Hi Guys, Welcome to Proto Coders Point, In this flutter tutorial we will learn how to play audio file by using assets_audio_player package that help you to play music from your project assets folder.
Video Tutorial
About Assets audio player package
This audio player flutter package is a very easy-to-use package that helps developers implement sound effects or play the audio files (mp3) from the assets folder, Can be used to develop apps that need some sound effects at a particular time (Eg: Game App).
Now with the latest update with the package, A new feature is been added i.e. we can play audio files from the network url or your own audio API & also listen to radio/Livestream audio music.
And if audio/music is playing & you exit the app, still the audio will be in background & show you Notification in notification bar you can also control the music that is playing from notification itself.
Feature of assets_audio_player package
You can play audio in various ways like
Assets File: Store audio in assets folder & play the audio by its path.
Network url: use exact url of audio file to play it.
Network livestream/radio url: use livestream url to play audio.
Various command/method to play with audio/sounds
- play()
- pause()
- stop()
- seek(Duration to)
- seekBy(Duration by)
- forword(speed)
- rewind(speed)
- next()
- previous().
There are more awesome feature that you can try ” to learn more about the assets audio player visit official site here“
How to add & use assets audio player package in flutter
1. Add Dependencies
Open your flutter project & navigate to pubspec.yaml file & open it, then under dependencies add the audio player package.
dependencies: flutter: sdk: flutter cupertino_icons: ^1.0.0 assets_audio_player: ^3.0.3+3 #add this
then don’t forget to hit pub get button, it will download the package in your flutter project.
2. Add audio/music file in a folder
Create new directory in your flutter project Eg: assets/sound/ and copy/paste a music audio file in it. Check out below screenshot.
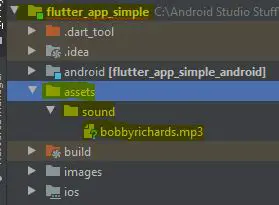
3. Declare the path of audio assets directory in pubspec.yaml
We need to specily the path of the audio directory, so that the app can read the files from the directory.
flutter: # To add assets to your application, add an assets section, like this: assets: - assets/sound/
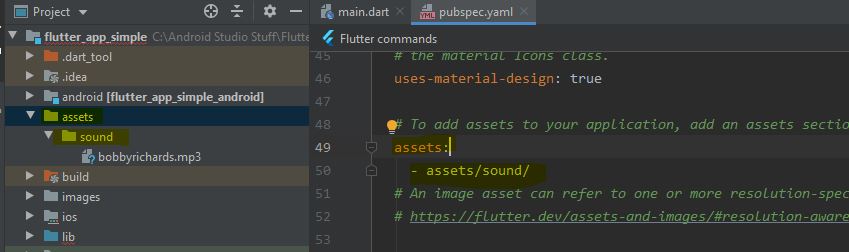
Snippet Code of Assets Audio Player Package
Importing the package
You need to import the audio player package in dart file, where you want to use it.
import 'package:assets_audio_player/assets_audio_player.dart';
Creating an instance of AssetAudioPlayer
AssetsAudioPlayer audioPlayer = AssetsAudioPlayer(); // this will create a instance object of a class
Now you can use audioPlayer object to set path of your audio file as shown below.
Open/initialize the audio file path
The below method create and initialize the audio file and play it automatically by default if you do not set autoStart: false.
audioplayer.open( Audio('assets/sound/bobbyrichards.mp3') );
if you don’t want autoplay of audio then you can set property autoStart: false,
& There is one more useful property called showNotification:true.
audioplayer.open( Audio('assets/sound/bobbyrichards.mp3'), autoStart: false, showNotification: true );
This above snippet code will play sound only when play() method get triggered when button is been pressed. and display a notification.
Play audio from network or from your Audio API
void playAudioNetwork() async{ try{ await player.open( Audio.network("URL PATH") ); }catch(t){ } }
Method used to control the audio player
play(): play the audio file.
pause(): pause the audio file.
stop(): completly stop the audio, then if played again then song start from beginning.
playOrPause(): if audio playing, then pause else play.
next(): play next song from the list of songs.
previous(): play prev song from the list of songs.
Jump to specific audio time length
if you want to play the music a particular time file or if you want to forword or rewind the audio at particular time in audio file.
audioPlayer.seek(Duration(seconds: 10)); // just to audio time 10
Forword or rewind the audio by specific time duration
audioPlayer.seekBy(Duration(seconds: 10)); // go 10 sec forword audioPlayer.seekBy(Duration(seconds: -10)); /go -10 sec backwork
Learn more from offical site here.
Complete Source code Example on Assets Audio Player package
Output
Check the output on my youtube channel, Video tutorial is above
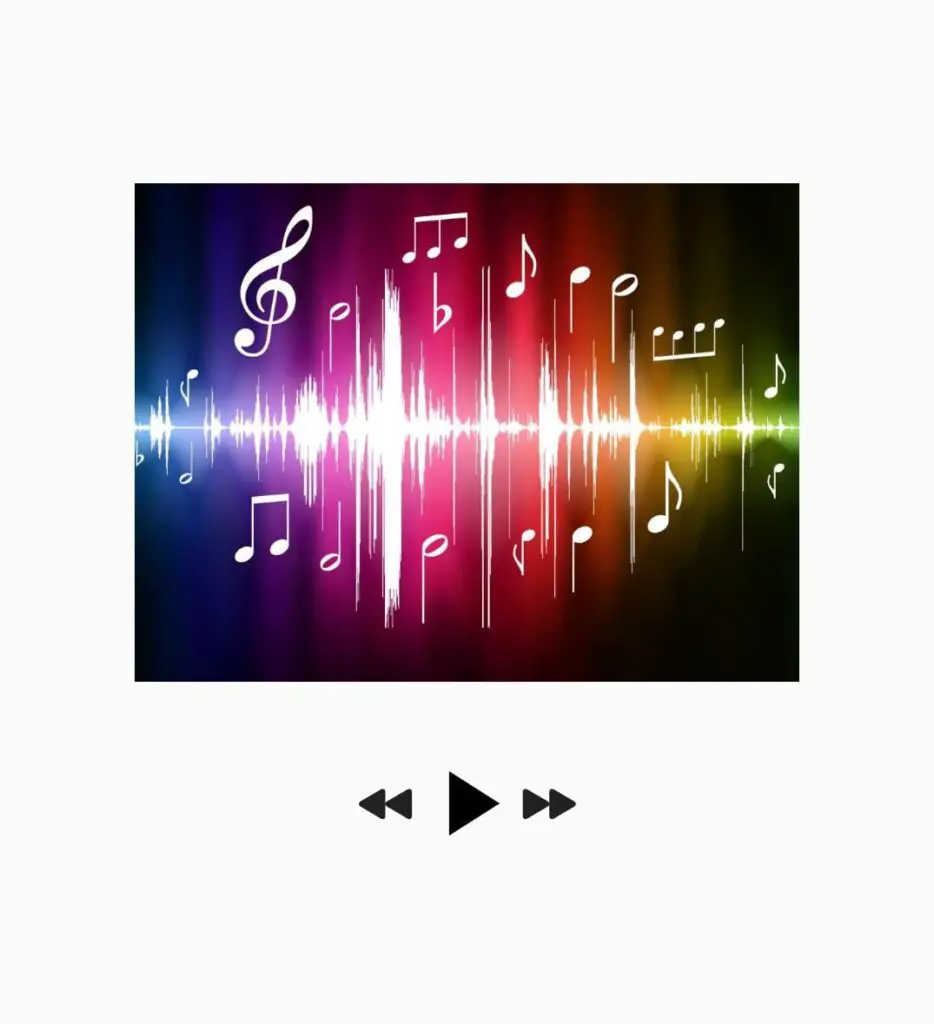
main.dart
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'package:assets_audio_player/assets_audio_player.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> with SingleTickerProviderStateMixin { late AnimationController iconController; // make sure u have flutter sdk > 2.12.0 (null safety) bool isAnimated = false; bool showPlay = true; bool shopPause = false; AssetsAudioPlayer audioPlayer = AssetsAudioPlayer(); @override void initState() { // TODO: implement initState super.initState(); iconController = AnimationController( vsync: this, duration: Duration(milliseconds: 1000)); audioPlayer.open(Audio('assets/sound/bobbyrichards.mp3'),autoStart: false,showNotification: true); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( centerTitle: true, title: Text("Playing Audio File Flutter"), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Image.network("https://i.pinimg.com/originals/f7/3a/5b/f73a5b4b7262440684a2b5c39e684304.jpg",width: 300,), SizedBox(height: 30,), Row( mainAxisAlignment: MainAxisAlignment.center, children: [ InkWell(child: Icon(CupertinoIcons.backward_fill),onTap: (){ audioPlayer.seekBy(Duration(seconds: -10)); },), GestureDetector( onTap: () { AnimateIcon(); }, child: AnimatedIcon( icon: AnimatedIcons.play_pause, progress: iconController, size: 50, color: Colors.black, ), ), InkWell(child: Icon(CupertinoIcons.forward_fill),onTap: (){ audioPlayer.seekBy(Duration(seconds: 10)); audioPlayer.seek(Duration(seconds: 10)); audioPlayer.next(); },), ], ), ], ), )); } void AnimateIcon() { setState(() { isAnimated = !isAnimated; if(isAnimated) { iconController.forward(); audioPlayer.play(); }else{ iconController.reverse(); audioPlayer.pause(); } }); } @override void dispose() { // TODO: implement dispose iconController.dispose(); audioPlayer.dispose(); super.dispose(); } }