Hi Guy’s, Welcome to Proto Coders Point. In this Flutter Article let’s learn how to apply typewritter typing animation effect to flutter text widget.
The Typewriter text effect is an animation where a text is been typed automatically letter by letter, and it feels like the text is been typed one by one.
This kind of animation effect on text is used when you want to grab attention on your app user and make them focus on the Text that is appearing on the screen.
Video Tutorial
Flutter TypeWritter Text Effect – Source Code
import 'package:flutter/material.dart'; void main() => runApp(const MyApp()); class MyApp extends StatelessWidget { const MyApp({super.key}); @override Widget build(BuildContext context) { return MaterialApp( // Remove the debug banner debugShowCheckedModeBanner: false, title: 'Proto Coders Point', theme: ThemeData( primarySwatch: Colors.blue, ), home: const TypeWritterText(), ); } } class TypeWritterText extends StatefulWidget { const TypeWritterText({Key? key}) : super(key: key); @override State<TypeWritterText> createState() => _TypeWritterTextState(); } class _TypeWritterTextState extends State<TypeWritterText> { int _currentIndex = 0; int _currentCharIndex = 0; final List<String> _strings = [ "Welcome to ProtoCodersPoint.com", "Get Programming Solution Here", "And more...", ]; void _typeWrittingAnimation(){ if(_currentCharIndex < _strings[_currentIndex].length){ _currentCharIndex++; }else{ _currentIndex = (_currentIndex+1)% _strings.length; _currentCharIndex = 0; } setState(() { }); Future.delayed(const Duration(milliseconds: 50),(){ _typeWrittingAnimation(); }); } @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: const Text("Proto Coders Point"), ), body: Center( child: Container( padding: const EdgeInsets.all(20), width: double.infinity, child: Align( alignment: Alignment.centerLeft, child: Text( _strings[_currentIndex].substring(0,_currentCharIndex), style: TextStyle( fontSize: 24, fontStyle: FontStyle.italic, fontWeight: FontWeight.bold), ), ), ), ), floatingActionButton: FloatingActionButton( onPressed: _typeWrittingAnimation, child: const Icon(Icons.play_arrow), )); } }
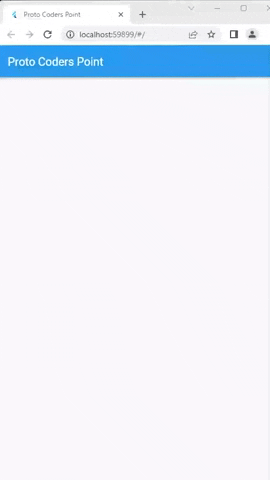
The logic to make text to be typed letter by letter on the screen is by using substring() method.
Here we have a function _typeWrittingAnimation, that keeps on calling itself after every milliseconds say 100 milliseconds, We have 2 integer variable _currentIndex & _currentCharIndex. The _currentCharIndex gets incremented by 1 until it is not end of the string, once the currentCharIndex reaches the end of the string,The _currentIndex is set to next List Item String in the given list.