Hi Guys, Welcome to Proto Coders Point. In flutter 2.5 release, will learn how to make use of flutter Material Banner to show snackbar Flutter Material Banner to app user.
Material you – Material Banner in flutter 2.5 release notes
In Flutter 2.0 release version, they have announced a feature that show a snackbar at the bottom of app screen to notify user about any quick guide information,
ScaffordMessenger Snackbar used to work something that auto dismissable after few seconds, Therefore Snackbar is been used mostly to show a quick message on screen.
So, Now with Flutter 2.5.0 upgrade you can add a Material Banner at top of a screen that will be visible to user until user dismiss it.
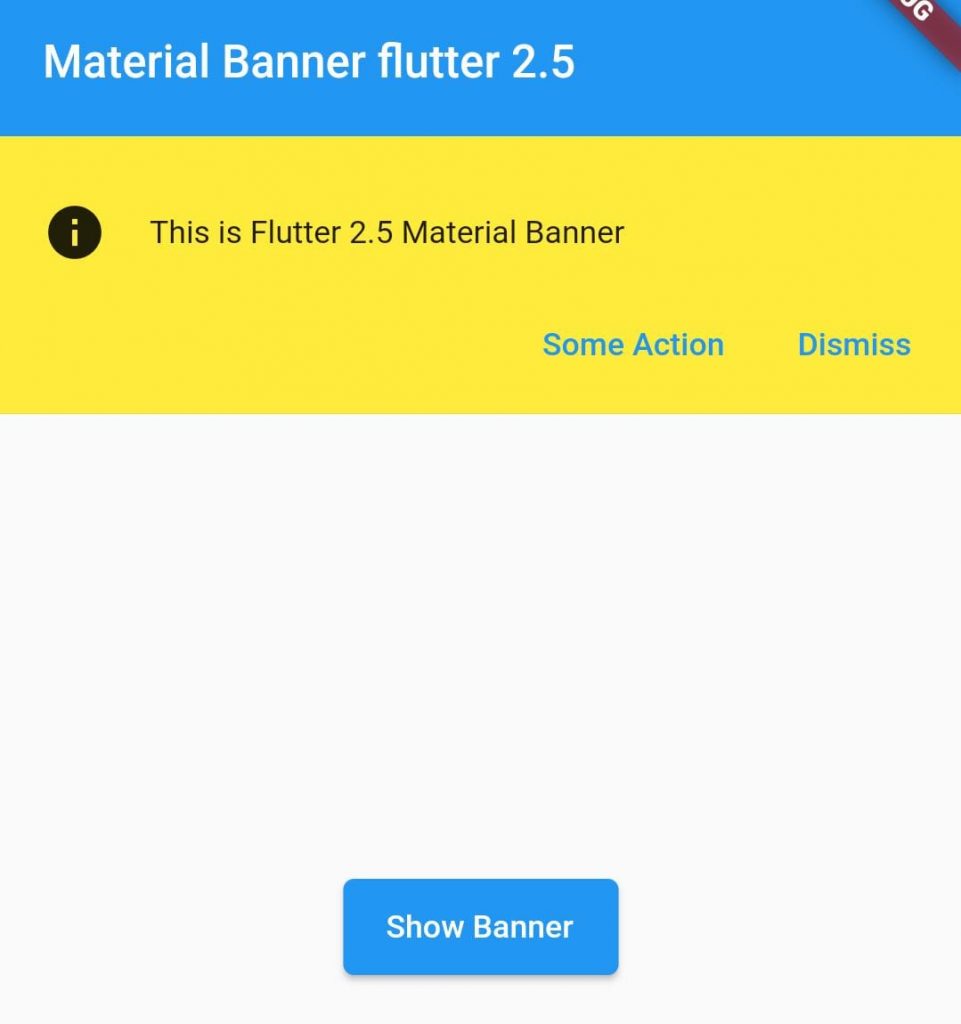
Video Tutorial
Flutter Upgrade – How to update flutter version
To update your flutter SDK version to 2.5 you just need to run a command on a Command Prompt or IDE Terminal.
flutter upgrade

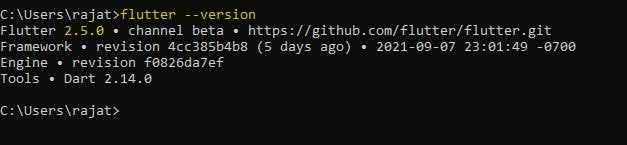
Now, you can use feature of Flutter 2.5.0
Flutter Material Banner Properties
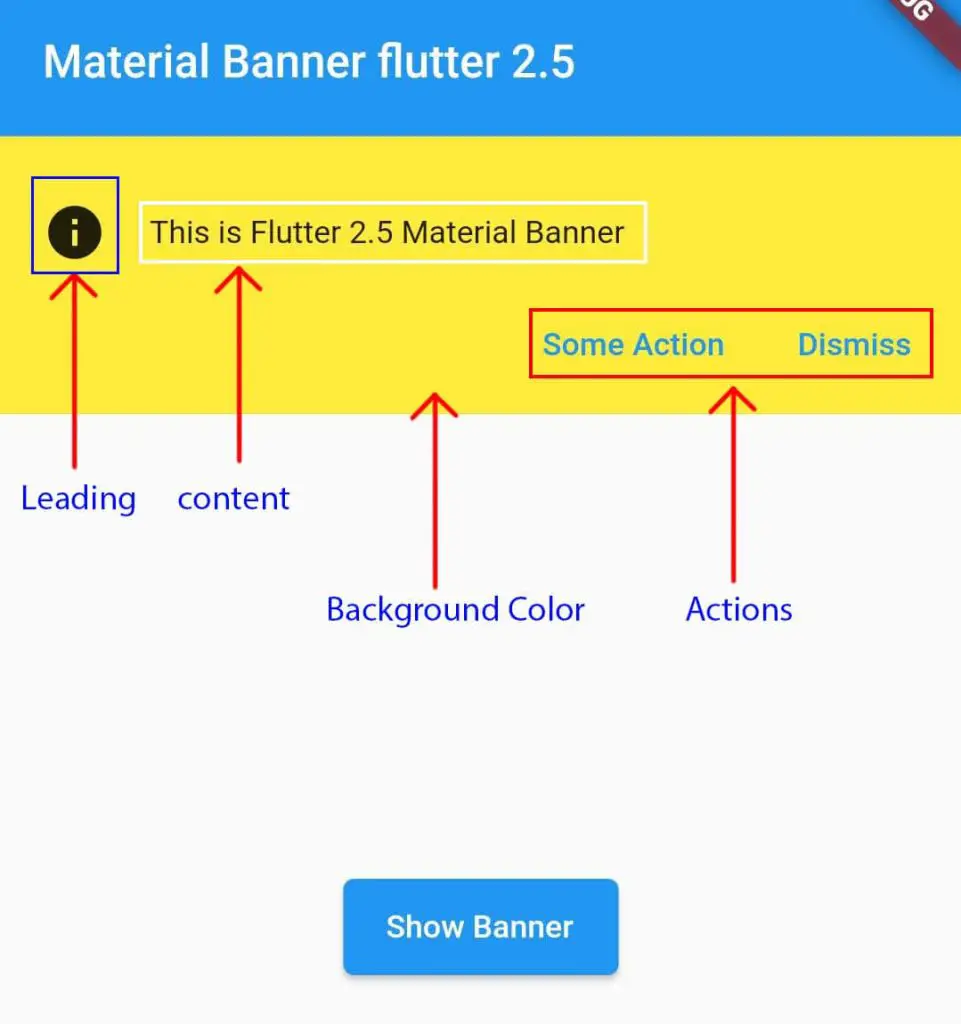
Snippet code
ScaffoldMessenger.of(context).showMaterialBanner( MaterialBanner( content: const Text('This is Flutter 2.5 Material Banner'), leading: const Icon(Icons.info), backgroundColor: Colors.yellow, actions: [ TextButton(onPressed: (){}, child: const Text('Some Action')), TextButton(onPressed: (){ ScaffoldMessenger.of(context).hideCurrentMaterialBanner(); }, child: const Text('Dismiss')), ], ) );
Properties to customize Material Banner in flutter
properties | usage |
content: | Text Message to be shown to user’s |
leading: | show a image logo or Icons about the banner information eg: info, warning, success…etc |
backgroundColor: | Gives color to Material Banner. |
actions:[] | (mandatory) It accept array of widget when user can interact to perform some event. |
The Flutter Material Banner according to it’s guidelines, The state of Banner should show only once to the user. If showMaterialBanner is called more then one time, then ScaffordMessenger will maintain a queue for next banner to be shown.
Therefore when previous banner(current active banner) is dismissed then new banner that is in queue will be shown.
removeCurrentMaterialBanner()
As you read above Scafford Messenger showMaterialBanner, maintain a queue of banner, if show MaterialBanner is called more then once, therefore we need to remove the current Material Banner which is active before invoking new Banner.
To do that we just need to call ..removeCurrentMaterialBanner() before invoking new Material Banner in flutter.
ScaffoldMessenger.of(context)..removeCurrentMaterialBanner()..showMaterialBanner( MaterialBanner( content: const Text('This is Flutter 2.5 Material Banner'), leading: const Icon(Icons.info), backgroundColor: Colors.yellow, actions: [ TextButton(onPressed: (){}, child: const Text('Some Action')), TextButton(onPressed: (){ ScaffoldMessenger.of(context).hideCurrentMaterialBanner(); }, child: const Text('Dismiss')), ], ) );
Complete Code – Flutter dart 2.5.0 to show Material Banner (Snackbar) on top
main.dart
Here we have a button ‘Show Banner’ then pressed will call a method that will invoke material banner in flutter.
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text('Material Banner flutter 2.5'), ), body: Center( child: ElevatedButton( child: const Text('Show Banner'), onPressed: (){ showMaterialBanner(); }, ), ) ); } //invoke and display a banner in flutter app showMaterialBanner(){ ScaffoldMessenger.of(context)..removeCurrentMaterialBanner()..showMaterialBanner( MaterialBanner( content: const Text('This is Flutter 2.5 Material Banner'), leading: const Icon(Icons.info), backgroundColor: Colors.yellow, actions: [ TextButton(onPressed: (){}, child: const Text('Some Action')), TextButton(onPressed: (){ ScaffoldMessenger.of(context).hideCurrentMaterialBanner(); }, child: const Text('Dismiss')), ], ) ); } }