Hi Guys, Welcome to Proto Coders Point, In this quick code snippet article, let’s learn different way to reverse a string in python with & without using python inbuild methods.
Different ways to reverse a string in python language
Let’s get Started
Example 1: Reverse a string in using for loop
Below example reverse a string without using inbuilt function in python
str = "ebutuoY no tnioP sredoC otorP ebircsbuS" st ="" for i in str: st = i + st print(st)
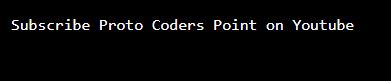
Example 2: Reverse string in Python using an slice
We can easily reverse a string by using python operator called extended slice operator.
Basically slice operator in python accepts 3 parameters (start, stop & step), In below example for start & stop index we pass no value, which will by default start index from 0 and end with n-1, then in step parameter we pass -1, which means string be been slice and traverse from end of given string, up to the 1st index position and thus we have a reversed string.
def rev(s): s = s[::-1] return s s = "Proto Coders Point" print("The original string : ",s) print("The reversed string : ",rev(s))

Example 3: python code to reverse a string using join & reversed function
Here we are using python reversed function that is an inbuild method using which we can reverse the given string
def rev(str): rev_str = "". join(reversed(str)) return rev_str s = "Proto Coders Point" print ("The original string : ",s) print ("The reversed string using join & reversed func : ",rev(s))
