Hi Guys, Welcome to Proto Coders Point.
There are some situation when you want to pass data from one page to another page.
For Example: We Have a listview with a list of data and each item in the list has some data. Eg: Title, a Image & when a user select a item from the list we want to send same selected data to another screen to view in detail.
So In this flutter tutorial example, We will create a listview with items in it & will learn listview onTap selected item send data to next screen.
In other words flutter send data to another page.
Demo
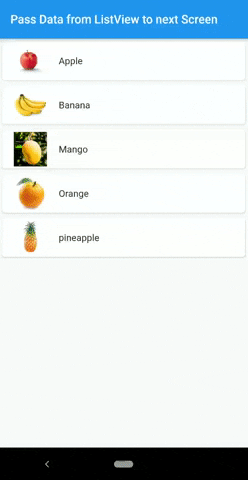
Video Tutorial
So let’s begin with flutter tutorial to send selected data from listview to next page
Flutter Listview onTap on Selected Item – send data to new screen
Here we need to create 2 screens and a DataModel
(Screen 1) main.dart: Will generate list of data and show in listview.
(Screen 2) FruitDetail.dart: User selected item data will be shown here.
(DataModel) FruitDataModel: Holds Information or a data.
Create files as below and add respectively code in it. Refer my project file structure.
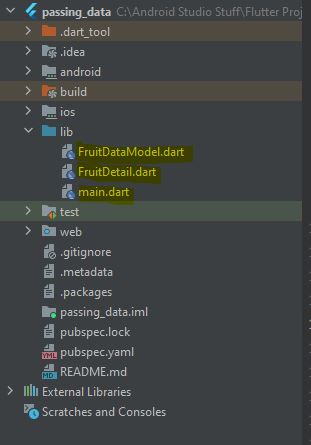
1. FruitDataModel.dart
A Data Model are class of entities that holds information or a data.
In our app, DataModel hold String data.
Eg: Name of Fruit.
ImageUrl of Fruit.
Description of Fruit.
class FruitDataModel{ final String name,ImageUrl,desc; FruitDataModel(this.name, this.ImageUrl, this.desc); }
DataModel will have a constructor to assign the value to variables strings.
2. FruitDetail.dart
This is UI Screen 2 to display detail of Selected Item from the listview.
FruitDetail has a constructor that accept Fruitdata object list of type<FruitDataModel>.
by using the FruitDataModel we can access the data of selected item using indexId. & FruitDataModel class object that holds the details of selected fruit from the listview in main.dart
The FruitDetail page will have UI like Appbar, Image.network to load url images & a Text Widget.
In AppBar will print fruit name.
Image.Network will load Image from url.
Text will show description detail of Fruit.
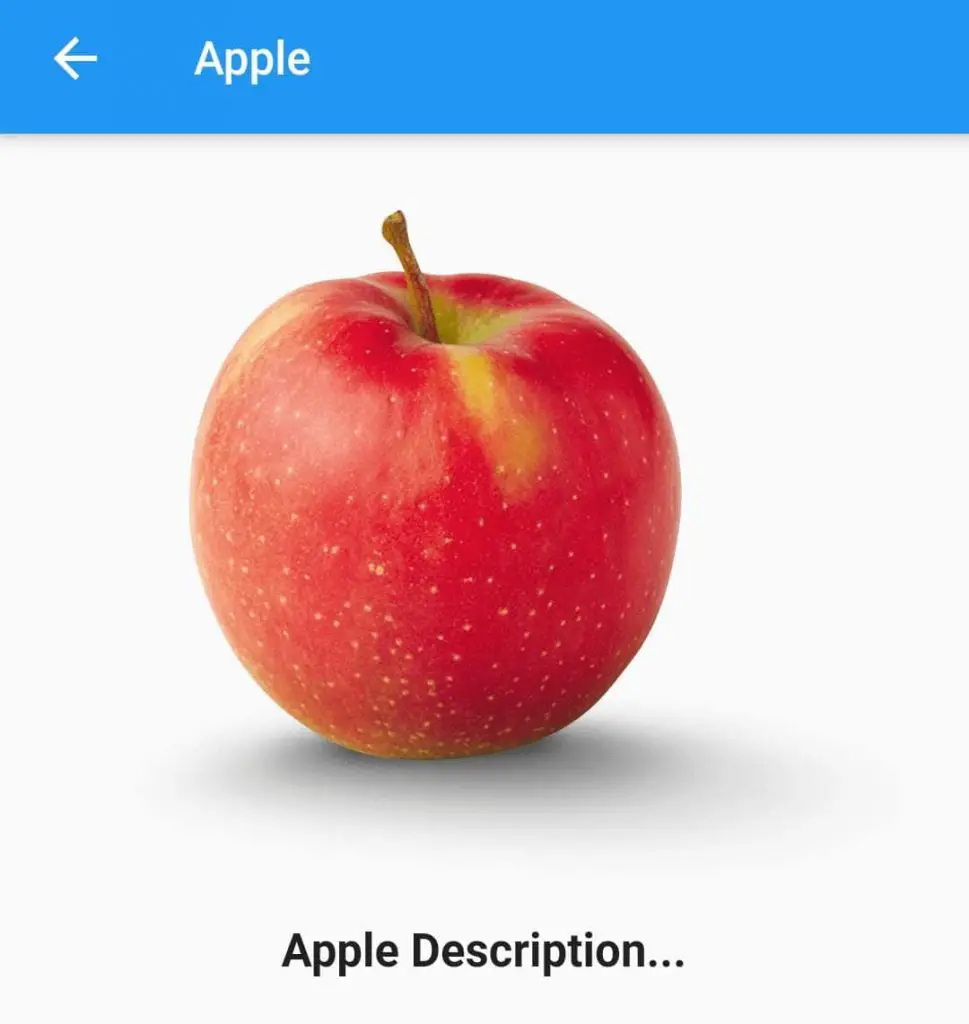
FruitDetail.dart – code
import 'package:flutter/material.dart'; import 'package:passing_data/FruitDataModel.dart'; class FruitDetail extends StatelessWidget { final FruitDataModel fruitDataModel; const FruitDetail({Key? key, required this.fruitDataModel}) : super(key: key); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text(fruitDataModel.name),), body: Column( children: [ Image.network(fruitDataModel.ImageUrl), SizedBox( height: 10, ), Text(fruitDataModel.desc,style: TextStyle(fontWeight: FontWeight.bold,fontSize: 20),) ], ), ); } }
3. main.dart – generate list and display it in listview
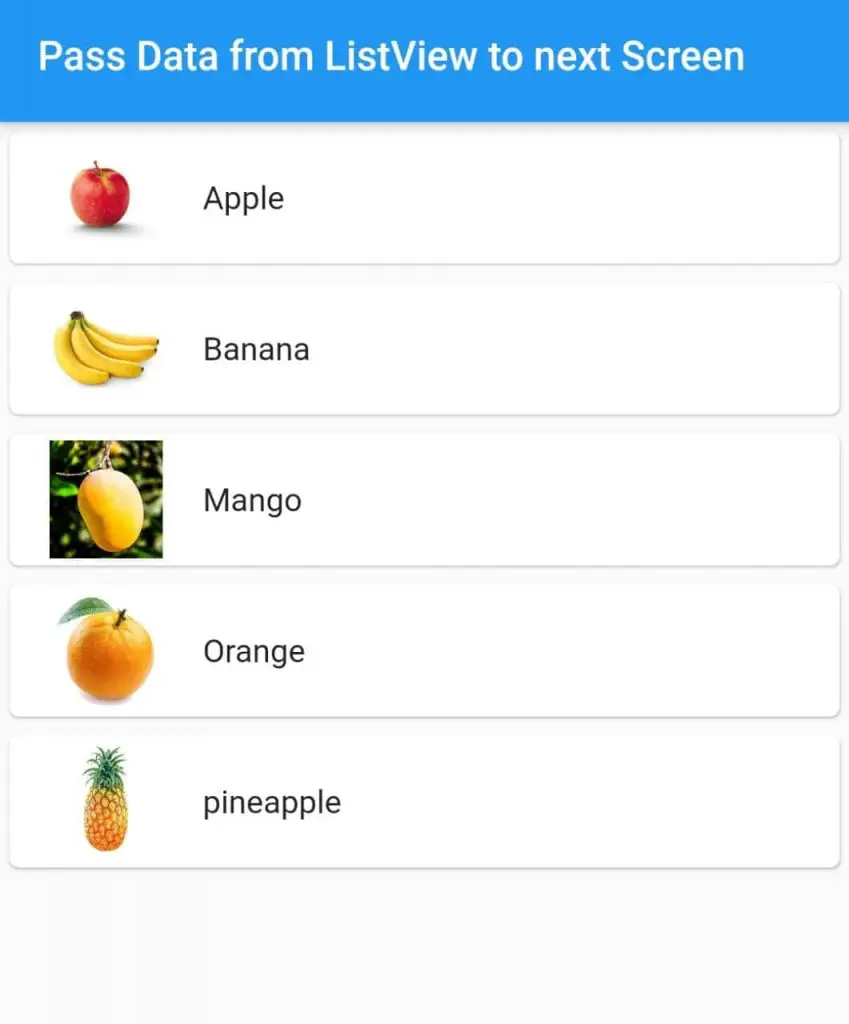
This is the screen 1, Where we will generate static list of data(just for an example) & then show the data in listview.builder.
let’s create list of data(fruit name & imageUrl array list in flutter)
static List<String> fruitname =['Apple','Banana','Mango','Orange','pineapple']; static List url = ['https://www.applesfromny.com/wp-content/uploads/2020/05/Jonagold_NYAS-Apples2.png', 'https://cdn.mos.cms.futurecdn.net/42E9as7NaTaAi4A6JcuFwG-1200-80.jpg', 'https://upload.wikimedia.org/wikipedia/commons/thumb/9/90/Hapus_Mango.jpg/220px-Hapus_Mango.jpg', 'https://5.imimg.com/data5/VN/YP/MY-33296037/orange-600x600-500x500.jpg', 'https://5.imimg.com/data5/GJ/MD/MY-35442270/fresh-pineapple-500x500.jpg'];
In above snippet, We have created list of fruitName & fruit ImageUrl.
Now Let’s merge the array and generate list of data object which will be of type ‘FruitDataModel’.
final List<FruitDataModel> Fruitdata = List.generate( fruitname.length, (index) => FruitDataModel('${fruitname[index]}', '${url[index]}','${fruitname[index]} Description...'));
above ‘Fruitdata’ list object will hold each fruit combined data.
How to access data from DataModel object
Now to access data from list of datamodel object, simply use like shown below.
1. Fruitdata.length: Will return int value, saying size of the given list.
2. Fruitdata[index_no].name: Will return Fruitname of particular index no.
Eg: Fruitdata[0].name = ‘ Apple’.
Fruitdata[1].name = ‘Mango’.
3. Fruitdata[index_no].ImageUrl: will return Url of Image.
Let’s Build list using listview builder
ListView.builder( itemCount: Fruitdata.length, itemBuilder: (context,index){ return Card( child: ListTile( title: Text(Fruitdata[index].name), leading: SizedBox( width: 50, height: 50, child: Image.network(Fruitdata[index].ImageUrl), ), onTap: (){ Navigator.of(context).push(MaterialPageRoute(builder: (context)=>FruitDetail(fruitDataModel: Fruitdata[index],))); }, ), ); } )
Here in above snippet code, we are loading and show the item in a listview using Fruitdata object, and when a user Tap on any listview item, we are Navigating the user to FruitDetail.dart page by passing selected data from listview.
The data will be passed from page one(main.dart) to page two to constructor of page two (i.e. FruitDetail.dart)
main.dart – complete Code
import 'package:flutter/material.dart'; import 'package:passing_data/FruitDataModel.dart'; import 'package:passing_data/FruitDetail.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), debugShowCheckedModeBanner: false, ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { static List<String> fruitname =['Apple','Banana','Mango','Orange','pineapple']; static List url = ['https://www.applesfromny.com/wp-content/uploads/2020/05/Jonagold_NYAS-Apples2.png', 'https://cdn.mos.cms.futurecdn.net/42E9as7NaTaAi4A6JcuFwG-1200-80.jpg', 'https://upload.wikimedia.org/wikipedia/commons/thumb/9/90/Hapus_Mango.jpg/220px-Hapus_Mango.jpg', 'https://5.imimg.com/data5/VN/YP/MY-33296037/orange-600x600-500x500.jpg', 'https://5.imimg.com/data5/GJ/MD/MY-35442270/fresh-pineapple-500x500.jpg']; final List<FruitDataModel> Fruitdata = List.generate( fruitname.length, (index) => FruitDataModel('${fruitname[index]}', '${url[index]}','${fruitname[index]} Description...')); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar(title: Text('Pass Data from ListView to next Screen'),), body: ListView.builder( itemCount: Fruitdata.length, itemBuilder: (context,index){ return Card( child: ListTile( title: Text(Fruitdata[index].name), leading: SizedBox( width: 50, height: 50, child: Image.network(Fruitdata[index].ImageUrl), ), onTap: (){ Navigator.of(context).push(MaterialPageRoute(builder: (context)=>FruitDetail(fruitDataModel: Fruitdata[index],))); }, ), ); } ) ); } }