Hi Guys, Welcome to Proto Coders Point, In this Flutter Tutorial we will Implement Flutter Registration and Login page using Firebase Authentication Flutter.
If you are Creating a new Flutter Project Have you setup Firebase Project in the Console ( Check out Flutter Firebase Project integration Setup )
Flutter Login and Registration Page using Firebase as BackEnd
Straight into point let’s Start implementing Flutter Registration Page
Create a new Flutter project call it flutter login demo
As usual you need to Create a new Flutter Project, I m making user of android-studio as my development Kit.
Open android-studio > New > New Flutter Project > Give a name to your project (Flutter Login Demo)
Create the Simple TextField UI for our Flutter Login Page and Registration Page
This Firebase authentication Flutter tutorial need 2 Screen page one for Registration and other for Login
main.dart
The Below lines of Code is the Registration Page UI
Note: below Source code is just an UI design without any Functionality of Firebase Authentication.
The Fully functional code will be given at the bottom of this tutorial.
import 'package:flutter/material.dart'; import 'package:modal_progress_hud/modal_progress_hud.dart'; import 'login.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { bool showProgress = false; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Firebase Authentication"), ), body: Center( child: ModalProgressHUD( inAsyncCall:showProgress , child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( "Registration Page", style: TextStyle(fontWeight: FontWeight.w800, fontSize: 20.0), ), SizedBox( height: 20.0, ), TextField( keyboardType: TextInputType.emailAddress, textAlign: TextAlign.center, onChanged: (value) {}, decoration: InputDecoration( hintText: "Enter your Email", border: OutlineInputBorder( borderRadius: BorderRadius.all(Radius.circular(32.0)))), ), SizedBox( height: 20.0, ), TextField( obscureText: true, textAlign: TextAlign.center, onChanged: (value) {}, decoration: InputDecoration( hintText: "Enter your Password", border: OutlineInputBorder( borderRadius: BorderRadius.all(Radius.circular(32.0)))), ), SizedBox( height: 20.0, ), Material( elevation: 5, color: Colors.lightBlue, borderRadius: BorderRadius.circular(32.0), child: MaterialButton( onPressed: () { // Navigator.push( // context, // MaterialPageRoute(builder: (context) => MyLoginPage()), // ); }, minWidth: 200.0, height: 45.0, child: Text( "Register", style: TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0), ), ), ) ], ), ), ), ); } }
login.dart
Likewise same the below code is just an UI without any Firebase authentication.
import 'package:flutter/material.dart'; import 'package:modal_progress_hud/modal_progress_hud.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyLoginPage(), ); } } class MyLoginPage extends StatefulWidget { @override _MyLoginPageState createState() => _MyLoginPageState(); } class _MyLoginPageState extends State<MyLoginPage> { bool showProgress = false; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Firebase Authentication"), ), body: Center( child: ModalProgressHUD( inAsyncCall: showProgress, child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( "Login Page", style: TextStyle(fontWeight: FontWeight.w800, fontSize: 20.0), ), SizedBox( height: 20.0, ), TextField( keyboardType: TextInputType.emailAddress, textAlign: TextAlign.center, onChanged: (value) {}, decoration: InputDecoration( hintText: "Enter your Email", border: OutlineInputBorder( borderRadius: BorderRadius.all(Radius.circular(32.0)))), ), SizedBox( height: 20.0, ), TextField( obscureText: true, textAlign: TextAlign.center, onChanged: (value) {}, decoration: InputDecoration( hintText: "Enter your Password", border: OutlineInputBorder( borderRadius: BorderRadius.all(Radius.circular(32.0)))), ), SizedBox( height: 20.0, ), Material( elevation: 5, color: Colors.lightBlue, borderRadius: BorderRadius.circular(32.0), child: MaterialButton( onPressed: () {}, minWidth: 200.0, height: 45.0, child: Text( "Login", style: TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0), ), ), ) ], ), ), ), ); } }
In both the Registration and Login pages we have 2 Flutter TextField and an materialButton, when clicked perform an Registration or Login task.
It also has a Modal Progress HUD flutter library which will show registering or login wait progress indicator when button is click until the authentication process.
Adding Flutter Firebase Dependencies into our project
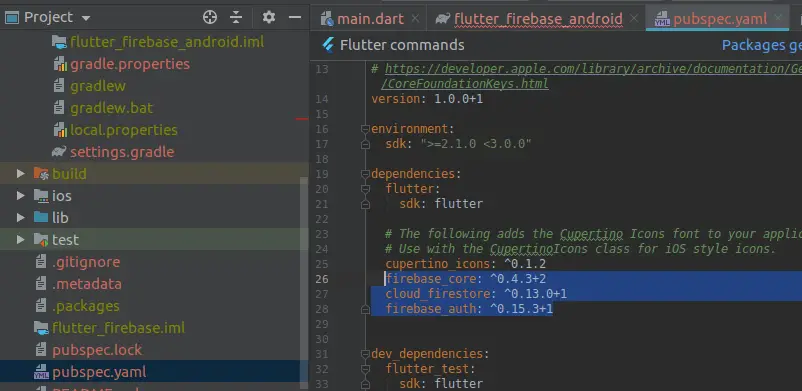
cloud_firestore: ^0.13.0+1 firebase_auth: ^0.15.3+1 firebase_core: ^0.4.3+2 modal_progress_hud: ^0.1.3
Add the required Flutter Firebase dependencies into pubspec.yaml file
Activiting Authentication Sign-In Method in Firebase Console
To make use of Firebase Authentication Free Services, you need to first activate which Sign in Method do you want to user.
In this Tutorial, as you know we are implementing Email, Password Firebase Authentication we need to activate this service.
Go to Firebase Console select your Project that we have created in tutorial “Flutter Firebase Project integration Setup” and Navigate towords Authentication option
Click on Set-up Sign-In method and Then Enable Email/Password method
Then, all set you are ready to make use of Firebase Email/Password Sign-in method
Registration Snippet Code of Flutter Firebase
You need to import Firebase Auth when you want to user it.
import 'package:firebase_auth/firebase_auth.dart';
final _auth = FirebaseAuth.instance; String email, password; try { final newUser = await _auth.createUserWithEmailAndPassword( email: email, password: password); if (newUser != null) { Navigator.push(context, MaterialPageRoute( builder: (context) => MyLoginPage()), ); } } catch (e) { print(e); }
Here we have created an FirebaseAuth instance that can handle creating new users with their email and password. And once user get registered successfully we navigate the user to login Screen.
Login Snippet Code of Flutter Firebase
final _auth = FirebaseAuth.instance; String email, password; try { final newUser = await _auth.signInWithEmailAndPassword( email: email, password: password); if (newUser != null) { //successfully login //navigate the user to main page // i am just showing toast message here } } catch (e) {}
Here we make user of signInWithEmailAndPassword firebase class method to help the user to Log-In into our Flutter Application.
Complete Source Code for Flutter Login and Registration Page using Firebase Authentication
main.dart ( Registration Page )
import 'package:flutter/material.dart'; import 'package:modal_progress_hud/modal_progress_hud.dart'; import 'package:firebase_auth/firebase_auth.dart'; import 'login.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { final _auth = FirebaseAuth.instance; bool showProgress = false; String email, password; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Firebase Authentication"), ), body: Center( child: ModalProgressHUD( inAsyncCall: showProgress, child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( "Registration Page", style: TextStyle(fontWeight: FontWeight.w800, fontSize: 20.0), ), SizedBox( height: 20.0, ), TextField( keyboardType: TextInputType.emailAddress, textAlign: TextAlign.center, onChanged: (value) { email = value; //get the value entered by user. }, decoration: InputDecoration( hintText: "Enter your Email", border: OutlineInputBorder( borderRadius: BorderRadius.all(Radius.circular(32.0)))), ), SizedBox( height: 20.0, ), TextField( obscureText: true, textAlign: TextAlign.center, onChanged: (value) { password = value; //get the value entered by user. }, decoration: InputDecoration( hintText: "Enter your Password", border: OutlineInputBorder( borderRadius: BorderRadius.all(Radius.circular(32.0)))), ), SizedBox( height: 20.0, ), Material( elevation: 5, color: Colors.lightBlue, borderRadius: BorderRadius.circular(32.0), child: MaterialButton( onPressed: () async { setState(() { showProgress = true; }); try { final newuser = await _auth.createUserWithEmailAndPassword( email: email, password: password); if (newuser != null) { Navigator.push( context, MaterialPageRoute( builder: (context) => MyLoginPage()), ); setState(() { showProgress = false; }); } } catch (e) {} }, minWidth: 200.0, height: 45.0, child: Text( "Register", style: TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0), ), ), ), SizedBox( height: 15.0, ), GestureDetector( onTap: () { Navigator.push( context, MaterialPageRoute(builder: (context) => MyLoginPage()), ); }, child: Text( "Already Registred? Login Now", style: TextStyle( color: Colors.blue, fontWeight: FontWeight.w900), ), ) ], ), ), ), ); } }
login.dart ( Login Page )
create a new dart file under your flutter project > lib > login.dart
import 'package:flutter/material.dart'; import 'package:modal_progress_hud/modal_progress_hud.dart'; import 'package:firebase_auth/firebase_auth.dart'; import 'package:fluttertoast/fluttertoast.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyLoginPage(), ); } } class MyLoginPage extends StatefulWidget { @override _MyLoginPageState createState() => _MyLoginPageState(); } class _MyLoginPageState extends State<MyLoginPage> { final _auth = FirebaseAuth.instance; bool showProgress = false; String email, password; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Firebase Authentication"), ), body: Center( child: ModalProgressHUD( inAsyncCall: showProgress, child: Column( mainAxisAlignment: MainAxisAlignment.center, children: <Widget>[ Text( "Login Page", style: TextStyle(fontWeight: FontWeight.w800, fontSize: 20.0), ), SizedBox( height: 20.0, ), TextField( keyboardType: TextInputType.emailAddress, textAlign: TextAlign.center, onChanged: (value) { email = value; // get value from TextField }, decoration: InputDecoration( hintText: "Enter your Email", border: OutlineInputBorder( borderRadius: BorderRadius.all(Radius.circular(32.0)))), ), SizedBox( height: 20.0, ), TextField( obscureText: true, textAlign: TextAlign.center, onChanged: (value) { password = value; //get value from textField }, decoration: InputDecoration( hintText: "Enter your Password", border: OutlineInputBorder( borderRadius: BorderRadius.all(Radius.circular(32.0)))), ), SizedBox( height: 20.0, ), Material( elevation: 5, color: Colors.lightBlue, borderRadius: BorderRadius.circular(32.0), child: MaterialButton( onPressed: () async { setState(() { showProgress = true; }); try { final newUser = await _auth.signInWithEmailAndPassword( email: email, password: password); print(newUser.toString()); if (newUser != null) { Fluttertoast.showToast( msg: "Login Successfull", toastLength: Toast.LENGTH_SHORT, gravity: ToastGravity.CENTER, timeInSecForIos: 1, backgroundColor: Colors.blueAccent, textColor: Colors.white, fontSize: 16.0); setState(() { showProgress = false; }); } } catch (e) {} }, minWidth: 200.0, height: 45.0, child: Text( "Login", style: TextStyle(fontWeight: FontWeight.w500, fontSize: 20.0), ), ), ) ], ), ), ), ); } }
All set you Flutter App is now been integrated with Firebase Authentication Service, your Flutter app can now handle Login and Registration using Firebase services.
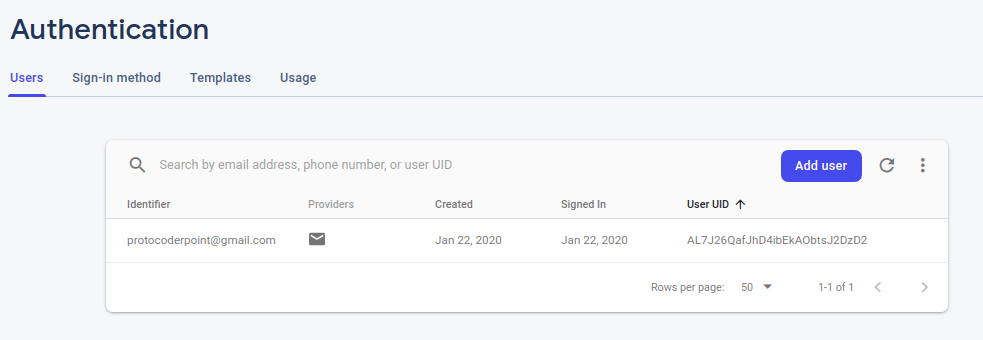