BwLhVP13PalBm8ga1hbW5pVx8bswNWCjqZj2XxTFvwQ__u4ytDKvfFi5I2W9MDtH3wFXxww19EVYkN8IzIDJLh_aw/s1920/space-soldier-ai-wallpaper-4k.webp'), fit: BoxFit.cover ) ), child: Column( mainAxisAlignment: MainAxisAlignment.end, children: [ Expanded( child: ListView.builder(itemCount:_messages.length,itemBuilder: (context,index){ final message = _messages[index]; return Messages(isUser: message.isUser, message: message.message, date: DateFormat('HH:mm').format(message.date)); }) ), Padding( padding: const EdgeInsets.all(8.0), child: Row( children: [ Expanded( flex: 15, child: TextFormField( style: TextStyle(color: Colors.white), controller: _userInput, decoration: InputDecoration( border: OutlineInputBorder( borderRadius: BorderRadius.circular(15), ), label: Text('Enter Your Message') ), ), ), Spacer(), IconButton( padding: EdgeInsets.all(12), iconSize: 30, style: ButtonStyle( backgroundColor: MaterialStateProperty.all(Colors.black), foregroundColor: MaterialStateProperty.all(Colors.white), shape: MaterialStateProperty.all(CircleBorder()) ), onPressed: (){ sendMessage(); }, icon: Icon(Icons.send)) ], ), ) ], ), ), ); } } class Message{ final bool isUser; final String message; final DateTime date; Message({ required this.isUser, required this.message, required this.date}); } class Messages extends StatelessWidget { final bool isUser; final String message; final String date; const Messages( { super.key, required this.isUser, required this.message, required this.date }); @override Widget build(BuildContext context) { return Container( width: double.infinity, padding: EdgeInsets.all(15), margin: EdgeInsets.symmetric(vertical: 15).copyWith( left: isUser ? 100:10, right: isUser ? 10: 100 ), decoration: BoxDecoration( color: isUser ? Colors.blueAccent : Colors.grey.shade400, borderRadius: BorderRadius.only( topLeft: Radius.circular(10), bottomLeft: isUser ? Radius.circular(10): Radius.zero, topRight: Radius.circular(10), bottomRight: isUser ? Radius.zero : Radius.circular(10) ) ), child: Column( crossAxisAlignment: CrossAxisAlignment.start, children: [ Text( message, style: TextStyle(fontSize: 16,color: isUser ? Colors.white: Colors.black), ), Text( date, style: TextStyle(fontSize: 10,color: isUser ? Colors.white: Colors.black,), ) ], ), ); } }