Hi Guys, Welcome to Proto Coders Point, In this android tutorial we gonna integrate an app for OTP Verification in android.
For this, we gonna make use of the TextLocal SMS Service platform. I have already talked about TextLocal an India’s No 1 SMS service provider
Check out this Post Send SMS using text local an SMS GATEWAY – Android Studio Application
Let’s begin Integrating OTP Verification in our android application.
Mobile Number OTP Verification / SMS OTP verification in Android
Step 1: Creating a new account in textlocal.in or log in
go to textlocal.in create a new account or login with an old account if you have signup below with TextLocal.
After a Successful log In you will see a dashboard something like below
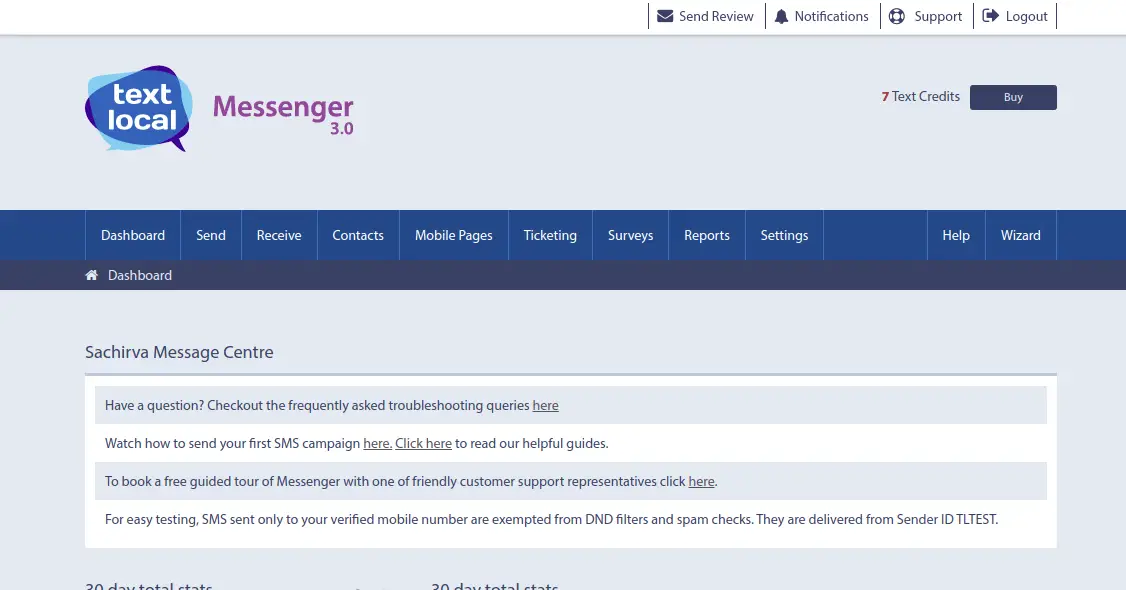
TextLocal provides you Free SMS Credit of 10, using which you can send up to 10 free SMS After you use all the Free SMS Credits you may need to Buy their services for more SMS benefits. For App testing you can user textlocal otp generator.
Step 2: Creating a new API KEY in Text Local to send OTP
On your text local dashboard go to settings > API KEY.
Then Create a new API KEY
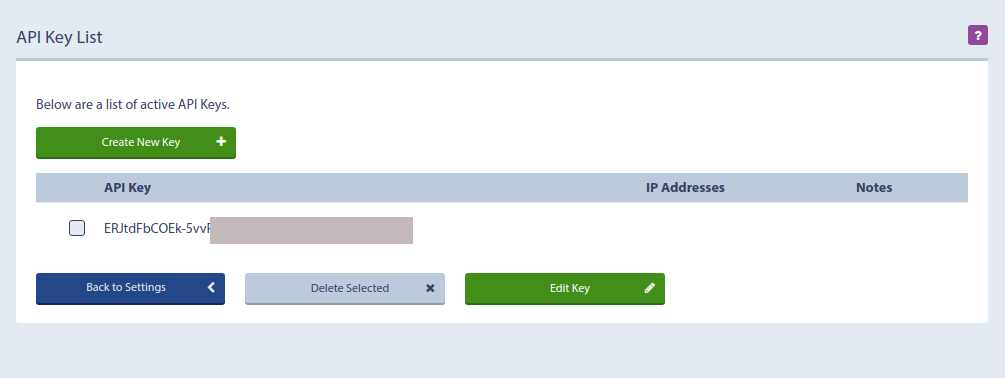
Note down the API key, because we then in our android application to send OTP to a mobile number via Text local.
Step 3: Creating a new Android Project
Now open Android Studio as usual and create a new android application and name it as Android OTP Verification in android or anything your wish.
Step 4: Creating an OTP verification UI in android
step1: create an XML file
under res > drawable > creating an XML file and name it as:
edittext_stroke_borders.xml
<shape xmlns:android="http://schemas.android.com/apk/res/android"> <corners android:radius="10dp" /> <stroke android:width="1dp" android:color="@color/colorPrimary" /> </shape>
this above XML code will create a transparent border that we can use to show in EditText view as the background.
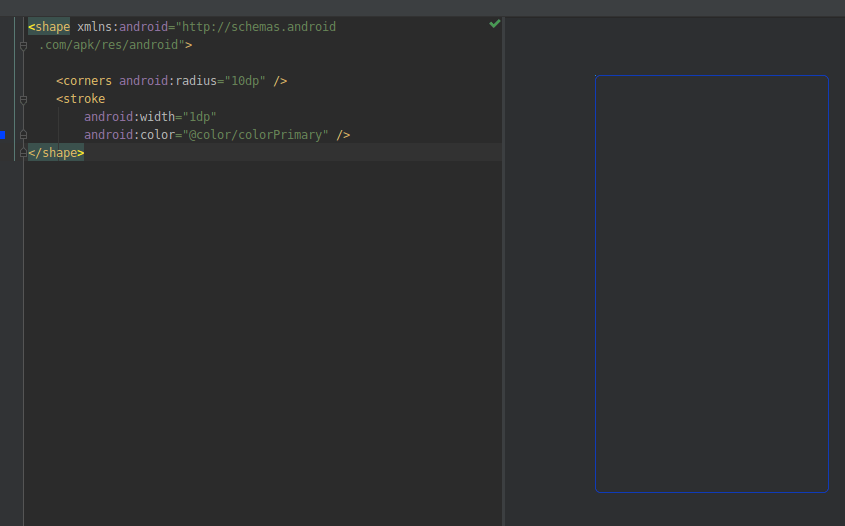
Step2: login page UI design
main activity.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" android:gravity="center" android:orientation="vertical"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:gravity="center" android:text="Welcome to Proto Coders Point" android:textColor="@android:color/black" android:textSize="20sp" android:layout_gravity="center"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:gravity="center" android:text="Android Tutorial on OTP VERIFICATION" android:textColor="@android:color/black" android:textSize="20sp" android:layout_gravity="center"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Login " android:textColor="@android:color/black" android:textSize="30sp" android:layout_gravity="center"/> <EditText android:id="@+id/userphoneno" android:layout_width="match_parent" android:layout_height="50dp" android:ems="10" android:layout_margin="10dp" android:padding="5dp" android:background="@drawable/edittext_stoke_borders" android:inputType="number" android:hint="Enter Your Phone" tools:layout_editor_absoluteX="118dp" tools:layout_editor_absoluteY="76dp" /> <Button android:id="@+id/getotp" android:layout_width="wrap_content" android:layout_height="wrap_content" android:gravity="center" android:textColor="@android:color/black" android:text="SignIN" android:layout_marginTop="10dp" android:layout_gravity="center" android:background="@color/colorAccent"/> </LinearLayout>
Step 3: create a new activity
you need a new activity where we are going verify the OTP, for that you need to create an Activity with an XML file
right-click on java > new > Activity > Empty Activity
Name it as OTP_Verification_Page
Then open the XML file of OTP Verification page and copy-paste below lines of XML UI code
activity_verify_otp.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".Activity.GetOPT" android:orientation="vertical" android:layout_gravity="center" android:gravity="center"> <Button android:id="@+id/get_otp_no" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_gravity="center" android:layout_margin="20dp" android:layout_marginTop="10dp" android:background="@color/colorPrimary" android:gravity="center" android:onClick="GetOTPNO" android:text="Get OTP Now" android:textColor="@android:color/white" /> <EditText android:id="@+id/otpedittext" android:layout_width="match_parent" android:layout_height="50dp" android:ems="10" android:layout_margin="10dp" android:padding="5dp" android:background="@drawable/edittext_stoke_borders" android:inputType="number" android:hint="......" android:letterSpacing="0.5" android:maxLength="6" android:gravity="center" tools:layout_editor_absoluteX="118dp" tools:layout_editor_absoluteY="76dp" /> <Button android:id="@+id/verifyotp" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_gravity="center" android:layout_margin="20dp" android:layout_marginTop="10dp" android:background="@color/colorAccent" android:gravity="center" android:onClick="verifyOTP" android:text="Verify" android:textColor="@android:color/black" /> </LinearLayout>
Step 4: create another Activity
like the same process as above create another Activity,
Here we gonna navigate the user if the OTP Verification mobile number was successful.
activity_successpage.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".SuccessLoginIn" android:gravity="center"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="25sp" android:textColor="@android:color/black" android:text="OTP VERIFIED SUCCESSFULLY"/> </LinearLayout>
Now we are done with OTP Verification UI android, Now let’s add some backend code in MainActivity.java, VerifyOTP.java, SuccessLogin.java.
Step 5: Adding Backend Java code
MainActivity.java
package com.ui.otpverfication; import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText; public class MainActivity extends AppCompatActivity { Button getotp; EditText userphoneno; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); getotp=(Button)findViewById(R.id.getotp); userphoneno=(EditText)findViewById(R.id.userphoneno); getotp.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if(userphoneno.getText().toString().trim().isEmpty()) { userphoneno.setError("Enter Phone No"); }else{ Intent i = new Intent(MainActivity.this, VerifyOTP.class); i.putExtra("phone",userphoneno.getText().toString().trim()); startActivity(i); finish(); } } }); } }
Here we are simply passing mobile number entered by users via intent to VerifyOTP.java page.
VerifyOTP.java
package com.ui.otpverfication; import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; import android.os.StrictMode; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.Toast; import java.io.BufferedReader; import java.io.InputStreamReader; import java.net.HttpURLConnection; import java.net.URL; import java.util.Random; public class VerifyOTP extends AppCompatActivity { int randonnumber; String phonenumber; Button verifyotp,getVerifyotp; EditText otp_edittext; String otp_text; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_verify_otp); verifyotp=(Button)findViewById(R.id.verifyotp); getVerifyotp=(Button)findViewById(R.id.get_otp_no); otp_edittext=(EditText)findViewById(R.id.otpedittext); final StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build(); StrictMode.setThreadPolicy(policy); Intent intent=getIntent(); phonenumber=intent.getStringExtra("phone"); Toast.makeText(VerifyOTP.this, ""+phonenumber, Toast.LENGTH_SHORT).show(); } void initialsendotp(){ try { // Construct data String apiKey = "apikey=" + "ERJtdFbCOEk-5vvFn7mjg4SuywzHCcQkNxxxx(Replace with your API KEY)"; Random random = new Random(); randonnumber=random.nextInt(99999); String message = "&message=" + "Hey, Your OTP is " +randonnumber; String sender = "&sender=" + "TXTLCL"; String numbers = "&numbers=" +phonenumber; // Send data HttpURLConnection conn = (HttpURLConnection) new URL("https://api.textlocal.in/send/?").openConnection(); String data = apiKey + numbers + message + sender; conn.setDoOutput(true); conn.setRequestMethod("POST"); conn.setRequestProperty("Content-Length", Integer.toString(data.length())); conn.getOutputStream().write(data.getBytes("UTF-8")); final BufferedReader rd = new BufferedReader(new InputStreamReader(conn.getInputStream())); final StringBuffer stringBuffer = new StringBuffer(); String line; while ((line = rd.readLine()) != null) { stringBuffer.append(line); } rd.close(); } catch (Exception e) { System.out.println("Error SMS "+e); } } public void GetOTPNO(View view) { initialsendotp(); Toast.makeText(VerifyOTP.this,"OTP SENT< Please Wait you may recieved in a movement",Toast.LENGTH_LONG).show(); getVerifyotp.setVisibility(View.GONE); } public void verifyOTP(View view) { Toast.makeText(VerifyOTP.this,"Verify Button",Toast.LENGTH_LONG).show(); otp_text = otp_edittext.getText().toString().trim(); if(otp_text.equals(String.valueOf(randonnumber))) { Toast.makeText(VerifyOTP.this,"user login in successfully",Toast.LENGTH_LONG).show(); finish(); Intent mainactivity = new Intent(VerifyOTP.this,SuccessLoginIn.class); startActivity(mainactivity); } else{ Toast.makeText(VerifyOTP.this,"Invalid OTP, Please Try Again",Toast.LENGTH_LONG).show(); } } }
Here we have 2 buttons, one will send OTP to the registered mobile number and another button will simply verify the OTP entered by the user. and if the OTP entered by the user is correct the user will be navigated to the success page.
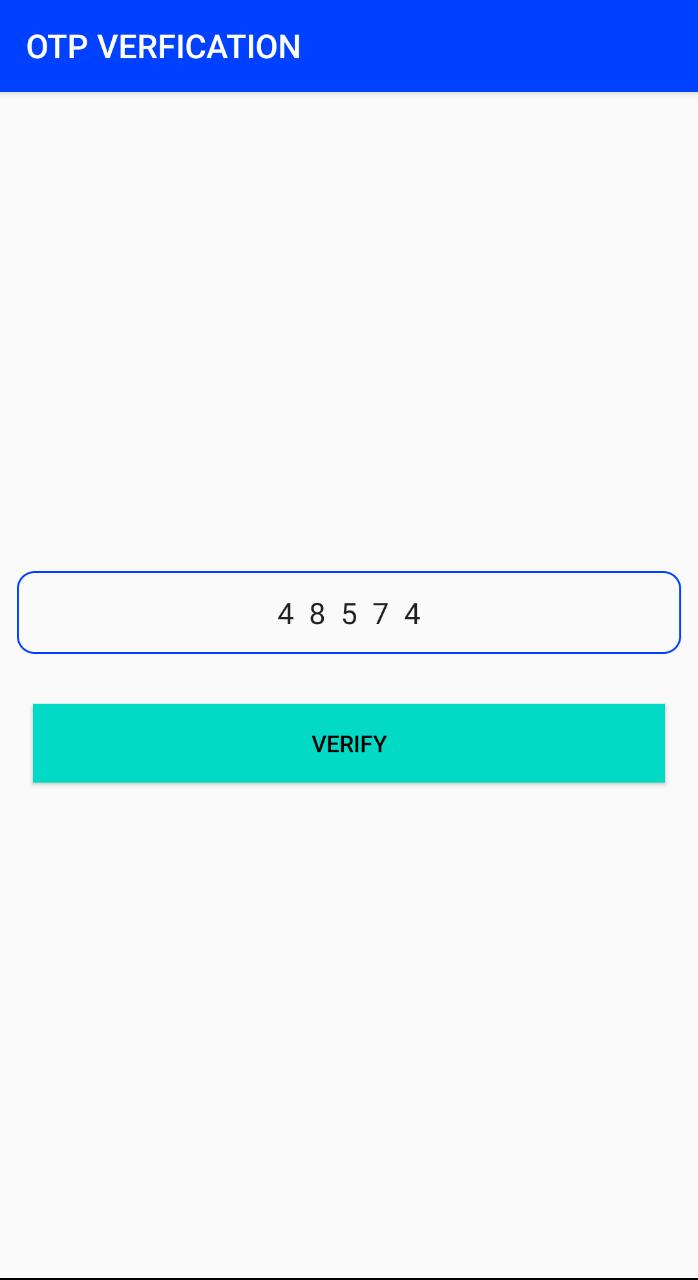
SuccessPage.java
package com.ui.otpverfication; import androidx.appcompat.app.AppCompatActivity; import android.os.Bundle; public class SuccessLoginIn extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_success_login_in); } }
here we are simply setting the content view with the layout XML file.
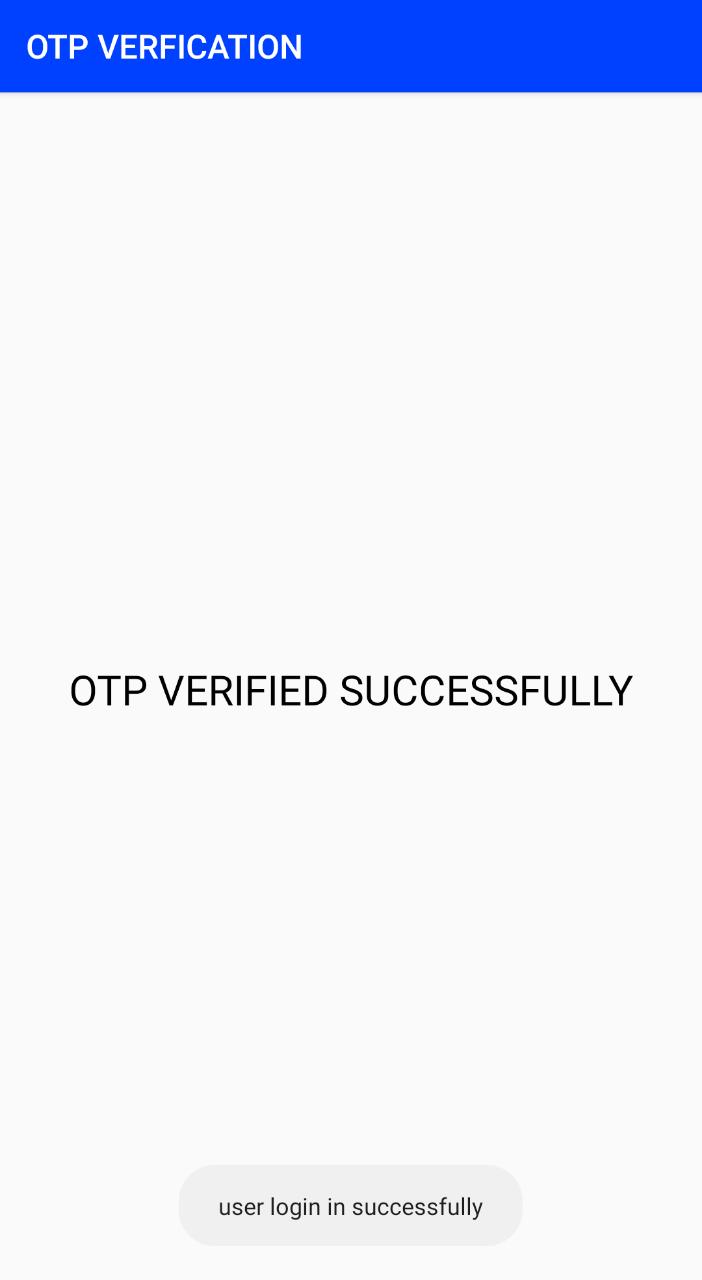
After all, Don’t forget to set <user-permission> INTERNET under AndroidManifest.xml
AndroidManifest.xml
<uses-permission android:name="android.permission.INTERNET"/> // need to make network calls
Recommended Android Articles
Reading incoming message automatically in android to verify otp