Hi Guys, Welcome to Proto Coders Point. We will learn how to implement press back again to exit flutter app, you might have used some app that has feature like double back press to exit app, So
In this Flutter tutorial, We gonna learn how to implement flutter double back to close app.
I have already wrote an article on press again to exit flutter app using WillPopScope flutter widget. check it out or see video
In this tutorial, We will make use of a plugin called ‘double_back_to_close‘ to easily implement flutter exit app on backpress again.
Let’s get Started
Video Tutorial
Using Double back to close as library
To use it you need to install it as dependencies in your flutter project.
1. Open a Flutter Project
In your favorite IDE, open existing flutter project or create a new project as usual.
2. Add Dependencies
Now, Open pubspec.yaml & add below line in dependencies section.
dependencies: double_back_to_close:
Then hit pub get or run flutter pub get cmd
3. Import double_back_to_close.dart
Now to implement press back again to exit, you need to import it where required.
import 'package:double_back_to_close/double_back_to_close.dart';
How to use DoubleBack Widget
Just wrap DoubleBack Widget to root page, i mean Home page or Dashboard Page.
For example: Support you have a login page & after successful login you navigate user to dashboad/home page and from there, you want to app press back to exit feature.
Properties of Double Back Widget
Properties | |
child: | Load the page you want to show. |
message: | A message that pop up in Toast Message. |
waitForSecondBackPress: | Time for second back press to exit, default is 2 sec. |
onFirstBackPress: | A Callback function, which is called when user click back button once. |
textStyle: | Change text styling of toast meesage. |
background: | Change background color. |
backgroundRadius: | Alter Radius of toast message |
condition: | show press back again to exit, only when specific conditin is true Eg: if user is on HomePage then exit if user is in some other page do nothing. |
Default ( Toast message before exit app)
class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const DoubleBack( message: "Back press again to Exit App", child: MyHomePage(title: 'Flutter Demo Home Page') ), ); } }
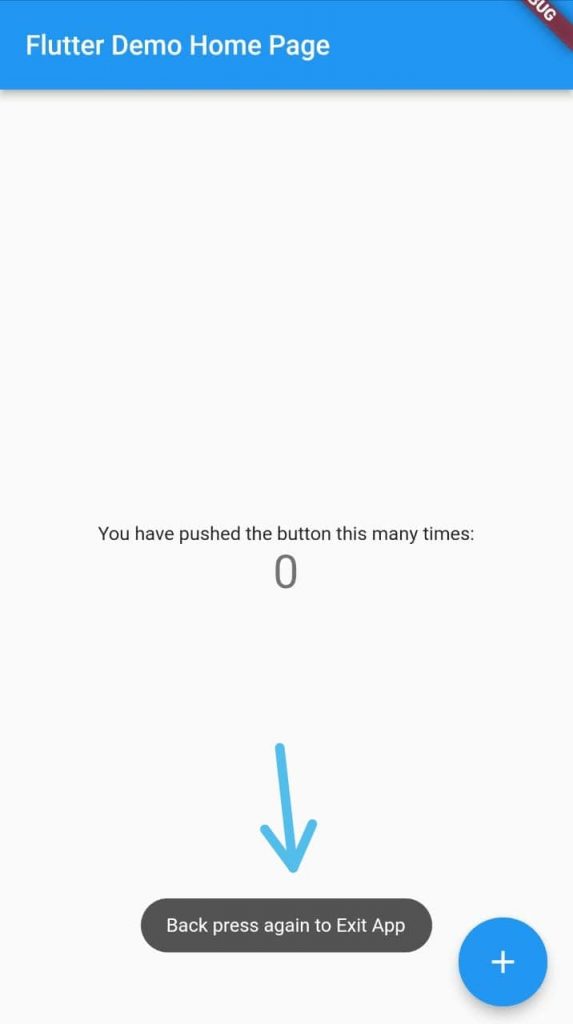
Custom Styling to toast message on backpress to exit
class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const DoubleBack( message: "Back press again to Exit App", textStyle: TextStyle(fontSize: 15,color: Colors.white), background: Colors.red, backgroundRadius: 10, child: MyHomePage(title: 'Flutter Demo Home Page') ), ); } }
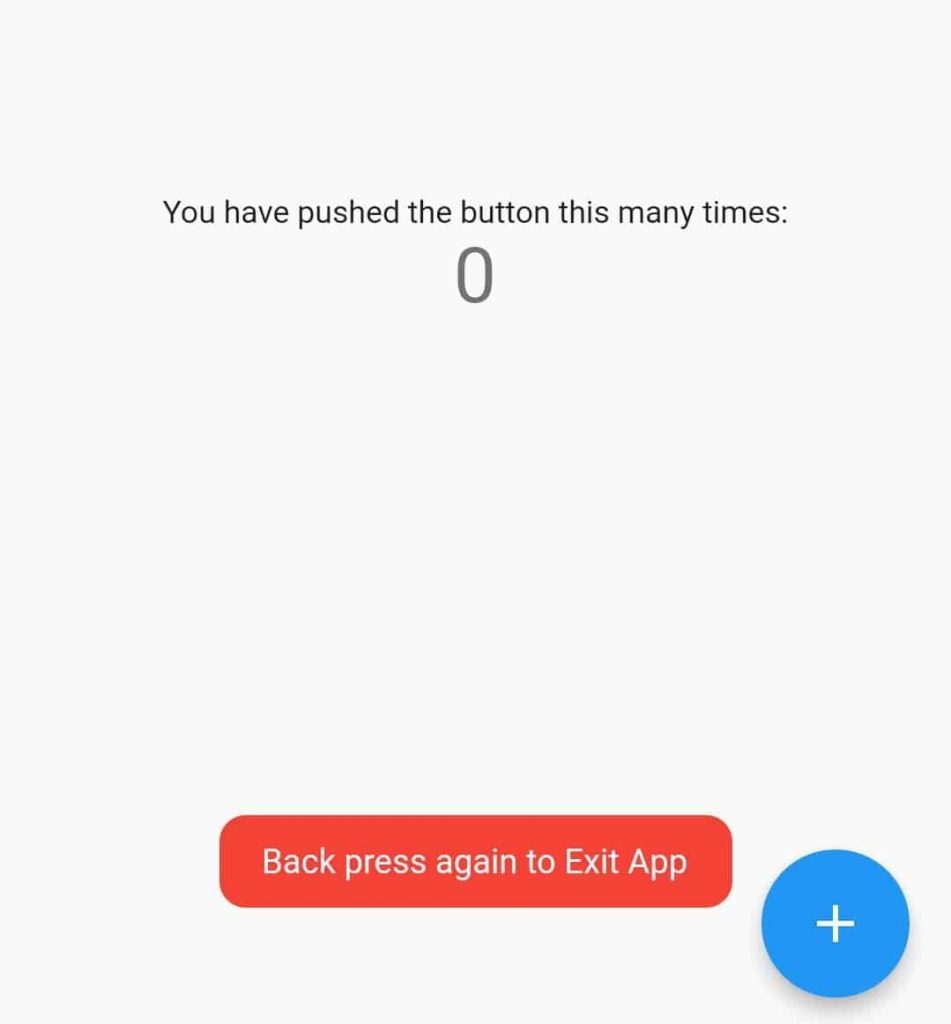
Show snackbar on Press again to exit app
class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: DoubleBack( onFirstBackPress: (context){ final snackBar = SnackBar(content: Text("Double back Press to exit App"),); ScaffoldMessenger.of(context).showSnackBar(snackBar); }, textStyle: TextStyle(fontSize: 15,color: Colors.white), background: Colors.red, backgroundRadius: 10, child: MyHomePage(title: 'Flutter Demo Home Page') ), ); } }
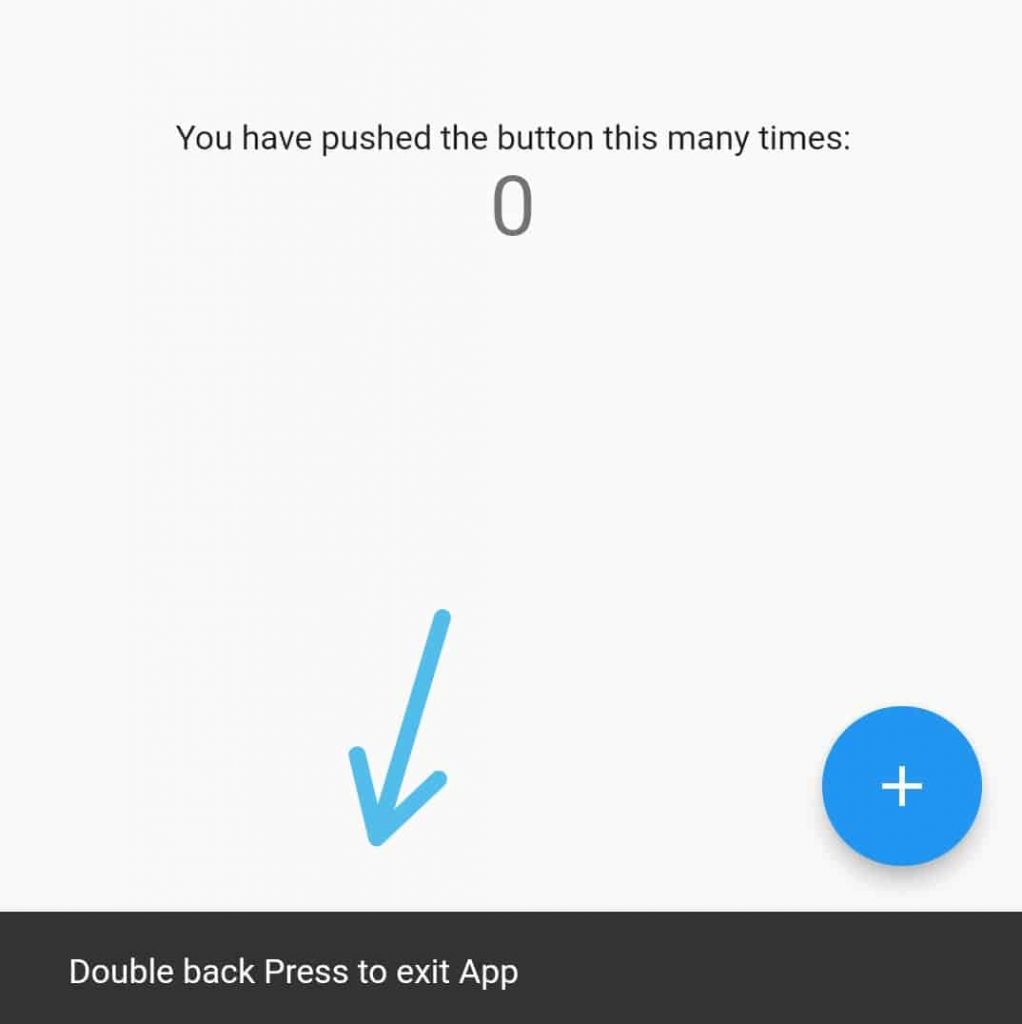
Custom condition
Show press back again to exit, only when specific condition is true Eg: if user is on HomePage then exit the app, if user is in some other page do other event.
class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( // Imagine that you are using materialbottom and only want to close when tabIndex = 0 home: DoubleBack( condition: tabIndex == 0, // only show message when tabIndex=0 onConditionFail: (){ setState((){ tabIndex = 0; // if not 0, set pageview jumptopage 0 }); } child: Home(), ), ); } }