Hi Guys, Welcome to Proto Coders Point In this Flutter Tutorial we will look into flutter share plugin with example.
What is Flutter Share plugin?
In Flutter share plugin is very useful when user want’s to sharing contents from flutter app to any of his friends via the platform share dialog box.
This plugin is wraped with ACTION_VIEW INTENT as in android and UIActivityViewController as on iOS devices.
whenever the flutter app user wants to share any contents he can just click on share button which simply pop-up a share dialog using which he/she can easily share contents.
Let’s begin implementing Flutter share Plugin library
Flutter Share Plugin with Example
Step 1 : Add dependencies
To make user of this plugin you need to add share plugin depencencies under project pubspec.yaml file
On right side you will see your Flutter project,
your project name > pubspec.yaml
dependencies: share: ^0.6.3+5 //add this line
The Version here given may get update so please visit official site here
Step 2 : Import share.dart package
Once you have add the dependencies file, to make use of this share plugin you need to import package share.dart in any dart file where you need to use this flutter plugin.
import 'package:share/share.dart';
Step 3 : Invoke share plugin method where ever required
To invoke or show a share dialog box in Android or iOS device all you need to do is just invoke share method like this :
Share.share('check out my website https://example.com');
This share method also takes an (optional) subject property that can be used when sharing through email
Share.share('check out my website https://example.com', subject: 'Look what I made!');
Flutter Share Plugin with Complete Source Code Example
main.dart file
Just copy paste below flutter source code under main.dart file
import 'package:flutter/material.dart'; import 'package:share/share.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Flutter Share Intent"), ), body: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Center( child: Text( "Example on Share Plugin Flutter", style: TextStyle(fontWeight: FontWeight.w600, fontSize: 25.0), ), ), SizedBox( height: 25, ), Center( child: MaterialButton( elevation: 5.0, height: 50.0, minWidth: 150, color: Colors.blueAccent, textColor: Colors.white, child: Icon(Icons.share), onPressed: () { Share.share( 'check out my website https://protocoderspoint.com/'); }, ), ), SizedBox( height: 25.0, ), Center( child: Text( "Share with Subject works only while sharing on email", style: TextStyle(fontWeight: FontWeight.w600, fontSize: 15.0), ), ), Center( child: MaterialButton( elevation: 5.0, height: 50.0, minWidth: 150, color: Colors.green, textColor: Colors.white, child: Icon(Icons.share), onPressed: () { Share.share( 'check out my website https://protocoderspoint.com/', subject: 'Sharing on Email'); }, ), ), ], ), ); } }
Result :
UI Design
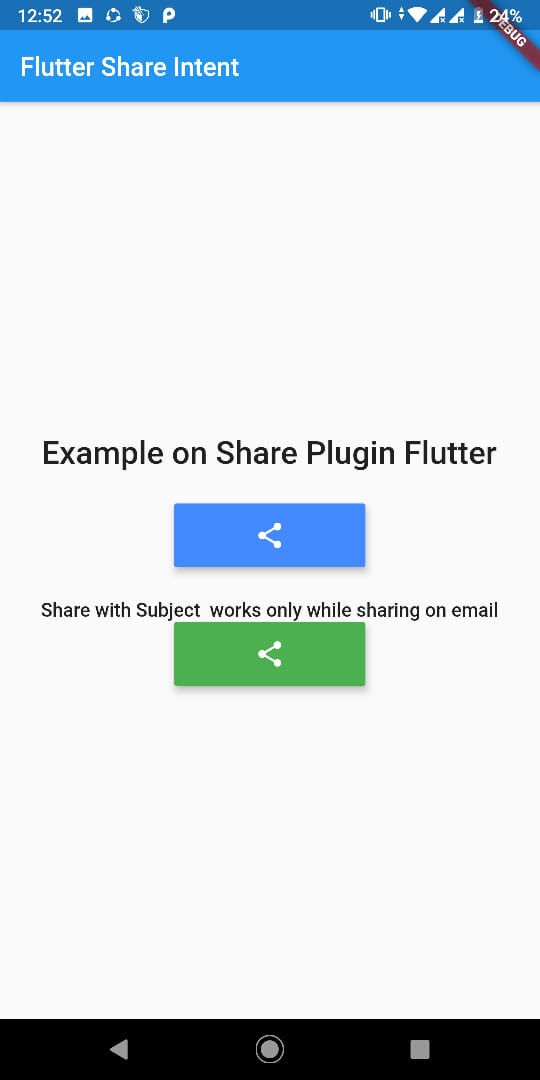
pop-up of share platform dialog box
When user clicks on blue share button and select any messenger to share contents
Then when user choice to share on Email