Hi Guys, Welcome to Proto Coders Point, In this flutter tutorial we will cover flutter logger & how to log data in a flutter to help developers in debugging & improving projects for long-term maintenance.
Please note that in this flutter tutorial we will not cover logging in file or logging to remote service.
What is Logger – flutter?
A logger object is used to log/show messages on the IDE console Logcat window.
In Flutter, logging is the most important & useful feature provided that helps developers track, trace, find out the error log & debug logger error.
How to var log message in flutter?
Generally logging messages in flutter can be done using 2 ways
1. Using stdout & stderr
Generally printing a message on the console screen is done using the print() method by importing ‘dart:io’ & by invoking stdout & stderr.
Eg:
stdout.writeln("This log is using stdout"); stderr.writeln("This log is using stderr");
Output on Console

Using Print() method Example
print("This Message using Print method");

Suppose, the log message or error log message is long the android just ignores some of the log & print only half of it, In that case you can use ‘debugPrint()’ it will print whole list of log data error.
2. Other is using ‘dart:developer” log() function
This allows developer to include a bit more information in logging output messages.
So to use developer.log function in flutter print to console or devtool, you need to just import ‘dart:developer’ as devlog & now use it as shown below ( devlog.log(“This is Log message from developer log method”); )
import 'dart:developer' as devlog; void main() { devlog.log("This is developer log func message",name:'MyLog'); }
output: developer.log console log will only be show in devTool

So now you have seen above log method are default dart logs
Here i have found out best flutter logger library that help in printing different kind of logs on console.
Best Flutter Library to log message on console
Flutter Logger Library
Flutter Logger library is very helpful for developer because it help usin printing log message on console n beautiful format.
We all use default log messages to print some statements on the console, as shown above, but they get missed & are difficult to find & might not even see them.
How to use flutter logger library
Learn more obout Logger from Official site
1. Add dependencies
Open pubspec.yaml > under dependenices section add logger: ^1.0.0
dependencies: logger: ^1.0.0 #version might change
2. Import the logger dart class
Then to make use of logger flutter you need to import the logger.dart file where you are going to use them.
import 'package:logger/logger.dart';
3. Creating instance of logger class & Printing on the console
default logger instance
final logger = new Logger();
Custom logger instance
Logger in flutter takes some of the properties that you can customize how your logger message will be displayed on the console screen.
final logger = new Logger( printer: PrettyPrinter( methodCount: 1, lineLength: 50, errorMethodCount: 3, colors: true, printEmojis: true ), );
Then now once your instance of Logger is been created now you can use the instance object name to print log on console, as shown below
logger.v("Verbose Log"); logger.d("Debug Log"); logger.i("Info Log"); logger.w("Warning Log"); logger.e("Error Log"); logger.wtf("What a terriable failure log");
There are various kinds of log message that you can use to print on the console such as Verbose log, Debug Log, Info Log, Warning Log, Error Log, as shown in the above snippet code.
Output – flutter logger example
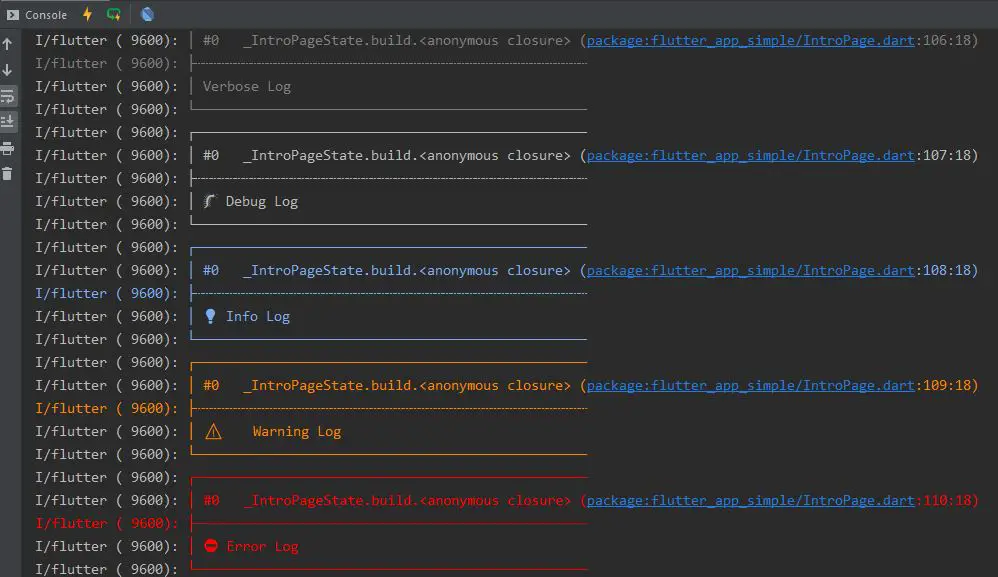