There might be a need during the dart/flutter app development where you need to write a function that return list of all the dates between two given list. This functionality is useful in such scenarios where you need to work on date range and display it to the user or on calender into your flutter application.
In this article let’s check out 2 ways by which we can get list of dates between given dates
1. Using DateTime() and Simply use For loop to filter data
List<DateTime> getDaysInBetween(DateTime startDate, DateTime endDate) { List<DateTime> days = []; for (int i = 0; i <= endDate.difference(startDate).inDays; i++) { days.add(startDate.add(Duration(days: i))); } return days; } // try it out void main() { DateTime startDate = DateTime(2024, 2, 1); DateTime endDate = DateTime(2024, 2, 10); List<DateTime> days = getDaysInBetween(startDate, endDate); // print the result without time days.forEach((day) { print(day.toString().split(' ')[0]); }); }
In above example, We have created a function ‘getDaysInBetween’ that accepts 2 parameter i.e. startDate & endDate. This main task of this function is getting all the date between the given date range.
This function will have a for loop that will iterate to endDate using difference method to calculate the number of days between the startDate & endDate.
Then this loop will add each date to the List of type DateTime by incrementing day by 1 using Duration.
Then finally pass two dates to the getDaysInBetween
and get the list.
but the date list you receive will also contain time in it so we need to split the time, therefore we make use of forEach loop to iterate the list and print it on screen.
Output
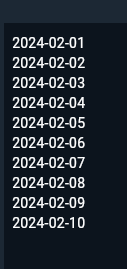
2. Using List.generate() method
List<DateTime> getDaysInBetween(DateTime startDate, DateTime endDate) { final daysToGenerate = endDate.difference(startDate).inDays + 1; return List.generate(daysToGenerate, (i) => startDate.add(Duration(days: i))); } // try it out void main() { DateTime startDate = DateTime(2024, 2, 1); DateTime endDate = DateTime(2024, 2, 10); List<DateTime> days = getDaysInBetween(startDate, endDate); // print the result without time days.forEach((day) { print(day.toString().split(' ')[0]); }); }
You can also get list of dates between any two given dates using simply using inbuilt method of dart language i.e. List.generate().