Hi Guys welcome to Proto Coders Point In this Flutter Tutorial we gonna implement Flutter Refresh Indicator that help app user to refresh the data by just pull down to refresh listview.
Brief about Refresh Indicator
The refresh indicator will be the parent of its child or children, The progress loading indicator will appear when a user will scroll descentdant is over-scrolled. An animated circular progress indicator is faded into view.
When the user swipe down to refresh the data, the onRefresh callback property is been called.The callback is expected to update the scrollable’s contents and then complete the Future it returns.
I have already implemented this feature in android application.
Check out Swipe Refresh Layout – Android Pull down to refresh widget
Let’s begin implementing Refresh Indicator in our Flutter Project.
Flutter Refresh Indicator – A pull to refresh listview with example
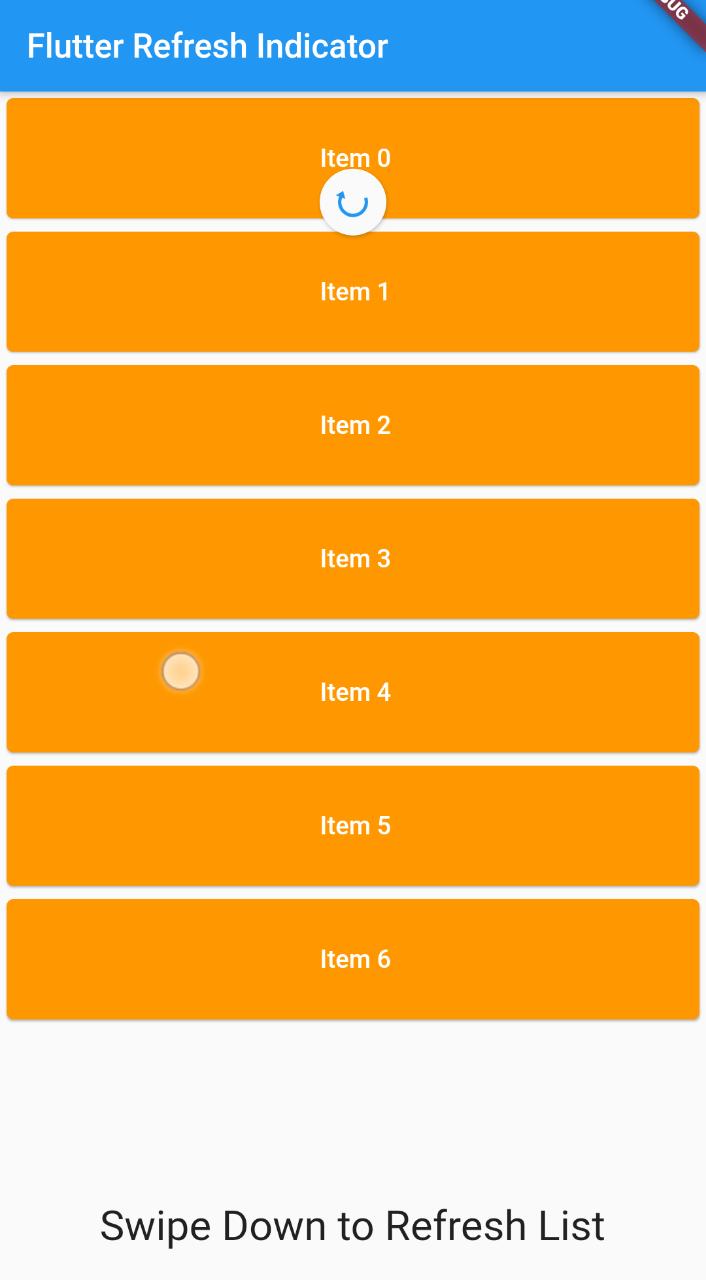
Create new Flutter project
offcourse you need to create a new flutter project or just open any existing project, I am using android-studio to implement this widget.
File > New > New Flutter Project
Remove all the Default code that comes, when you create new Flutter project.
Just Copy paste below code in main.dart
The below code is just to get ride of default code generated by flutter.
Find the complete code of RefreshIndicator below at the end of this tutorial.
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Flutter Refresh Indicator"), ), body: Container(), ); } }
Import async & math package
import 'dart:async'; import 'dart:math';
we are making use of async task and math library.
async package is used so that we can use Future,await and async function.
Math package is used to make use of Random method to generate random numbers.
Variable and method we gonna use
var refreshkey = GlobalKey<RefreshIndicatorState>(); var list; var random;
GlobalKey : A key that is unique across the entire app. learn more
list : a list that holds array of list data.
random : which holds random number 0 – 10 using Random() method.
initState
This will be called only once when app get open
@override void initState() { super.initState(); random = Random(); refreshlist(); }
refreshlist() is an Future async function that refresh the list data, we need to user Future function because onRefresh property of RefreshIndicator accept a Future.
Future<Null> refreshlist() async { refreshkey.currentState?.show( atTop: true); // change atTop to false to show progress indicator at bottom await Future.delayed(Duration(seconds: 2)); //wait here for 2 second setState(() { list = List.generate(random.nextInt(10), (i) => " Item $i"); }); }
Here when user pull down to refresh the list is been re-generate again with new data.
UI Design snippet code
In the below code we have RefreshIndicator as a parent of ListView.builder
SizedBox which has it child as Card() where card color is orange,
This card() Widget has child as Text() widget to show text inside the cards.
Scaffold( appBar: AppBar( title: Text("Flutter Refresh Indicator"), ), body: Column( children: <Widget>[ Expanded( child: RefreshIndicator( key: refreshkey, child: ListView.builder( itemCount: list?.length, itemBuilder: (context, i) => SizedBox( height: 80.0, child: Card( color: Colors.orange, child: Center( child: Text( list[i], style: TextStyle( fontSize: 15.0, color: Colors.white, fontWeight: FontWeight.w600), )), ), ), ), onRefresh: refreshlist, ), ), Text( "Swipe Down to Refresh List", style: TextStyle(fontSize: 25.0), ), SizedBox( height: 25.0, ) ], ), );
Complete Code of Refresh Indicator flutter with example
Copy paste the Below code in main.dart file
main.dart
import 'package:flutter/material.dart'; import 'dart:async'; import 'dart:math'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { var refreshkey = GlobalKey<RefreshIndicatorState>(); var list; var random; @override void initState() { super.initState(); random = Random(); list = List.generate(random.nextInt(10), (i) => " Item $i"); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Flutter Refresh Indicator"), ), body: Column( children: <Widget>[ Expanded( //Expanded is used so that all the widget get fit into the available screen child: RefreshIndicator( key: refreshkey, child: ListView.builder( itemCount: list?.length, itemBuilder: (context, i) => SizedBox( height: 80.0, child: Card( color: Colors.orange, child: Center( child: Text( list[i], // array list style: TextStyle( fontSize: 15.0, color: Colors.white, fontWeight: FontWeight.w600), )), ), ), ), onRefresh: refreshlist, //refreshlist function is called when user pull down to refresh ), ), Text( "Swipe Down to Refresh List", style: TextStyle(fontSize: 25.0), ), SizedBox( height: 25.0, ) ], ), ); } Future<Null> refreshlist() async { refreshkey.currentState?.show( atTop: true); // change atTop to false to show progress indicator at bottom await Future.delayed(Duration(seconds: 2)); //wait here for 2 second setState(() { list = List.generate(random.nextInt(10), (i) => " Item $i"); }); } }
Comments are closed.