Hi Guys, Welcome to Proto Coders Point, Here is a dart program to find product of 2 numbers without using * operator.
Dart program find multiplication of 2 number without * operator
In this dart program, we will not use multiplication operator i.e. *, Instead we will use iteration/loop technique to find the product of 2 number in dart program without * operator.
Product of 2 number program in dart language – Source Code
void main(){ int a = 2, b = 3, result; result =product(a, b); print('$result'); } // calculate multiplication and return the result int product(int a,int b){ int temp =0; while(b != 0){ temp += a; b--; } return temp; }
output
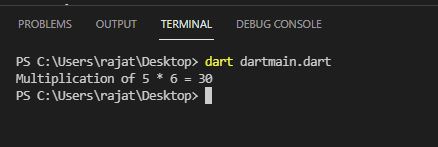
Program Explanation
In the above dart program,
We habe initialize number to 2 variables a & b, & then passing a,b values to a functon ‘product()’, that will perform multiplication without * operator.
In product() function, we have declared a temp variable as ‘0’.
The while loop, check if ‘b’ variable is not equal to ‘0’, if the while condition is true then execute the iteration loop.
So, inside while loop, we are adding the value of ‘temp’ + ‘a’ variable & then decrement value ‘b’ by -1.
There when b == 0, i.e. b !=0 (condition become false)exit the loop, & therefore we have product of a * b in temp variable, which is returned by product function & this we are print multiplication of 2 numbers on console without using dart arithmetic operator.