Hi Guys, Welcome to Proto coders point, In this flutter tutorial we will implement font awesome icons a package that provides more than 1500+ icons in flutter page UI design.
This font awesome icon flutter package is very useful because with few lines of code we can easily use free icons in our flutter application.
To view all the available and free icons provided by fontawesome just visit fontawesome.com & make use of the search box to discover/find out your desired icon fr the flutter app.
so lets begin with implementation of flutter awesome fonts package.
Adding icon package in your flutter app
1. Create/Open a flutter project
Offcourse you need to create/open a new flutter project in your favorite IDE, android studio or vscode.
In my case, my favorite IDE is android studio – so i will be working with it.
if flutter plugin not install in your android studio check this Install Flutter plugin & flutter sdk in android studio.
create new project IDE -> New -> New Flutter Project -> give a name to flutter project -> Next -> finish.
your flutter will be created.
2. Add font_awesome_flutter dependencies
Now once your flutter project is read and completed with the gradle build process, then now it time to add the dependencies.
then open pubspec.yaml file, that you will find in project secton on the left side of your IDE.
and app icon package dependencies
dependencies: font_awesome_flutter: ^9.0.0
3. Import it where icons are required from font awesome
then after adding the dependenies in your flutte project now to use it in your dart code you just need to import the font_awesome_flutter.dart library file.
import 'package:font_awesome_flutter/font_awesome_flutter.dart';
4. Snippet code – How to use FaIcon widget in a flutter
FaIcon ( FontAwesomeIcons.googlePay, color: Colors.deepOrange, size: 35, )
Complete Source code – font awesome icons example 1
This icon package provide more than 1500+ free icons that you can use in your flutter project.
Based on font awesome, Include all free icons:
- Regular Icons.
- Solid Icons.
- Brand icons.
Source code
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'package:font_awesome_flutter/font_awesome_flutter.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( centerTitle: true, title: Text("Font Awesome Icons") ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text("Few Free Icon from Font Awesome Flutter package",textAlign:TextAlign.center,style: TextStyle(fontSize: 30),), SizedBox(height: 20,), //brand Icons Text("Brand Icons",textAlign: TextAlign.start,style: TextStyle(fontWeight: FontWeight.bold,fontStyle:FontStyle.italic,fontSize: 20),), Row( mainAxisAlignment: MainAxisAlignment.center, children: [ FaIcon(FontAwesomeIcons.googlePay,color: Colors.deepOrange,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.facebook,color: Colors.blue,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.github,color: Colors.black,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.twitter,color: Colors.blueAccent,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.aws,color: Colors.deepOrange,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.pinterest,color: Colors.red,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.wordpress,color: Colors.deepPurple,size: 35,), ], ), SizedBox(height: 25,), //Regular Icons Text("Regular Icons",textAlign: TextAlign.start,style: TextStyle(fontWeight: FontWeight.bold,fontStyle:FontStyle.italic,fontSize: 20),), Row( mainAxisAlignment: MainAxisAlignment.center, children: [ FaIcon(FontAwesomeIcons.smile,color: Colors.green,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.laugh,color: Colors.blue,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.dollarSign,color: Colors.green,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.accessibleIcon,color: Colors.red,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.iceCream,color: Colors.pink,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.plane,color: Colors.brown,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.peopleArrows,color: Colors.deepPurple,size: 35,), ], ), SizedBox(height: 25,), //Regular Icons Text("Solid Icons",textAlign: TextAlign.start,style: TextStyle(fontWeight: FontWeight.bold,fontStyle:FontStyle.italic,fontSize: 20),), Row( mainAxisAlignment: MainAxisAlignment.center, children: [ FaIcon(FontAwesomeIcons.virus,color: Colors.red,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.mask,color: Colors.blue,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.dollarSign,color: Colors.green,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.atom,color: Colors.red,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.truckMonster,color: Colors.black,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.bacteria,color: Colors.red,size: 35,), SizedBox(width: 15,), FaIcon(FontAwesomeIcons.arrowAltCircleRight ,color: Colors.deepPurple,size: 35,), ], ), ], ), ), ); } }
Font awesome icon output
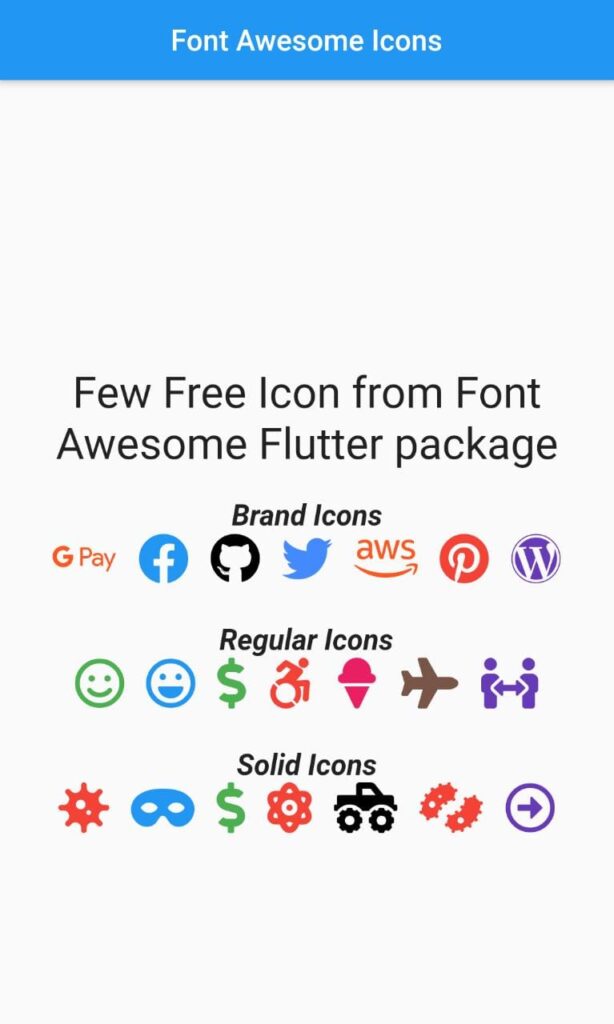
This flutter icons package provide lots of regular & also many brand icons that you are allowed to use freely, such as:
- google play icon,
- Amazon AWS icon,
- facebook icons
and much more like regular icons such as:
- home Icon,
- setting icon
- back arrow icon,
- forward arrow icon
- menu icon & much more using this package.
Bottom Navigation Bar – Font Awesome Icons Example 2
code
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'package:font_awesome_flutter/font_awesome_flutter.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( centerTitle: true, title: Text("Font Awesome Icons") ), body: Container(), bottomNavigationBar: BottomNavigationBar( items: [ BottomNavigationBarItem(icon: FaIcon(FontAwesomeIcons.home),label: "Home"), BottomNavigationBarItem(icon: FaIcon(FontAwesomeIcons.idBadge),label: "Profile"), BottomNavigationBarItem(icon: FaIcon(FontAwesomeIcons.cog),label: "Setting"), ], ) , ); } }
Output
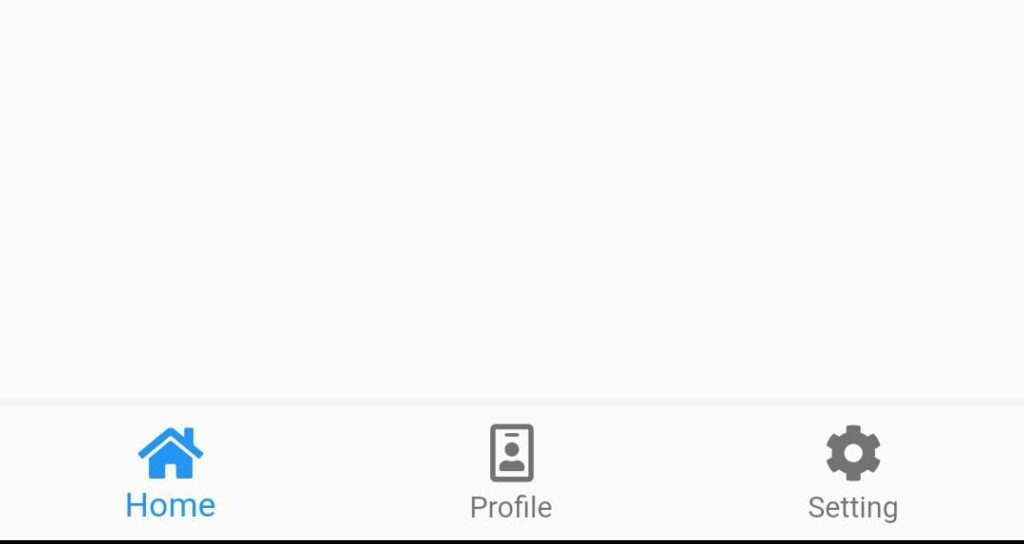