Hi Guys, Welcome to Proto Coders Point, In this Nodejs project for beginner, We will learn how to build RESTAPI in nodejs using ExpressJS & MongoDB as NoSQL database.
NodeJS RESTAPI Example – Book Directory Project
In this NodeJS tutorial, We will develop backend RESTAPI a “Book directory project”, where we will perform CRUD operation like Create, Read, Update & Delete data from MongoDB Database.
Let’s get started with developing book list api using nodejs
1. Check for NODEJS & MONGODB Installed
I assume that you have installed the required environment(i.e. nodejs & mongoDB).
To check if nodejs & mongodb is installed or no, simply run npm -v
& mongo --version
in your command prompt & thus if installed it will show the version that is been installed.
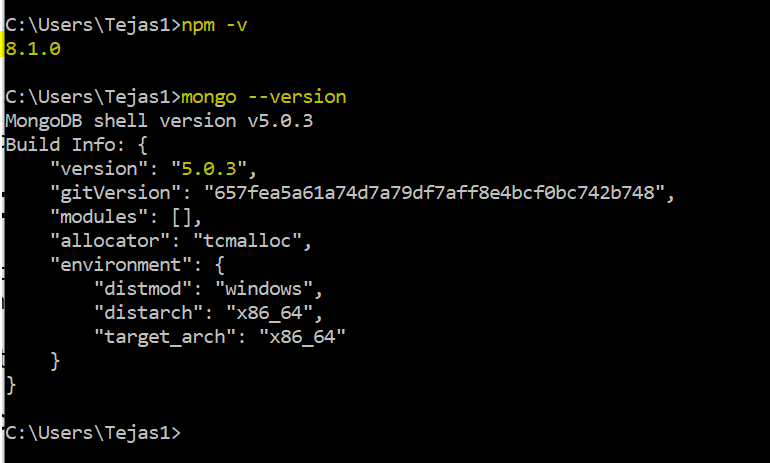
2. Create nodejs project folder & Create package.json file
open command prompt
- Navigate to drive or directory where you want to create nodejs project
- Create folder (book_dir_api) –
mkdir book_dir_api
- Navigate to newly created folder –
cd book_dir_api
- create package.json file –
npm init
- open nodejs project in vscode editor –
code .
npm init will create a package.json file & will ask you to enter some details about your nodejs project. if you don’t want to fill any details information then simply run npm init -y
3. Install required nodejs module
We need 4 nodejs packages/modules
- Express: For creating server & routes, basically express is used as middleware.
- body-Parser: To be able to read & display response in json form.
- mongoose: To store data in mongodb and get and update the data(Basicallly for CRUD operation).
- nodemon: To auto restart nodejs server, when file changes is made.
Install them all at once using below command
npm i express body-parser mongoose nodemon
After installing all the above nodejs modules, your package.json will look like this,
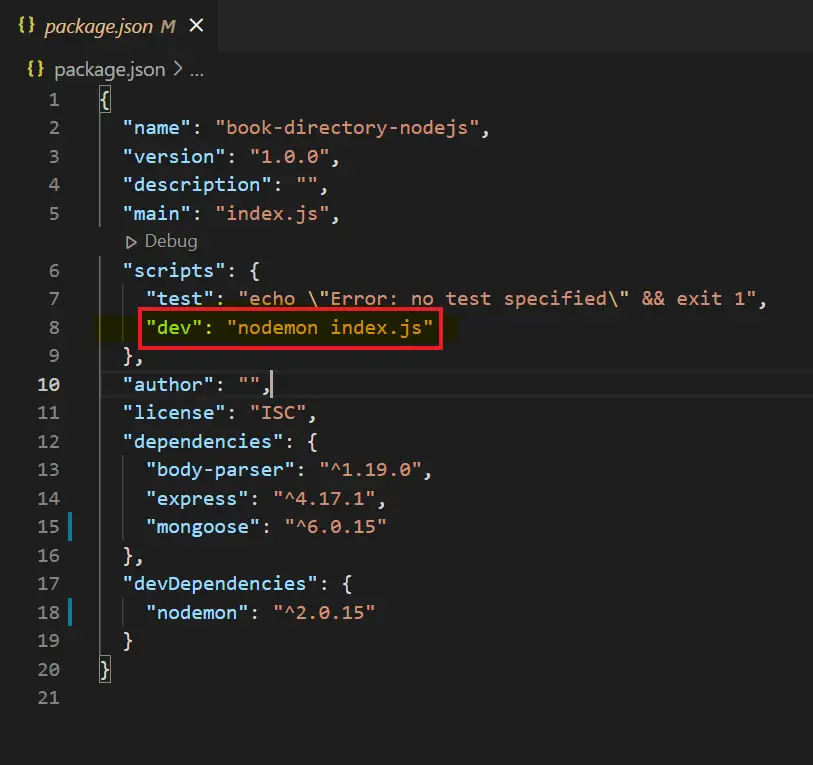
check the highlight in above screenshot
"scripts": { "dev": "nodemon index.js" // }
index.js is the file that start/restarts every time there is some change in script.
4. Coding for nodejs book directory api mini project
My NodeJS Structure
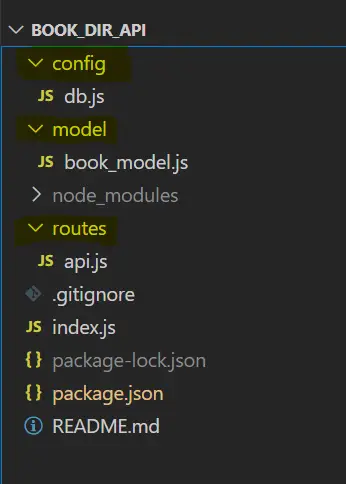
Create 3 folders & respective js file as in above screenshot
1. config > db.js : Handles MongoDB connection using mongoose.
2. model > book_model.js : Will have mongodb mongoose schema(data structure).
3. routes > api.js : Will have all the routes to perform CRUD operation.
It will handle routes such as:-
4. index.js : Putting all together, main file.
- get: Get all books list of data from DB.
- post: Insert new Book records into Database.
- put: used to perform update operation.
- delete: delete book record by using book id.
Codes
config -> db.js
const mongoose = require('mongoose'); var url ='mongodb://localhost:27017/booksDB'; const connection = mongoose.createConnection(url); module.exports = connection;
model -> book_model.js
const mongoose = require('mongoose'); const db = require('../config/db'); const bookSchema = new mongoose.Schema({ title:{ type:String, default:"----" }, isbn:{ type:Number, }, author:{ type:String, default:"----" } }); const bookmodel = db.model('books',bookSchema); module.exports = bookmodel;
routes -> api.js
const router = require('express').Router(); const bookModel = require('../model/book_model'); router.get('/books', async function (req, res) { const bookList = await bookModel.find(); console.log(bookList); res.send(bookList); }); router.get('/books/:id', async function (req, res) { const { id } = req.params; const book = await bookModel.findOne({isbn : id}); if(!book) return res.send("Book Not Found"); res.send(book); }); router.post('/books', async function (req, res) { const title= req.body.title; const isbn = req.body.isbn; const author = req.body.author; const bookExist = await bookModel.findOne({isbn : isbn}); if (bookExist) return res.send('Book already exist'); var data = await bookModel.create({title,isbn,author}); data.save(); res.send("Book Uploaded"); }); router.put('/books/:id', async function (req, res) { const { id } = req.params; const { title, authors, } = req.body; const bookExist = await bookModel.findOne({isbn : id}); if (!bookExist) return res.send('Book Do Not exist'); const updateField = (val, prev) => !val ? prev : val; const updatedBook = { ...bookExist , title: updateField(title, bookExist.title), authors: updateField(authors, bookExist.authors), }; await bookModel.updateOne({isbn: id},{$set :{title : updatedBook.title, author: updatedBook.authors}}) res.status(200).send("Book Updated"); }); router.delete('/books/:id', async function (req, res) { const { id } = req.params; const bookExist = await bookModel.findOne({isbn : id}); if (!bookExist) return res.send('Book Do Not exist'); await bookModel.deleteOne({ isbn: id }).then(function(){ console.log("Data deleted"); // Success res.send("Book Record Deleted Successfully") }).catch(function(error){ console.log(error); // Failure }); }); module.exports = router;
index.js
In index.js, will bring all files together using express as middleware.
const express = require('express'); const bodyParser = require('body-parser'); const api = require('./routes/api'); const app = express(); const PORT = 5000; app.use(bodyParser.json()); app.use('/', api); app.listen(PORT, () => console.log(`App listening on port ${PORT}`));
5. Run the nodejs server index.js
npm run dev
thus your script will start running on localhost post 5000
http://localhost:5000/
Testing API in POSTMAN
1. Store Book Data in DB using POST method
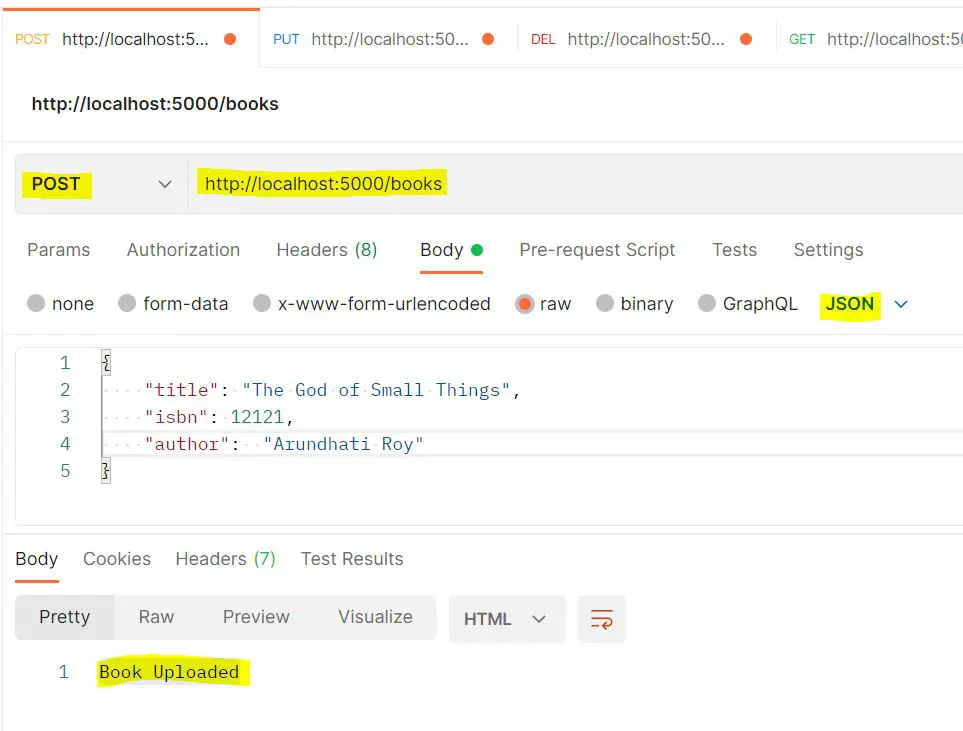
2. get book data using book id
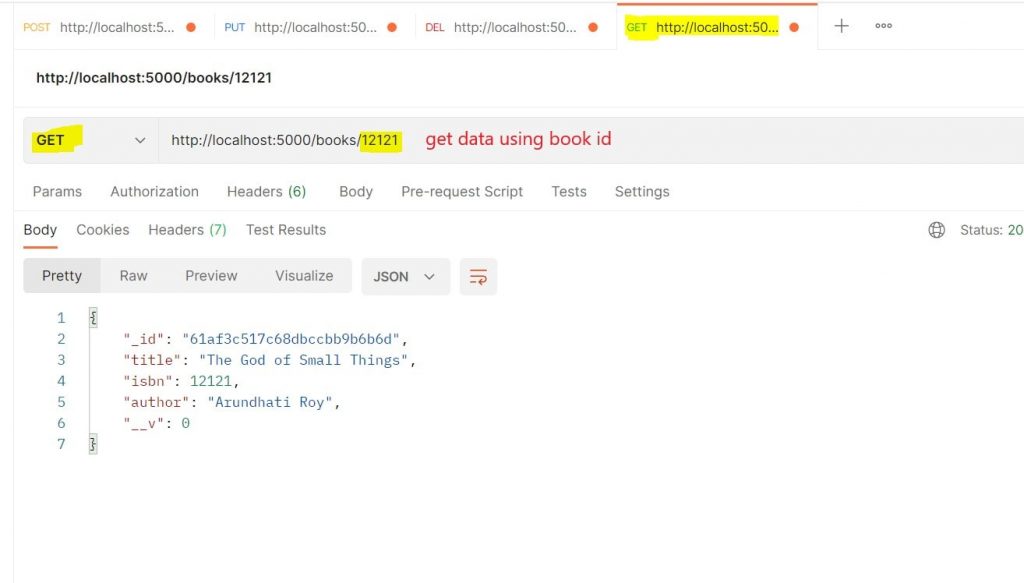
3. update book data using book id
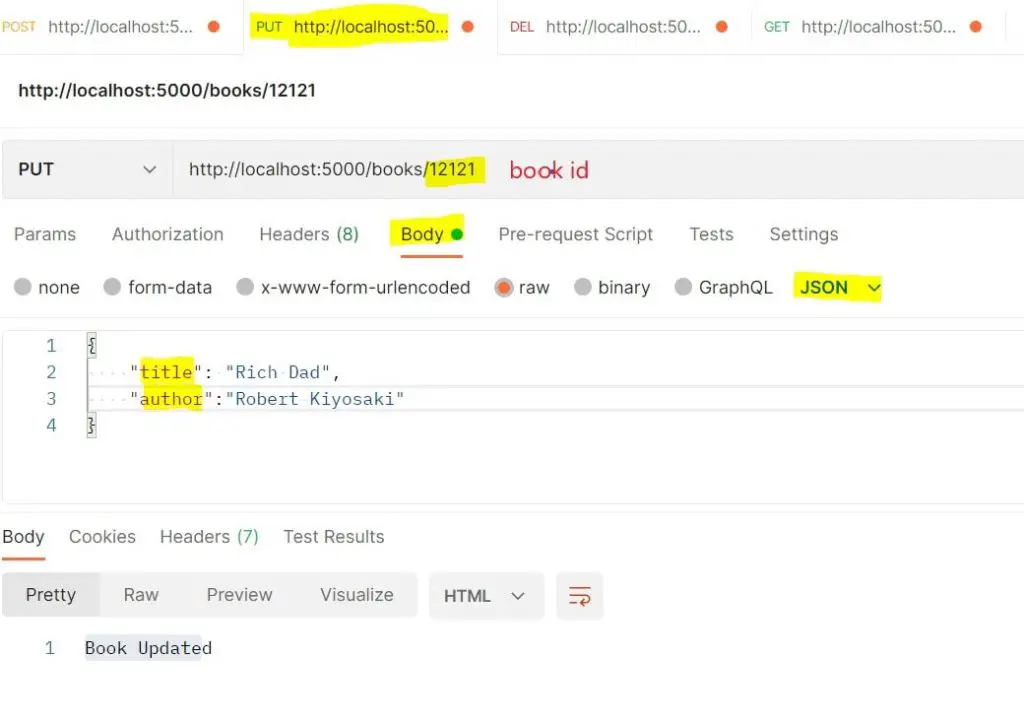
4. delete book data using book id
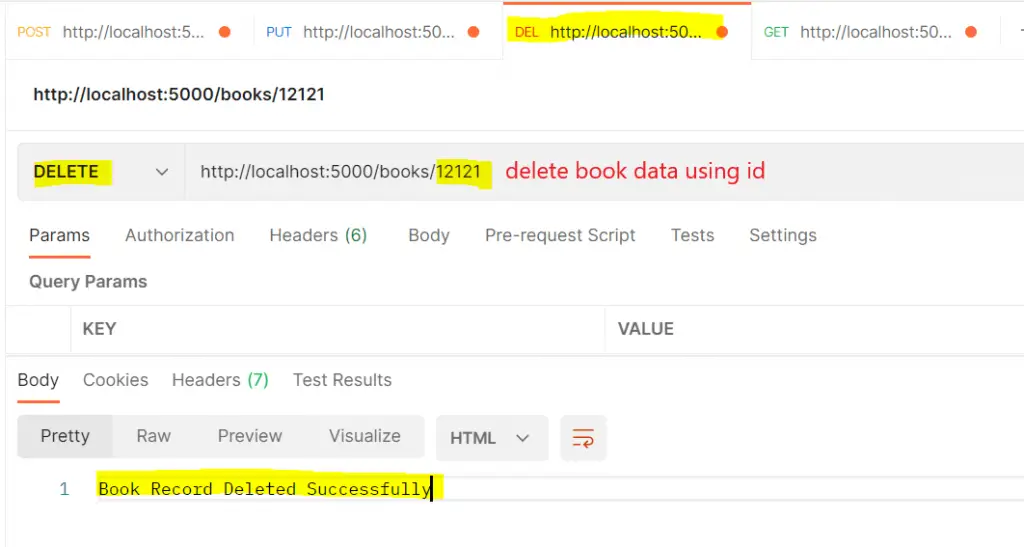