Here is a Basic Animation in flutter example, I had a container to which I wanted to give a border animation by using decoration> gradient property of container widget and apply animation to it. Here is the result of below flutter animation source code.
Video Tutorial
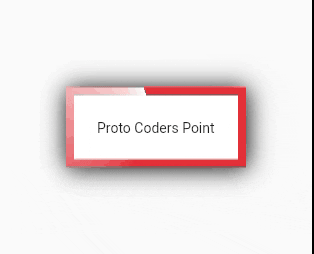
Source Code
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> with SingleTickerProviderStateMixin { late AnimationController _animationController; @override void initState() { // TODO: implement initState super.initState(); _animationController = AnimationController(vsync: this,duration: Duration(seconds: 1)); _animationController..addStatusListener((status) { if(status == AnimationStatus.completed) _animationController.forward(from: 0); }); _animationController.addListener(() { setState(() { }); }); _animationController.forward(); } @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Container( width: 180, height: 80, decoration: BoxDecoration( boxShadow: const[ BoxShadow(offset: Offset(1,1),blurRadius: 20,color: Colors.black) ], gradient: SweepGradient( startAngle: 4, colors: [Colors.red,Colors.white], transform: GradientRotation(_animationController.value*6) ) ), child: Padding( padding: EdgeInsets.all(8.0), child: Container( color: Colors.white, alignment: Alignment.center, child: Text("Proto Coders Point"), ), ), ), ), ); } }