Hi Guys, Welcome to Proto Coders Point.
In this Dart Programming Tutorial, will learn what is a spy number and write a dart programs to check if the given number is spy number or not.
A spy number program is the most frequently programming logic program that is been asked in technical round of job interview.
What is a spy number
A Integer number is been split into individual digits, and the sum and product is found, if the sum & product are equal then it’s called as spy number.
Spy Number Example
Let’s us take a number ‘123’ and then split it into individual digit number (1, 2, 3) and let’s check where the number is spy or not a spy, so to find it we need to get sum and product of it Eg:
Sum = (1 + 2 + 3) = 6.
Product = ( 1 * 2 * 3) = 6
If you observe sum and product are equal, Therefore, 123 is a spy number
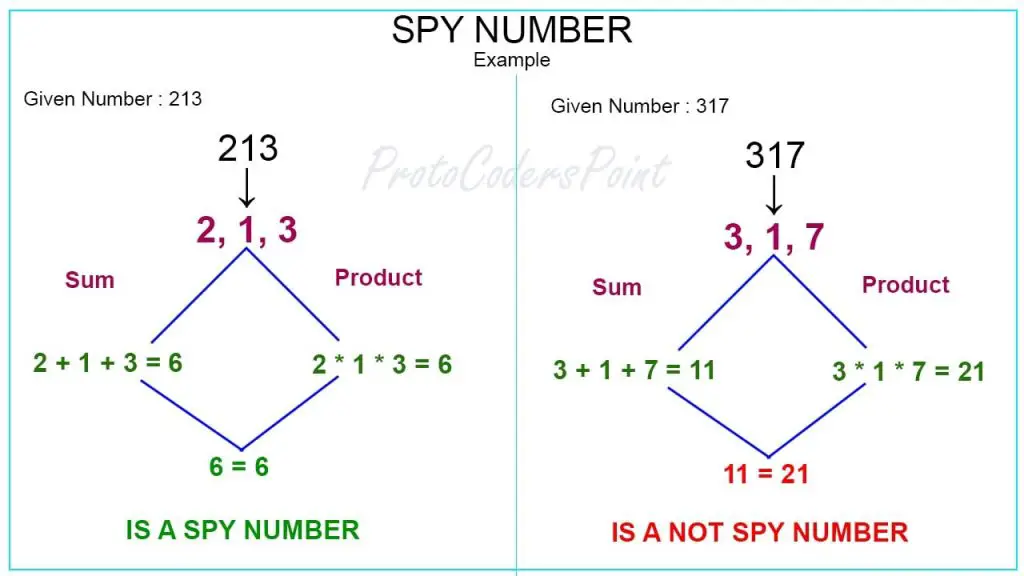
Algorithm to check spy number in dart
- Input num
- Declare three variable sum and product, lastdigit, initialize set value of sum = 0, product = 1.
- Find the last digit of given number Eg: (n%10) can be done using module operator.
- Add the last digit to sum variable Eg: sum = sum + lastdigit.
- Do multiplication using lastdigit Eg: product = product * lastdigit.
- Now remove last digit from num, to do so Divide num by 10.
- Goto step 3, loop run until num become 0.
- now if final sum and product are same/equal, then the given number(num) is a spy number else not a spy number.
Dart program to check if given number is spy number
import 'dart:io'; void main() { late int num; int product = 1, sum = 0, lastdigit; print('Enter a number to check for spy number'); num = int.parse(stdin.readLineSync()!); while(num>0){ lastdigit = num % 10; sum = sum +lastdigit; product = product * lastdigit; num = num~/10; } if(sum == product){ print("The Entered Number is Spy number"); }else{ print("The Entered Number is Not a Spy number"); } }
output
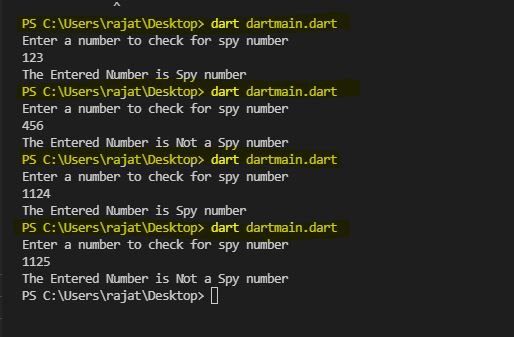
Print all spy number from 1 to n given range in dart program
import 'dart:io'; bool checkSpy(int number){ int product = 1, sum = 0, lastdigit; while(number>0){ lastdigit = number % 10; sum = sum +lastdigit; product = product * lastdigit; number = number~/10; } if(sum == product) return true; return false; } void main() { int startrange= 0, highrange = 0; print('Enter Start Range to check spy number'); startrange = int.parse(stdin.readLineSync()!); print('Enter High Range to check spy number'); highrange = int.parse(stdin.readLineSync()!); print(""); print("Spy Numbers Between $startrange to $highrange are "); for(int i=startrange; i<=highrange;i++){ if(checkSpy(i)){ print("$i"); } } }
output
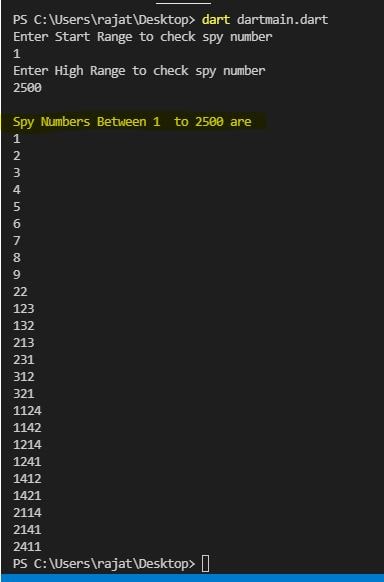