Hi Guys, Welcome to Proto Coders Point. In this Article will learn errors handling in flutter.
What is your code throws an error, suppose say initState method throws an error, this will simply return a red color screen with the error message printed in it, and no developer likes to see read color error screen in flutter development.
So here, In this flutter article, I have a solution for you, so that you learn how to handle errors in flutter by showing error with beautiful custom build design for error screen, so that we get rid of the red exception error page in flutter.
Error Widget in flutter
A ErrorWidget is an widget that is build specially for error handling, so this widget will render a separate custom build exception message screen when error occurs.
Flutter ErrorWidget is used when our app fails to build methods. and we can show error with custom UI, it will also help determine where the problem lies exactly.
How to show user friendly error page in flutter
It’s possible to show a custom UI error handling page by making use if ErrorWidget.builder (code example is below). Instead of Red error exception.
If your flutter app is published & is been used by lot’s of user, then at some point of time if error occurs then user might see red exception which is not good as user-experience & might leave a negative feedback about our app.
So it better to implement an custom global error handling in flutter by which we can show a user-freindly error page rather then red color error page with message.
How to use Flutter Error Widget
In void main(), before runApp(), add ErrorWidget.builder as shown below(UI Design in MaterialApp, customize as per your for error UI design).
void main() { // Set the ErrorWidget's builder before the app is started. ErrorWidget.builder = (FlutterErrorDetails details) { return MaterialApp( .... ); }; // Start the app. runApp(const MyApp()); }
Now, throws error, from where you think error might occur. Just for an example I am throwing error from initState() method as below:
@override void initState() { // TODO: implement initState super.initState(); throw("Error thrown from initState(), This is Dummy Error Example"); }
How to use ErrorWidget Builder in Flutter
Here is a example, The error is throws then user clicks on “don’t click me” button.
Complete Code with custom UI design for Error Widget for Error handling in flutter below. main.dart
import 'package:flutter/material.dart'; void main() { // Set the ErrorWidget's builder before the app is started. ErrorWidget.builder = (FlutterErrorDetails details) { // In release builds, show a yellow-on-blue message instead: return MaterialApp( home: Scaffold( body: Center( child: Container( alignment: Alignment.center, width: 250, height: 200, decoration: BoxDecoration( borderRadius: BorderRadius.circular(10), color: Colors.amber[300], boxShadow: [ BoxShadow(color: Colors.green, spreadRadius: 3), ], ), child: Text( ' Error!\n ${details.exception}', style: const TextStyle(color: Colors.red,fontSize: 20), textAlign: TextAlign.center, textDirection: TextDirection.ltr, ), ), ),), ); }; // Start the app. runApp(const MyApp()); } class MyApp extends StatefulWidget { const MyApp({Key? key}) : super(key: key); static const String _title = 'ErrorWidget Sample'; @override State<MyApp> createState() => _MyAppState(); } class _MyAppState extends State<MyApp> { bool throwError = false; @override Widget build(BuildContext context) { if (throwError) { // Since the error widget is only used during a build, in this contrived example, // we purposely throw an exception in a build function. return Builder( builder: (BuildContext context) { throw Exception('oh no, You clicked on Button..! | Error Caught'); }, ); } else { return MaterialApp( title: MyApp._title, home: Scaffold( appBar: AppBar(title: const Text(MyApp._title)), body: Center( child: TextButton( onPressed: () { setState(() { //by setting throwError to true, above if condition will execute. throwError = true; }); }, child: const Text('Do Not Click me')), ), ), ); } } }
Result of ErrorWidget for Error handling in flutter
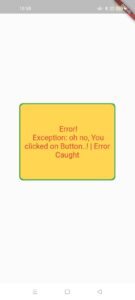