Hi Guy’s Welcome to Proto Coders Point, In this dart article let’s checkout how to sort a map by its value.
Suppose you have a map like this:
final Map<String,int> mayData = { 'a' : 100, 'b' : 90, 'c' : 50, 'd' : 150, 'e' : 200, 'r' : 600, };
And your task is to sort this map in a form of ascending/descending order as per the project requirement.
The solution to sort map values in ascending or descending order is quite easily & simple then you thing it is.
Flutter Dart Sort map in ascending order by its values
Video Tutorial
void main() { final Map<String,int> mapData = { 'a' : 100, 'b' : 90, 'c' : 50, 'd' : 150, 'e' : 200, 'f' : 600, }; // sorting the map value in ascending order by it's value. // convert the map data into list(array). List<MapEntry<String,int>> listMappedEntries = mapData.entries.toList(); // Now will sort the list listMappedEntries.sort((a,b)=> a.value.compareTo(b.value)); // list is been sorted // now convert the list back to map after sorting. final Map<String,int> sortedMapData = Map.fromEntries(listMappedEntries); print(sortedMapData); }
Output
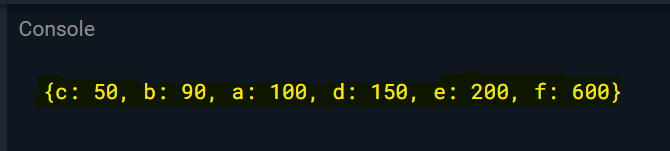
Flutter Dart Sort map in descending order by its values
The code is similar to above example, The only thing is while sorting compering, We must compare reverse i.e.
Instead if this:
listMappedEntries.sort((a,b)=> a.value.compareTo(b.value))
Do this:
listMappedEntries.sort((a,b)=> b.value.compareTo(a.value))
Video Tutorial
Complete Code
void main() { final Map<String,int> mapData = { 'a' : 100, 'b' : 90, 'c' : 50, 'd' : 150, 'e' : 200, 'f' : 600, }; // sorting the map value in ascending order by it's value. // convert the map data into list(array). List<MapEntry<String,int>> listMappedEntries = mapData.entries.toList(); // Now will sort the list in descending order listMappedEntries.sort((a,b)=> b.value.compareTo(a.value)); // list is been sorted // now convert the list back to map after sorting. final Map<String,int> sortedMapData = Map.fromEntries(listMappedEntries); print(sortedMapData); }
Output
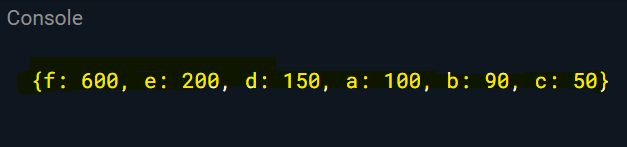
Flutter map sort by key example
All you need to do is use inbuilt sort function and compare map key with key, and thus map will get sorted by key.
listMappedEntries.sort((a,b)=> a.key.compareTo(b.key));
Complete Code
void main() { final Map<String,int> mapData = { 'a' : 100, 'z' : 90, 'f' : 50, 'b' : 150, 'n' : 200, 'r' : 600, }; // sorting the map value in ascending order by it's value. // convert the map data into list(array). List<MapEntry<String,int>> listMappedEntries = mapData.entries.toList(); // dart map sort by key listMappedEntries.sort((a,b)=> a.key.compareTo(b.key)); // list is been sorted // now convert the list back to map after sorting. final Map<String,int> sortedMapData = Map.fromEntries(listMappedEntries); print(sortedMapData); }
Output
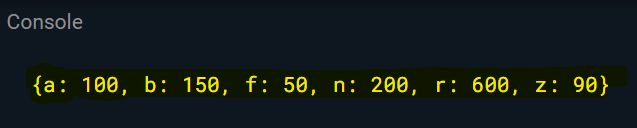
Similar Article Recommended
Sum of all values in map flutter