Hi Guy’s Welcome to Proto Coders Point, In this Flutter Article let’s learn how to implement Alphabetical scroll list in flutter.
An Alphabetical Scroll List also known as A-Z Scroll List, is the most commonly used in mobile applications that help users to quickly navigate to any large set of list in an alphabetical order.
Here are some common places where an Aplhabetical Scroll List can be used:
- Contact List.
- Music & Video Playlist.
- Address Books.
- Product Lists.
- Country or City List.
An Alphabetical Scroll List is versatile UI element that ca be applied in many use case like contact list, music, video, address, country and many more for efficient navigation through the large dataset.
How to Implement alphabetical scroll list in flutter
In Flutter to create an alphabetical listview which can be scrollable using easily A-Z Alphabet, We will be using a dependency/library called azlistview that allow scrolling to a specific items in the list for easy navigation.
Video Tutorial
Install Library into flutter project
Open pubspec.yaml file and under dependencies section add:
dependencies: azlistview: ^2.0.0 #version may change in future
After adding the dependencies hit pub get button or enter “flutter pub get” in terminal to download the package into your flutter project as external packages.
Import to use it
import 'package:azlistview/azlistview.dart';
Source Code to Implement A-Z Scroll list in flutter
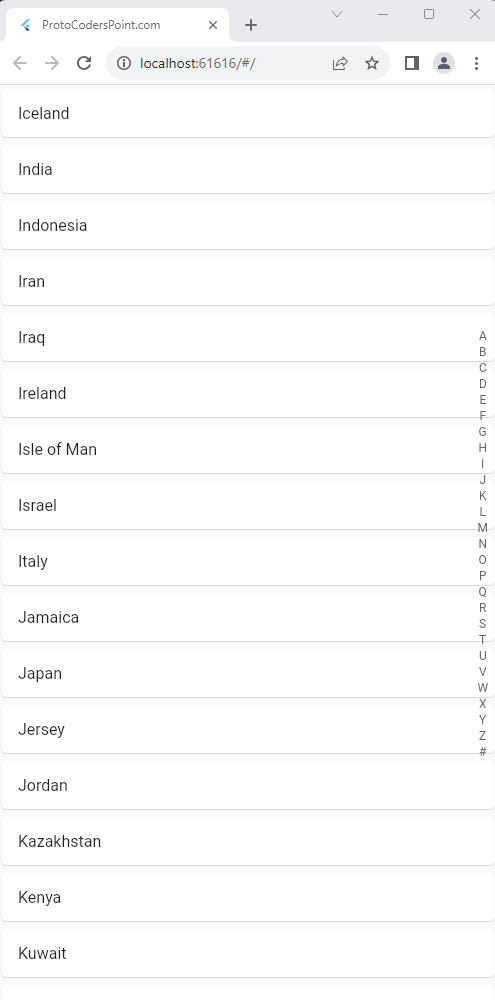
In “lib” directory of your flutter project, Create a Dart File by name “CountryList.dart”
CountryList.dart
This will be a class that extends ISuspensionBean which comes with azlistview package.
import 'package:azlistview/azlistview.dart'; class CountryList extends ISuspensionBean{ final String title; final String tag; CountryList({required this.title, required this.tag}); @override String getSuspensionTag() { // TODO: implement getSuspensionTag return tag; } }
main.dart
In this page we will load the list of country name which can we easily alphabetically scrolled to navigate to particular items in the listview using A-Z.
import 'dart:typed_data'; import 'package:azlistview/azlistview.dart'; import 'package:flutter/material.dart'; import 'package:flutter_ui/CountryList.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( // hide the debug banner debugShowCheckedModeBanner: false, title: 'ProtoCodersPoint.com', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( body: SafeArea( child: AlphabeticalListView(items: [ "Afghanistan", "Albania","Algeria","Andorra","Angola","Anguilla","Antigua & Barbuda","Argentina","Armenia","Aruba","Australia","Austria","Azerbaijan","Bahamas","Bahrain","Bangladesh","Barbados","Belarus","Belgium","Belize","Benin","Bermuda","Bhutan","Bolivia","Bosnia & Herzegovina","Botswana","Brazil","British Virgin Islands","Brunei","Bulgaria","Burkina Faso","Burundi","Cambodia","Cameroon","Cape Verde","Cayman Islands","Chad","Chile","China","Colombia","Congo","Cook Islands","Costa Rica","Cote D Ivoire","Croatia","Cruise Ship","Cuba","Cyprus","Czech Republic","Denmark","Djibouti","Dominica","Dominican Republic","Ecuador","Egypt","El Salvador","Equatorial Guinea","Estonia","Ethiopia","Falkland Islands","Faroe Islands","Fiji","Finland","France","French Polynesia","French West Indies","Gabon","Gambia","Georgia","Germany","Ghana","Gibraltar","Greece","Greenland","Grenada","Guam","Guatemala","Guernsey","Guinea","Guinea Bissau","Guyana","Haiti","Honduras","Hong Kong","Hungary","Iceland","India","Indonesia","Iran","Iraq","Ireland","Isle of Man","Israel","Italy","Jamaica","Japan","Jersey","Jordan","Kazakhstan","Kenya","Kuwait","Kyrgyz Republic","Laos","Latvia","Lebanon","Lesotho","Liberia","Libya","Liechtenstein","Lithuania","Luxembourg","Macau","Macedonia","Madagascar","Malawi","Malaysia","Maldives","Mali","Malta","Mauritania","Mauritius","Mexico","Moldova","Monaco","Mongolia","Montenegro","Montserrat","Morocco","Mozambique","Namibia","Nepal","Netherlands","Netherlands Antilles","New Caledonia","New Zealand","Nicaragua","Niger","Nigeria","Norway","Oman","Pakistan","Palestine","Panama","Papua New Guinea","Paraguay","Peru","Philippines","Poland","Portugal","Puerto Rico","Qatar","Reunion","Romania","Russia","Rwanda","Saint Pierre & Miquelon","Samoa","San Marino","Satellite","Saudi Arabia","Senegal","Serbia","Seychelles","Sierra Leone","Singapore","Slovakia","Slovenia","South Africa","South Korea","Spain","Sri Lanka","St Kitts & Nevis","St Lucia","St Vincent","St. Lucia","Sudan","Suriname","Swaziland","Sweden","Switzerland","Syria","Taiwan","Tajikistan","Tanzania","Thailand","Timor L'Este","Togo","Tonga","Trinidad & Tobago","Tunisia","Turkey","Turkmenistan","Turks & Caicos","Uganda","Ukraine","United Arab Emirates","United Kingdom","Uruguay","Uzbekistan","Venezuela","Vietnam","Virgin Islands (US)","Yemen","Zambia","Zimbabwe" ],), ), ); } } class AlphabeticalListView extends StatefulWidget { final List<String> items; AlphabeticalListView({Key? key, required this.items}) : super(key: key); @override State<AlphabeticalListView> createState() => _AlphabeticalListViewState(); } class _AlphabeticalListViewState extends State<AlphabeticalListView> { List<CountryList> items = []; @override void initState() { // TODO: implement initState super.initState(); initList(widget.items); } void initList(List<String> items){ this.items = items.map((item) => CountryList(title: item,tag: item[0])).toList(); } @override Widget build(BuildContext context) { return AzListView(data: items, itemCount: items.length, itemBuilder: (context,index){ final item = items[index]; return Card( child: ListTile( title: Text(item.title), ), ); }); } }