Hi Guys, Welcome to Proto Coders Point.
In this flutter tutorial, we will learn how to give hover effect in flutter web.
Note: Hover effect in flutter can only be implemented on flutter web app, therefore onhover in mobile device will not give any hovering effect.
Video Tutorial
so let’s begin with the coding.
How to give hover effect to any widget in flutter
1. Create a flutter project
Open you favorite IDE & create/open a new flutter project, I am using android-studio to build flutter projects.
File > New > New Flutter Project ( Give a good name to flutter project & finish) your project will get created.
2. Create a dart file OnHover.dart
Under lib directory, create a new dart file & name it as OnHover.dart.
So OnHover.dart will be our custom stateful widget which has properties such as animatedContainer & MouseRegion widget which is use to detect when our mouse pointer comes in MouseRegion to perform hover effect in flutter with animation to any widgets.
OnHover.dart
import 'package:flutter/material.dart'; class OnHover extends StatefulWidget { final Widget Function(bool isHovered) builder; const OnHover({Key? key, required this.builder}) : super(key: key); @override _OnHoverState createState() => _OnHoverState(); } class _OnHoverState extends State<OnHover> { bool isHovered = false; @override Widget build(BuildContext context) { final hovered = Matrix4.identity()..translate(0,-10,0); final transform = isHovered ? hovered : Matrix4.identity(); return MouseRegion( onEnter: (_)=> onEntered(true), onExit: (_)=> onEntered(false), child: AnimatedContainer( duration: Duration(milliseconds: 300), transform: transform, child: widget.builder(isHovered), ), ); } void onEntered(bool isHovered){ setState(() { this.isHovered = isHovered; }); } }
3. Add Hover effect to any widget in flutter
Now, our OnHover custom widget is ready(above), so now we can use it to give animation & hover effect to any widget in flutter.
To do that you need to simply wrap any widget with OnHover widget builder to apply Hovering effect.
Examples:
Flutter text animation on hover – change text color
OnHover(builder: (isHovered){ return Text("Flutter Text on hover - change text color on hovering with animation"); }),
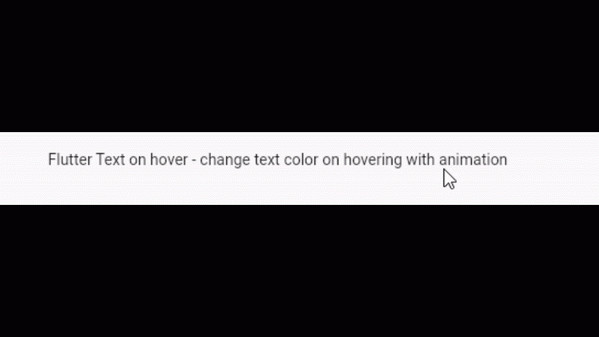
Change Container Color on Hover
OnHover(builder: (isHovered){ final color = isHovered ? Colors.grey : Colors.blue; return Container( color: color, width: 100, height: 100, ); }),
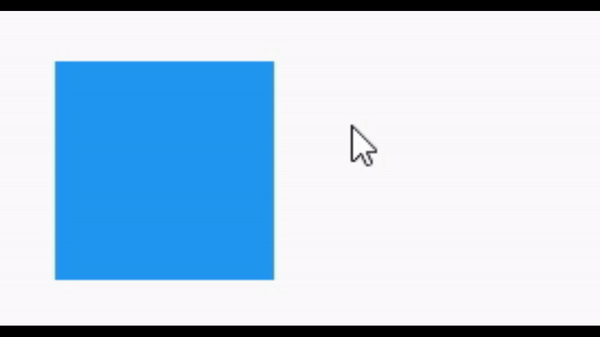
Hover elevation flutter
OnHover(builder: (isHovered){ return PhysicalModel( color: Colors.blue, elevation: isHovered ? 16 : 0, // if ishovered true then show elevation on hovering child: Container( width: 100, height: 100, ), ); }),
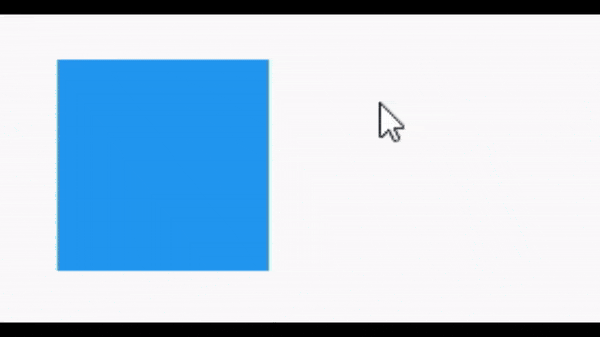
change flutter button color on hover
OnHover(builder: (isHovered){ final color = isHovered ? Colors.red : Colors.blue; return ElevatedButton( style: ElevatedButton.styleFrom(primary: color), onPressed: (){}, child: Text('Change Button Color on hover') ); }),
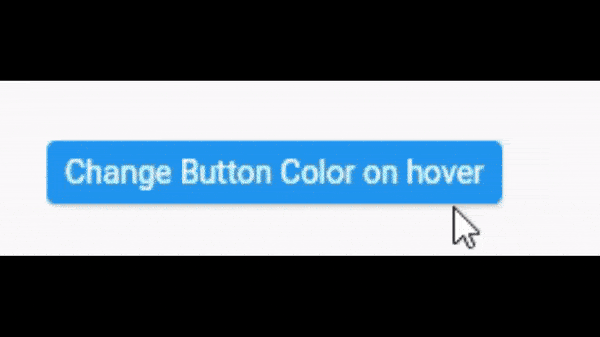
main.dart complete code
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'package:flutter_hover_eg/OnHover.dart'; import 'package:flutter_hover_eg/text_page.dart'; void main() { runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Hover Example"), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ OnHover( builder: (isHovered) { final color = isHovered ? Colors.orange : Colors.redAccent; return PhysicalModel( color: Colors.white, elevation: isHovered ? 16 : 0, child: Container( height: 50, width: 200, color: color, child: Center( child: Text("Proto Coders Point"), )), ); }, ), SizedBox( height: 30, ), OnHover(builder: (isHovered) { return Text( "Flutter Text on hover - change text color on hovering with animation"); }), SizedBox( height: 30, ), //if isHovered is true then show elevation else set elevation to 0 OnHover(builder: (isHovered) { return PhysicalModel( color: Colors.blue, elevation: isHovered ? 16 : 0, child: Container( width: 100, height: 100, ), ); }), OnHover(builder: (isHovered) { final color = isHovered ? Colors.grey : Colors.blue; return Container( color: color, width: 100, height: 100, ); }), SizedBox( height: 30, ), OnHover(builder: (isHovered) { final color = isHovered ? Colors.red : Colors.blue; return ElevatedButton( style: ElevatedButton.styleFrom(primary: color), onPressed: () {}, child: Text('Change Button Color on hover')); }), ], ), ), ); } }