Hi Guys, Welcome to Proto Coders Point. In this article let learn about how to hide widget in flutter, i.e. using Visibility, Opacity, OffStage & difference between them & which is the best widget to hide content in flutter.
Flutter Visibility Widget
Visibility widget is to show/hide widgets, it has a visible property, which helps us in showing or hiding child, when visible is set to false, the actual child is replaced with zero- sized box instead.
Snippet
// manage visibility Visibility( // showing the child widget visible: true, // true to show, false to hide child: Text( "Visible/Hide Text Example", ), ),
Properties
visible: bool (true or false) | To hide or show the child widget |
replacement: Widget | By default,zero – sizedBox is shown, when visible is set to false, you can use any widget as replacement |
maintainSize: bool | Suppose you want a empty space when visible is false. |
maintainInteractivity: bool | User can interact with widget, even when widget is invisible. |
Flutter Opacity Widget
The opacity widget in flutter is used to make it’s child transparent, it can be done by using opacity property and set it 0, (range 0.0 - 1.0)
. The child is completely invisible when set to opacity 0, but will acquire the space & widget is also interactive.
Snippet
Opacity( opacity: _visible ? 1.0 : 0.0, child: const Text("Now you see me, now you don't!"), )
Properties
child: Widget | A widget inside opacity |
opacity: 0.0 to 1.0 | Transparent level to apply to child widget |
alwaysIncludeSemantic: bool | Semantic info about child is always included. |
Flutter OffStage Widget
OffStage in flutter widget lays it child out, as if it in true but don’t draw/render the child widget view on screen, and all don’t take space of screen.
most of the time offstage is used to measure the dimension of widget without showing it on screen.
flutter offstage example
The below example, we have a offstage widget which has child as FlutterLogo widget, when offstage is true, the offstage will be hidden so when offstage is hidden i.e. true, we will display a button to get logo size, which will show size of FlutterLogo widget in snackbar.
import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); static const String _title = 'Flutter Code Sample'; @override Widget build(BuildContext context) { return MaterialApp( title: _title, home: Scaffold( appBar: AppBar(title: const Text(_title)), body: const Center( child: MyStatefulWidget(), ), ), ); } } class MyStatefulWidget extends StatefulWidget { const MyStatefulWidget({Key? key}) : super(key: key); @override State<MyStatefulWidget> createState() => _MyStatefulWidgetState(); } class _MyStatefulWidgetState extends State<MyStatefulWidget> { final GlobalKey _key = GlobalKey(); bool _offstage = true; Size _getFlutterLogoSize() { final RenderBox renderLogo = _key.currentContext!.findRenderObject()! as RenderBox; return renderLogo.size; } @override Widget build(BuildContext context) { return Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Offstage( offstage: _offstage, child: FlutterLogo( key: _key, size: 150.0, ), ), Text('Flutter logo is offstage: $_offstage'), ElevatedButton( child: const Text('Toggle Offstage Value'), onPressed: () { setState(() { _offstage = !_offstage; }); }, ), if (_offstage) ElevatedButton( child: const Text('Get Flutter Logo size'), onPressed: () { ScaffoldMessenger.of(context).showSnackBar( SnackBar( content: Text('Flutter Logo size is ${_getFlutterLogoSize()}'), ), ); }), ], ); } }
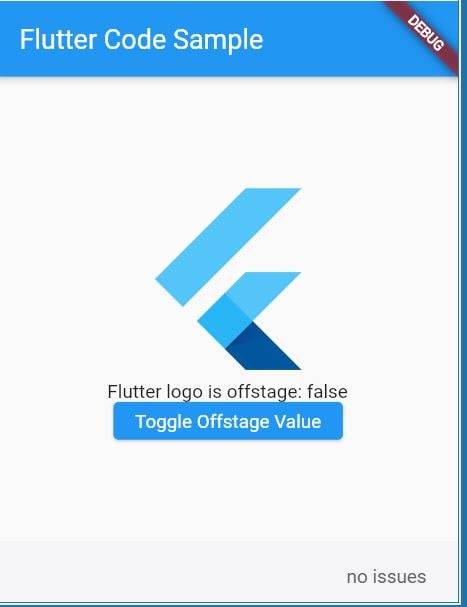
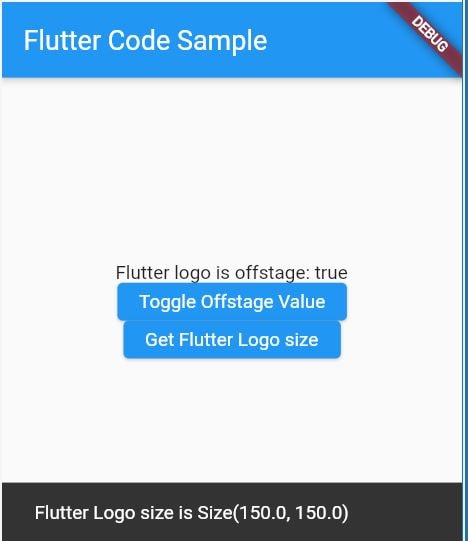
Different between Visibility, Opacity, OffStage Widget
Which is the best flutter widget to hide content.
Opacity
Alpha opacity is set to any widget you want, setting it to 0.0
is like invisible then setting to 0.1
is lightly visible and increase the visibility when range set between 0.0 to 1.0
, so i hope it easy to understand. Even after setting the opacity to 0.0
and making it invisible the widget will still occupy the space it require, because we have not actually removed the widget instead we just make is transparent, therefore user can touch and click it.
Offstage
The child widget is hidden with this one. It’s as if you put the widget “outside of the screen” so that users don’t notice it. The widget continues to progress through the flutter pipeline until it reaches the final “painting” stage, at which point it accomplishes nothing. This means that all state and animations will be preserved, but nothing will be rendered on the screen. It also won’t take up any space during layout, so there won’t be any gaps, and users won’t be able to click it.
Visibility
Visibility widget in flutter, is very user friendly and convenience, as we have discussed above when visible is set to false, the widget is completely removed and replaced with zero sizedBox, suppose if you want to keep the empty space, then make use of visibility properties like maintainAnimation
, maintainSize
, maintainState
, maintainInteractivity
etc.
when you use maintain state, it will wrap the child with Opacity
or Offstage
widget. When visible is false (i.e widget is hidden) but you have maintain size, so the empty space visible is wrapped with IgnorePointer
so that user can’t click on it, unless you make use of maintainInteractivity : true
Removing Widget
For example, you can use if (condition)
to determine whether or not a widget should be included in a list, or you can use condition? child: SizedBox()
to directly replace it with a SizedBox. This method avoids unnecessary calculations and is the most efficient.