Hi Guys, Welcome to Proto Coders Point, In this flutter article will learn how to implement password strength checker in flutter, That too without using any third party flutter_password_strength library’s.
Flutter Password Strength Checker
In this flutter example, Will follow below criteria to measure flutter password strength & validate:
- Password String length Less than 6 characters : Weak (password not accepted)
- my password Length i.e greater then 6 & less then 8 characters: Medium (password is accepted, but need strong)
- 8 characters or more: Strong.
- 8 characters or more and contains Capital Small letter, number & special character password validation: Great
To check if entered password contain Capital, Small letter, number & special character then you need to use below regular expression
RegExp pass_valid = RegExp(r"(?=.*\d)(?=.*[a-z])(?=.*[A-Z])(?=.*\W)");
Learn more about flutter password validation.
Flutter password validation + password strength checker
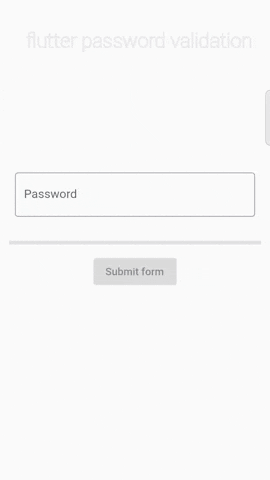
In this password validation + strength check tutorial, user entered password string length should be 8 or more characters, and the string should include Capital, Small, number & a special character.
Then, the strength of entered password will be shown in LinearProgressIndicator widget.
In LinearProgressIndicator:
- red: Weak.
- yellow: Medium.
- blue: Strong
- green: Super Strong
Only if flutter password strength is green then allowed to submit or create account, The submit form button is activiated to click as you can see the above gif example.
Password Validation Function Code Explaination
//A function that validate user entered password bool validatePassword(String pass){ String _password = pass.trim(); if(_password.isEmpty){ setState(() { password_strength = 0; }); }else if(_password.length < 6 ){ setState(() { password_strength = 1 / 4; //string length less then 6 character }); }else if(_password.length < 8){ setState(() { password_strength = 2 / 4; //string length greater then 6 & less then 8 }); }else{ if(pass_valid.hasMatch(_password)){ // regular expression to check password valid or not setState(() { password_strength = 4 / 4; }); return true; }else{ setState(() { password_strength = 3 / 4; }); return false; } } return false; }
Complete Source Code – Flutter Password Validation with strength check
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomepage(), ); } } class MyHomepage extends StatefulWidget { const MyHomepage({Key? key}) : super(key: key); @override State<MyHomepage> createState() => _MyHomepageState(); } class _MyHomepageState extends State<MyHomepage> { final _formKey = GlobalKey<FormState>(); // regular expression to check if string RegExp pass_valid = RegExp(r"(?=.*\d)(?=.*[a-z])(?=.*[A-Z])(?=.*\W)"); double password_strength = 0; // 0: No password // 1/4: Weak // 2/4: Medium // 3/4: Strong // 1: Great //A function that validate user entered password bool validatePassword(String pass){ String _password = pass.trim(); if(_password.isEmpty){ setState(() { password_strength = 0; }); }else if(_password.length < 6 ){ setState(() { password_strength = 1 / 4; }); }else if(_password.length < 8){ setState(() { password_strength = 2 / 4; }); }else{ if(pass_valid.hasMatch(_password)){ setState(() { password_strength = 4 / 4; }); return true; }else{ setState(() { password_strength = 3 / 4; }); return false; } } return false; } @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Form( key: _formKey, child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Padding( padding: const EdgeInsets.all(20.0), child: TextFormField( onChanged: (value){ _formKey.currentState!.validate(); }, validator: (value){ if(value!.isEmpty){ return "Please enter password"; }else{ //call function to check password bool result = validatePassword(value); if(result){ // create account event return null; }else{ return " Password should contain Capital, small letter & Number & Special"; } } }, decoration: InputDecoration(border: OutlineInputBorder(),hintText: "Password"), ), ), Padding( padding: const EdgeInsets.all(12.0), child: LinearProgressIndicator( value: password_strength, backgroundColor: Colors.grey[300], minHeight: 5, color: password_strength <= 1 / 4 ? Colors.red : password_strength == 2 / 4 ? Colors.yellow : password_strength == 3 / 4 ? Colors.blue : Colors.green, ), ), ElevatedButton(onPressed: password_strength != 1 ? null : (){ //perform click event }, child: Text("Submit form")) ], ), ), ), ); } }