Hi Guy’s Welcome to Proto Coders Point. This article is on how to upload files to Google Drive using NodeJS.
Uploading a file to google drive by using nodejs code take several steps, which include steps:
- Creating Project in Google Cloud Console.
- Enabling Google Drive API.
- Creating Service Account Credentials.
- Creating NodeJS Project and Install Necessary Libraries.
- NodeJS Code to Upload File to Google Drive.
Step 1: Setup a Google Cloud Console Project:
- Go to Google Cloud Console : https://console.cloud.google.com/.
- Create a new Project or select an existing one.
- Now Navigate to “API’s & Services” > “Library”.
- In Search for “Google Drive API” and click on enable button for your project.
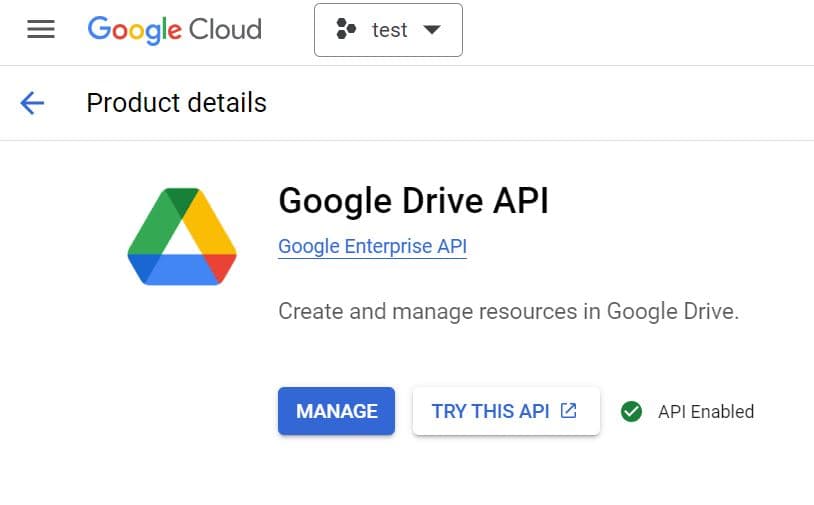
Step 2: Create Service Account Credentials API
In google cloud console, Navigate to “API’s & Services” > “Credentials”.
Click on “Create Credentials” > Select “Service Account”.
Enter Service Account Name, Service account ID then click DONE.
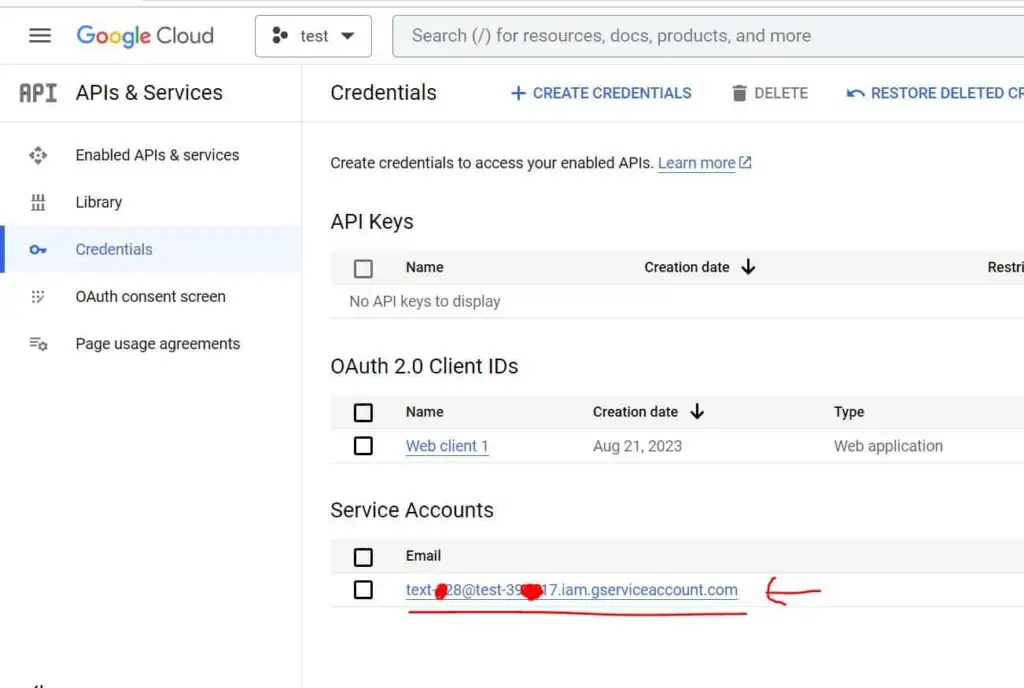
Step 3: Creating API Key JSON File for authentication
Once Service Account is been created, click on Service Account Email as soon in above image.
Then Click on Keys > Add Key > Create New Key
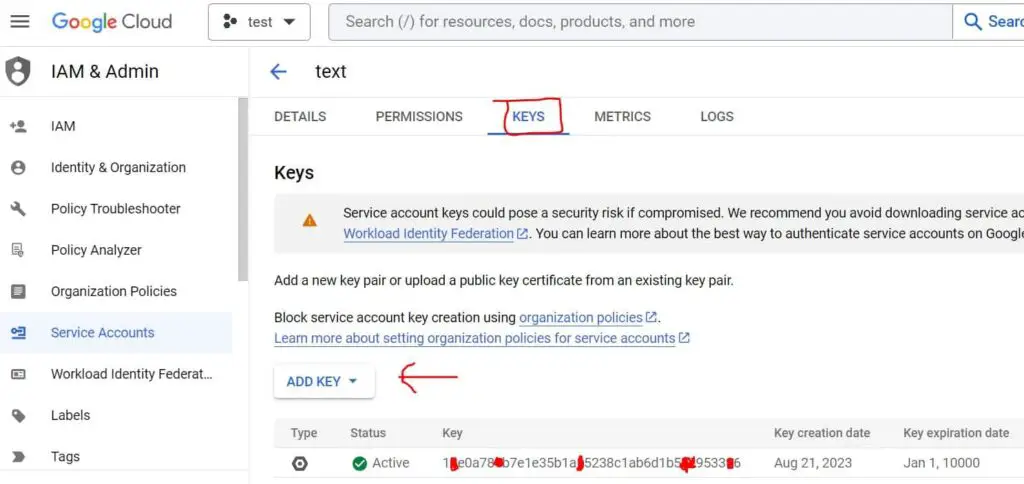
This will create a json file download it, It contain api key by making use of which you can write a nodeJS Script that can upload files into your google drive.
Step 4: Creating a Folder & Give Access
Now, Once Service Account email is created, We need to create a Folder on Google Drive that will have full access permission for the service account we created, Basically share that folder with Service Account we created in above step.
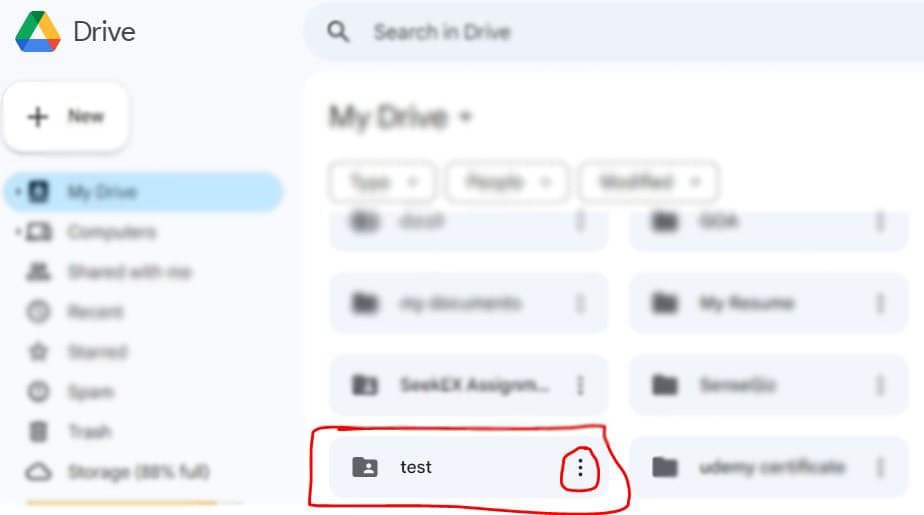
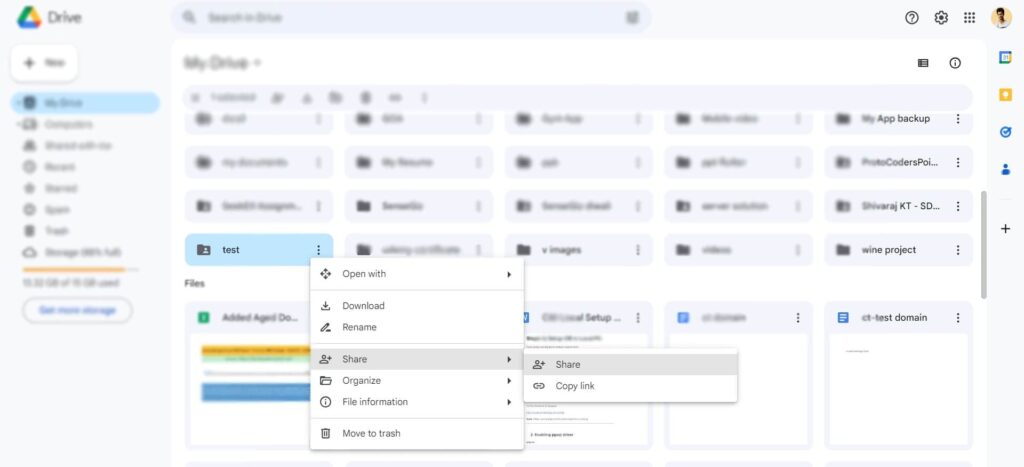
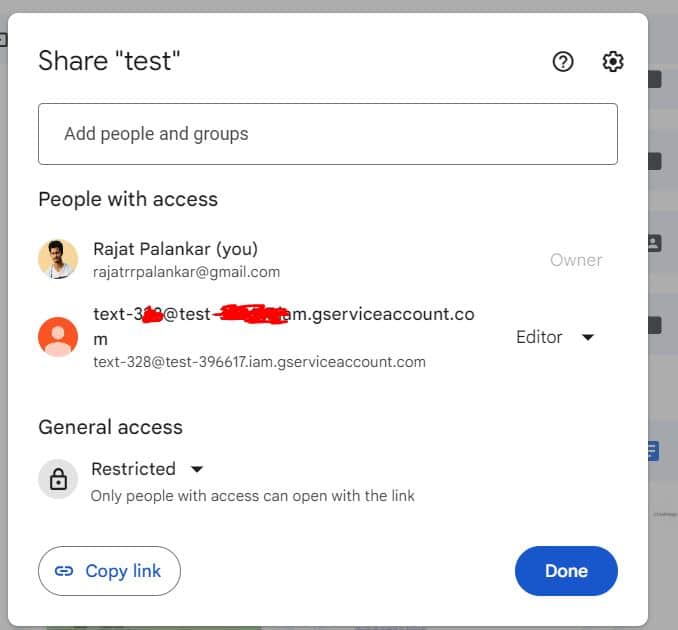
Click on 3 dot on the folder that you want to share with service account, then click on share and enter the email address of service account and done.
Step 5: Creating NodeJS Project
Create a folder & open a terminal in that folder path itself.
In Terminal/CMD enter npm init to make normal folder into nodeJS project
Step 6: Installing required Library/Module
To upload files into google drive from Nodejs script we need few library:
fs (File Sytem) -> To work with Files of System.
googleapis -> To communicate with google drive API.
Install those using below cmd:
npm install fs googleapis
Step 7: Finally NodeJS Script/Function to Upload file to Google Drive using Google Drive API
In your NodeJS Project, paste the credential .json file download from above step.
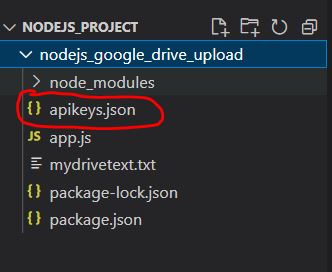
In my project I have created a javascript code by name app.js, what will have a function that will upload file to google drive.
app.js
const fs = require('fs'); const { google }= require('googleapis'); const apikeys = require('./apikeys.json'); const SCOPE = ['https://www.googleapis.com/auth/drive']; // A Function that can provide access to google drive api async function authorize(){ const jwtClient = new google.auth.JWT( apikeys.client_email, null, apikeys.private_key, SCOPE ); await jwtClient.authorize(); return jwtClient; } // A Function that will upload the desired file to google drive folder async function uploadFile(authClient){ return new Promise((resolve,rejected)=>{ const drive = google.drive({version:'v3',auth:authClient}); var fileMetaData = { name:'mydrivetext.txt', parents:['1bZoTbqCew34MGr1DfgczcA40ECM_QhKg'] // A folder ID to which file will get uploaded } drive.files.create({ resource:fileMetaData, media:{ body: fs.createReadStream('mydrivetext.txt'), // files that will get uploaded mimeType:'text/plain' }, fields:'id' },function(error,file){ if(error){ return rejected(error) } resolve(file); }) }); } authorize().then(uploadFile).catch("error",console.error()); // function call
Now run the code
node app.js