Hi Guys, Welcome to Proto Coders Point, In this flutter article let’s learn how to log long string in flutter, How to fix pring statement in flutter that only pring 1020 character & trancate the remaining string & leaves this symbol at end <..>.
Basically, The current version of dart language does not support printing logs that has more then 1020 character, instead print() statement in flutter will only print 1020 character & trancate remaining.
Check below screenshot
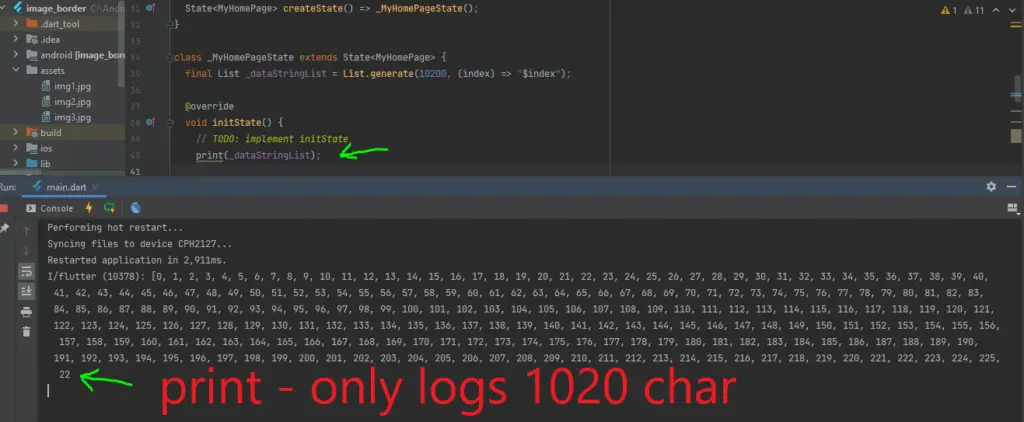
So, Here is a solution on How to Prin full long string/list without missing any data while debugging in flutter.
Print Long String in console While developing flutter app
Here is the right way to print logs message or data on console for debugging your flutter application.
Learn how about flutter logging
The Right way to log data on console is by importing dart inbuilt package “dart:developer”, This allows a developer to print longs information on console while debugging.
So to use developer.log function in flutter app you need to import it.
import 'dart:developer' as logDev;
Now use it to show logs in console like this:
devlog.log(“Your Log Message Here”,name:"myLog");
How to use dart.developer with log function
Video Tutorial
Complete Source Code
main.dart
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'dart:developer' as logDev; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { final List _dataStringList = List.generate(10200, (index) => "$index"); @override void initState() { // TODO: implement initState logDev.log(_dataStringList.toString(),name: "myLog"); super.initState(); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Flutter Log Messages"), ), body:Container() ); } }