Hi Guys, Welcome to Proto Coders Point, In this tutorial we will learn about arrays in dart flutter with an example
What is array in dart?
Same as other programming language, In dart also the defination of array is same.
An array in an object that can be used to store a list of collections of elements. In array list the collection can be of any datatype: numbers, String, etc.
In Flutter dart, array can be used to store multiple values in one datatype variable.
To access the value/element in the array list then you can do it through it index id.
The value stored in an array are called as elements.
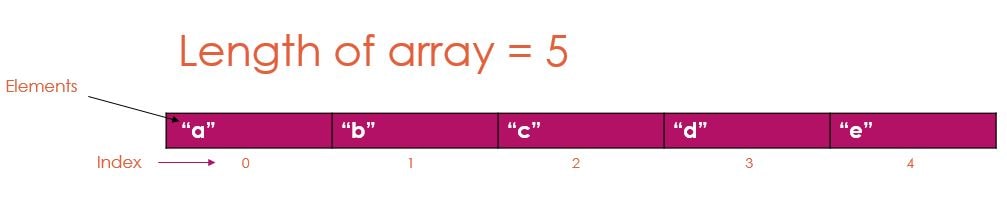
Initializing arrays in Dart
Using literal constructor
Using Literal constructor [] an new array can be created: as shown in below example:
import 'dart:convert'; void main() { var arr = ['a','b','c','d','e']; // array literal constructor print(arr); //print the array on the screen }
How to print array in flutter?
To print array list on the screen or on console log then
you can make use of print cmd to print on console
else if you want to display array in your flutter app then you can make use of Text widget to show the array list
new keyword and List
import 'dart:convert'; void main() { var arr = new List(5);// creates an empty array of length 5 // assigning values to all the indices //adding data to array list arr[0] = 'a'; arr[1] = 'b'; arr[2] = 'c'; arr[3] = 'd'; arr[4] = 'e'; print(arr); }
How to access particular array element? Method to read array list
first() |
Return first element of the array. |
last() |
last element of the array. |
isEmpty() |
It returns true if the list is empty; otherwise, it returns false . |
length() |
It returns the length of the array. eg : 5 |
Example
import 'dart:convert'; void main() { var arr = ['a','b','c','d','e']; print(arr.first);// first element of array print(arr.last);// last element of array print(arr.isEmpty);// to check whether the array is empty or not print(arr.length);// the lenght of the array }
Recommended Article
Dart data types – variable in dart
Flutter array – array in flutter
List in dart – convert list to set or vice versa