Hi Guys, Welcome to Proto Coders Point, so in this flutter tutorial blog article, will learn about flutter null safety with example.
What is null safety in dart?
A Flutter Null Safety feature is a secured way of developing a flutter application, In any programming language, a variable can have a value assigned to it or might be null.
// Eg: Snippet String name; // is null String firstname = "Rajat Palankar"; //has a value been assigned.
because in dart variable can be null & if a variable is null we cannot perform an action with it like, printing the null value on user screen or we cannot execute at string method to a null value & if we do so this may lead to null pointer exception & your flutter application will crash in run-time.
As shown in below Code and output Screenshot
Example without null safety flutter
#code
// Snippet Flutter Dart code class _MyHomePageState extends State<MyHomePage> { String name; // null value @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Text(name), // trying to print null # run time , null pointer exception ) ); } }
Output example
Here you can see that when i tried to print null in text widget it showing Failed assertion – A non-null string must be provided.
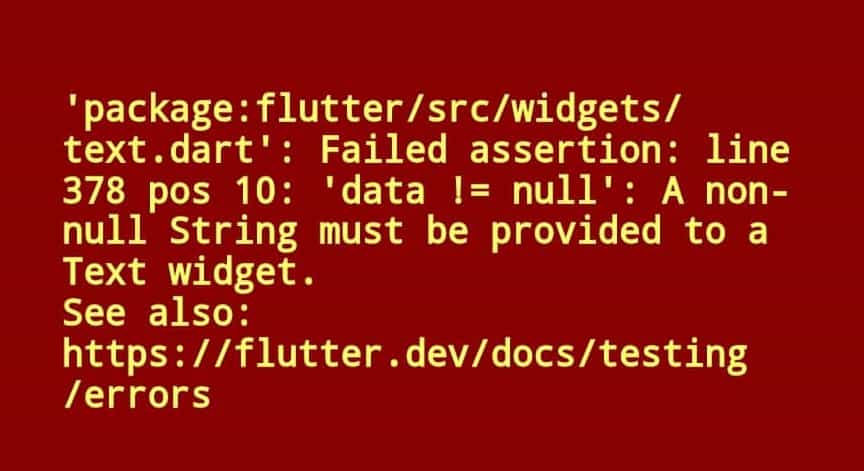
Flutter null safety enable
To solve null pointer exception flutter 2 came with null safety feature.
Set the minimum sdk constrant to 2.12.0 or higher & also check you have updated flutter version(flutter 2)
Then, if you want to use null safety dart then you need to enable null safety to do so, in your flutter project go to
Flutter Project > pubspec.yaml file
change sdk version >= 2.12.0
environment: sdk: ">=2.12.0 <3.0.0"
and run flutter pub get
So now you have successfully enabled null safety in flutter project, now all of our datatype are by default “Not Nullable”
Not nullable means they requires some value to be assigned to them. A String, Int, or any date type must have some value.
Eg: int number = 15; String name = " Proto Coders Point";
What if you want some datatype to be nullable
If in case, you want to make a datatype to be nullable then you need to add a question (?) mark to the datatype.
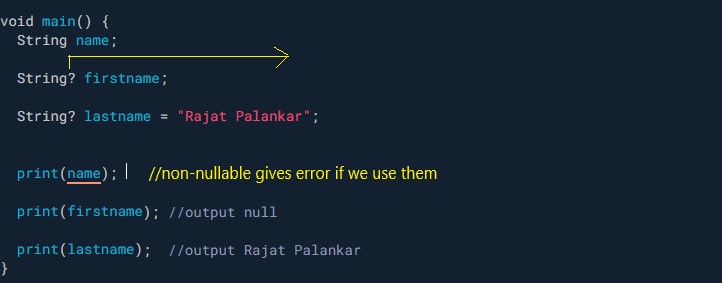
so in the above example, just by adding ‘?’ immediately after the data type, you can make it nullable which means Null is allowed.
Flutter null Safety Examples
- Null Safety Example 1
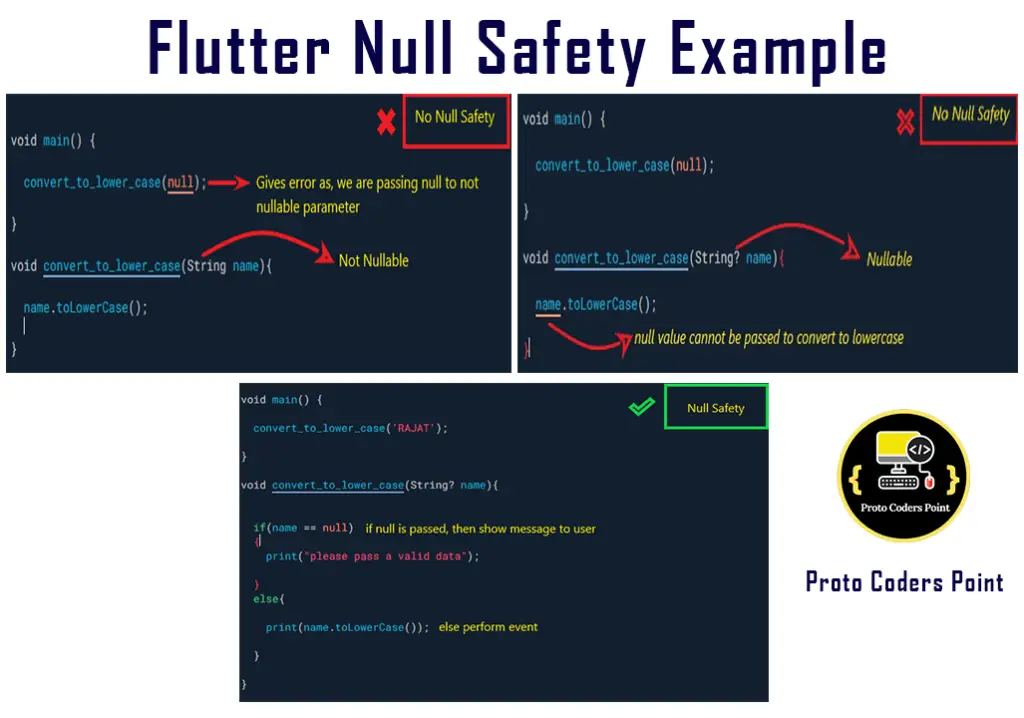
Late Modifier to achieve null safety
Example without late modifier which gives you null safety error.
String name; // null safety error @override void initState() { super.initState(); name = "Rajat Palankar"; }
The above code will give you a null safety compilation error because dart is not clever enough to understand that you have initialized the value to the variable afterwords.
Therefore, to future initialization or late initialization value to variable you can make use of “late modifier”, that tells compiler that value will be initialized later on.
what is late modifier?
In Flutter late keyword will lets you use non-nullable datatype. Now i dart 2.12 has add late modifier, late keyword is used in two case:
- While migrating flutter project to null safety.
- Lazy initializing of a variable.
Late keyword is used just to promise dart compiler that you are going to intialize the value to variable later on in future.
Note: if you don’t full fill as promised using late modifier then your flutte app will crash at runtime.
Late modifier example
late String name; // here I am using late keyword before datatype to tell the compiler that I will initialize the value later @override void initState() { super.initState(); name = "Rajat Palankar"; // as promised initializing is done here print(name); //now i can use the variable }