Hi Guys Welcome to Proto Coders Point, In this Flutter tutorial we will check how to encrypt password in flutter using flutter string encryption.
Video Tutorial
Flutter string encryption Package Plugin
This package is very useful for string encryption, it’s a Cross platform string encryption which uses commonly best Encrytion methods like (AES/CBC/PKCS5/RandomIVs/HMAC-SHA256 Integrity Check).
Installation of Flutter Encryption package
1. Adding Dependencies in pubspec.yaml file
open your flutter project that you have created in your IDE(android-studio).
In your project structure you may see a file by name pubspec.yaml name open, in this file under dependencies add the package
dependencies: flutter_string_encryption: ^0.3.1 // add this line // package version may change kindly check update version on offical site
2. Importing the package
Then after you have added the required flutter encryption package now you need to import the package where you will be using it in your code to encrypt or decrypt the string or the password the user enter.
import 'package:flutter_string_encryption/flutter_string_encryption.dart';
So now the flutter encryption and decryption package is been successfully added into your flutter project, now you can use it.
So now let’s check how to encrypt a string using flutter string encryption
Usage of the library
// create a PlatformStringCryptor
In Below Snippet code we have creating an object of PlatformStringCryptor that will help use in creatng salt and to generate key.
final cryptor = new PlatformStringCryptor();
// generate a Salt string using Cryptor
final String salt = await cryptor.generateSalt();
//generate a key from password
Here password is the text that user enter in textfield
final String key = await cryptor.generateKeyFromPassword(password, salt);
Encrypt A String
final String encrypted = await cryptor.encrypt("Password that you want to encrypt", key);
Decrypt A String
try { final String decrypted = await cryptor.decrypt(encrypted, key); print(decrypted); // - A string to encrypt. } on MacMismatchException { // unable to decrypt (wrong key or forged data) }
IMPORTANT NOTE
Then key that is used to Encrypt a string should be same while you want to decrypt the encrypted string.
Implementing Flutter Encryption decryption in Flutter Project
main.dart
import 'dart:math'; import 'package:flutter/material.dart'; import 'package:flutter_string_encryption/flutter_string_encryption.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', debugShowCheckedModeBanner: false, theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { TextEditingController pass = TextEditingController(); var key = "null"; String encryptedS,decryptedS; var password = "null"; PlatformStringCryptor cryptor; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Password Encrypt"), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Padding( padding: const EdgeInsets.fromLTRB(30, 0, 30, 0), child: TextField( controller: pass, decoration: InputDecoration( hintText: 'Password', hintStyle: TextStyle(color: Colors.black), enabledBorder: OutlineInputBorder( borderRadius: new BorderRadius.circular(10.0), borderSide: BorderSide( color: Colors.red ), ), focusedBorder: OutlineInputBorder( borderRadius: new BorderRadius.circular(10.0), borderSide: BorderSide( color: Colors.blue[400] ) ), isDense: true, // Added this contentPadding: EdgeInsets.fromLTRB(10, 20, 10, 10), ), cursorColor: Colors.white, ), ), RaisedButton( onPressed: (){ Encrypt(); }, child: Text("Encrypt"), ), RaisedButton( onPressed: (){ Decrypt(); }, child: Text("Decrypt"), ), ], ), ), ); } // method to Encrypt String Password void Encrypt() async{ cryptor = PlatformStringCryptor(); final salt = await cryptor.generateSalt(); password = pass.text; key = await cryptor.generateKeyFromPassword(password, salt); // here pass the password entered by user and the key encryptedS = await cryptor.encrypt(password, key); print(encryptedS); } // method to decrypt String Password void Decrypt() async{ try{ //here pass encrypted string and the key to decrypt it decryptedS = await cryptor.decrypt(encryptedS, key); print(decryptedS); }on MacMismatchException{ } } }
Output
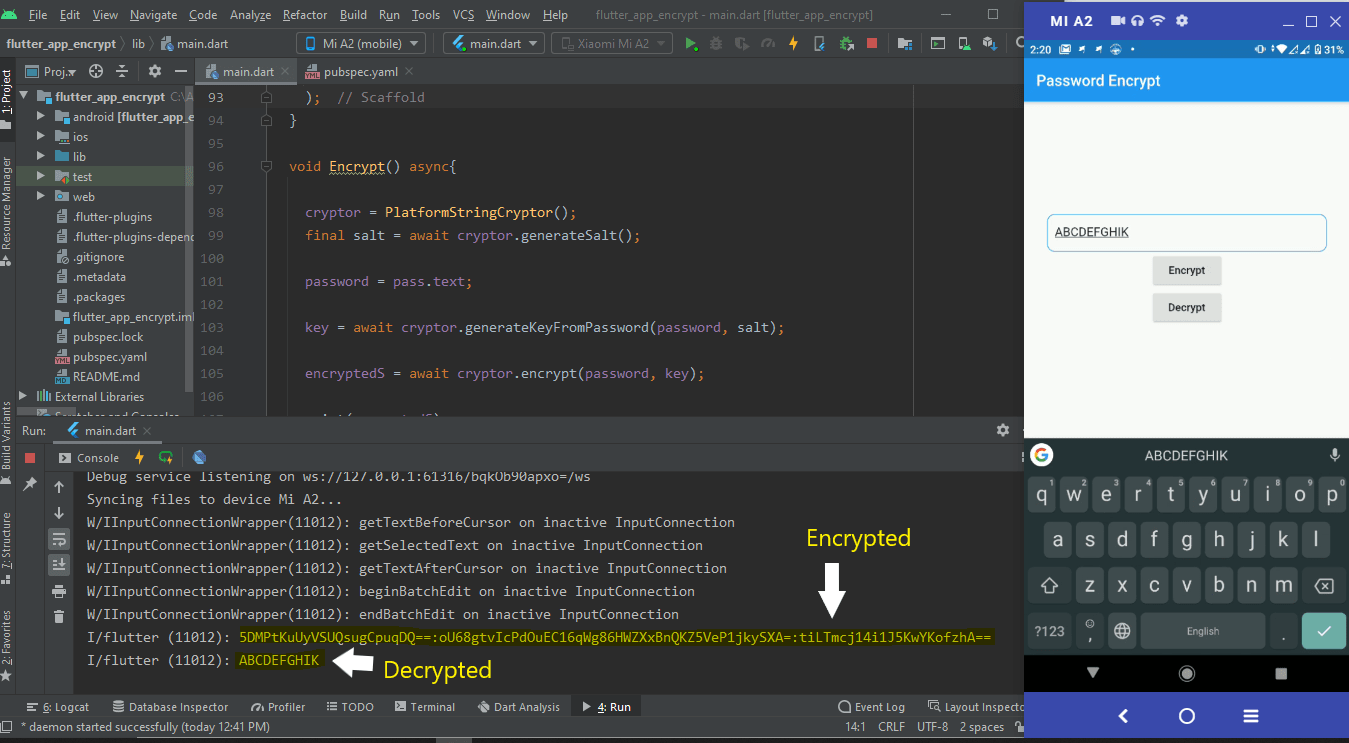