Hi Guys, Welcome to Proto Coders Point, In this Android Tutorial we will create an app that can support multiple languages, In other words, the user can select his desired language to change the whole app language in android.
This is a Simple Example of how to make an android multi language app with an example – locale in android.
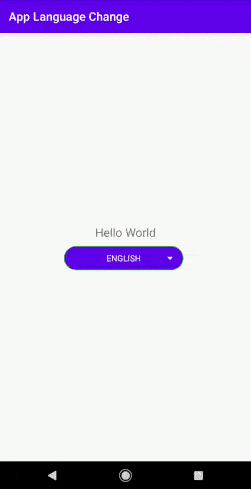
Video Tutorial on change whole app language android programmatically
Step 1 − Create a new Android Project in Android Studio
Create a new project in Android Studio, go to File ⇒ New Project and fill all required details to create a new project. ( Name it as Change App language)
Step 2 – Create a new strings.xml with locale
In the values directory, you need to create a separate string.xml(hi) or any other language
Here is how to create a locale in android string with a language
right-click on values directory => New => Values Resource File
The below dialog will pop up in the android studio
Here you need to select your locale languages that your app will support.
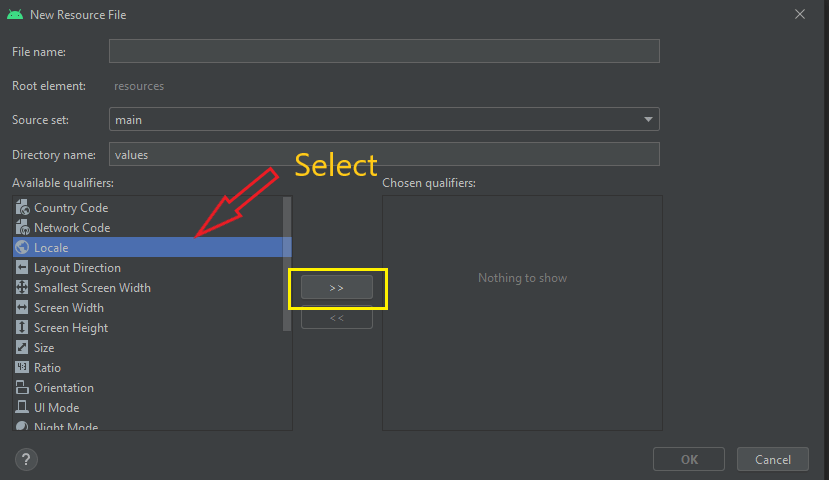
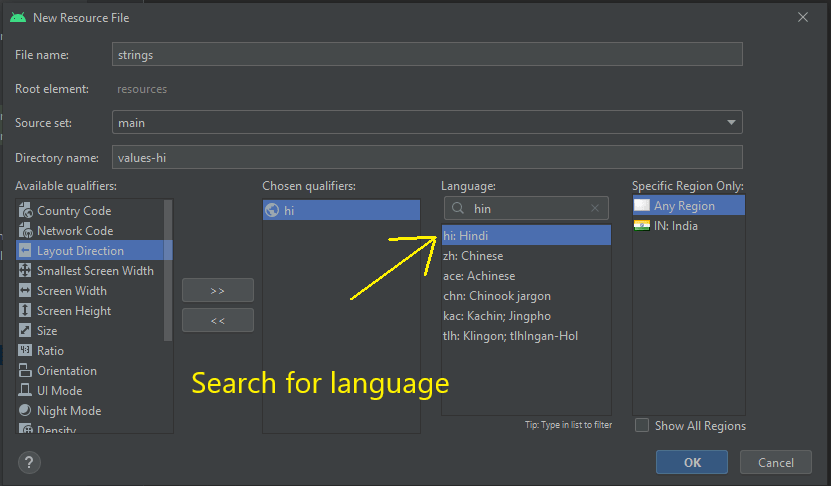
After creating saperate strings.xml files for particular languages your string directory will look something like this.
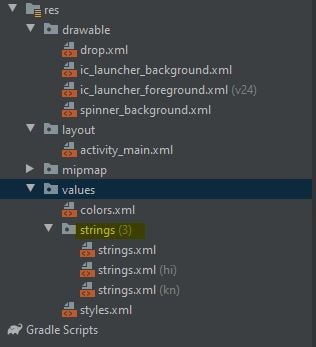
Now in those strings.xml file add the translated strings
string.xml (hi) for hindi language in your android app.
<?xml version="1.0" encoding="utf-8"?> <resources> <string name="app_name">ऐप भाषा बदलें</string> <string name="language">नमस्ते दुनिया</string> </resources>
string.xml (kn) for Kannada language in your app.
<?xml version="1.0" encoding="utf-8"?> <resources> <string name="app_name">ಅಪ್ಲಿಕೇಶನ್ ಭಾಷೆ</string> <string name="language">ಹಲೋ ವರ್ಲ್ಡ್</string> </resources>
likewise you can add different language that you want to add into your android applicaton.
Step 3 – Create a LocaleHelper Class
Now create new java file and name it as Localehelper and add the below code
This class will help you in getting and storing language that a user has previously selected before he close the application, we will use SharedPreferences to store the locale selected by user in app.
package com.example.applanguagechange.Language; import android.annotation.TargetApi; import android.content.Context; import android.content.SharedPreferences; import android.content.res.Configuration; import android.content.res.Resources; import android.os.Build; import android.preference.PreferenceManager; import java.util.Locale; /** * Created by abdalla on 10/2/17. */ public class LocaleHelper { private static final String SELECTED_LANGUAGE = "Locale.Helper.Selected.Language"; public static Context onAttach(Context context) { String lang = getPersistedData(context, Locale.getDefault().getLanguage()); return setLocale(context, lang); } public static Context onAttach(Context context, String defaultLanguage) { String lang = getPersistedData(context, defaultLanguage); return setLocale(context, lang); } public static String getLanguage(Context context) { return getPersistedData(context, Locale.getDefault().getLanguage()); } public static Context setLocale(Context context, String language) { persist(context, language); if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.N) { return updateResources(context, language); } return updateResourcesLegacy(context, language); } private static String getPersistedData(Context context, String defaultLanguage) { SharedPreferences preferences = PreferenceManager.getDefaultSharedPreferences(context); return preferences.getString(SELECTED_LANGUAGE, defaultLanguage); } private static void persist(Context context, String language) { SharedPreferences preferences = PreferenceManager.getDefaultSharedPreferences(context); SharedPreferences.Editor editor = preferences.edit(); editor.putString(SELECTED_LANGUAGE, language); editor.apply(); } @TargetApi(Build.VERSION_CODES.N) private static Context updateResources(Context context, String language) { Locale locale = new Locale(language); Locale.setDefault(locale); Configuration configuration = context.getResources().getConfiguration(); configuration.setLocale(locale); configuration.setLayoutDirection(locale); return context.createConfigurationContext(configuration); } @SuppressWarnings("deprecation") private static Context updateResourcesLegacy(Context context, String language) { Locale locale = new Locale(language); Locale.setDefault(locale); Resources resources = context.getResources(); Configuration configuration = resources.getConfiguration(); configuration.locale = locale; if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN_MR1) { configuration.setLayoutDirection(locale); } resources.updateConfiguration(configuration, resources.getDisplayMetrics()); return context; } }
Step 4 – UI Design
activity_main.xml
Add the following code to res/layout/activity_main.xml.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity" android:layout_gravity="center" android:gravity="center" android:orientation="vertical"> <TextView android:id="@+id/helloworld" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" android:textSize="20sp" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" /> <RelativeLayout android:id="@+id/showlangdialog" android:layout_width="200dp" android:layout_height="40dp" android:layout_marginTop="10dp" android:layout_marginEnd="3dp" android:layout_marginRight="3dp" android:background="@drawable/background_color" android:orientation="horizontal"> <TextView android:id="@+id/dialog_language" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_alignParentBottom="true" android:layout_gravity="center" android:gravity="center" android:background="@android:color/transparent" android:dropDownVerticalOffset="35dp" android:popupBackground="@color/colorPrimary" android:textColor="@color/white" android:text="English" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentRight="true" android:layout_centerVertical="true" android:layout_gravity="center" android:layout_marginRight="10dp" android:src="@drawable/drop" /> </RelativeLayout> </LinearLayout>
@drawable/spinner_background.xml
create a new drawable resource file under drawable folder and add the below code.
This code is just to give background to above relativelayout
<shape xmlns:android="http://schemas.android.com/apk/res/android"> <solid android:color="@color/colorPrimary" /> <corners android:radius="40dp" /> <stroke android:width="1dp" android:color="#05AC21" /> </shape>
Create a vector drop image in drawable folder
Right Click(drawable) > New > Vector Image ( select a arrow down vector image )
Step 5 – Java Code to switch between string.xml to use
Main_Activity.java
package com.example.applanguagechange; import androidx.appcompat.app.AlertDialog; import androidx.appcompat.app.AppCompatActivity; import android.content.Context; import android.content.DialogInterface; import android.content.res.Resources; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.RelativeLayout; import android.widget.TextView; public class MainActivity extends AppCompatActivity { TextView helloworld,dialog_language; int lang_selected; RelativeLayout show_lan_dialog; Context context; Resources resources; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); dialog_language = (TextView)findViewById(R.id.dialog_language); helloworld =(TextView)findViewById(R.id.helloworld); show_lan_dialog = (RelativeLayout)findViewById(R.id.showlangdialog); if(LocaleHelper.getLanguage(MainActivity.this).equalsIgnoreCase("en")) { context = LocaleHelper.setLocale(MainActivity.this,"en"); resources =context.getResources(); dialog_language.setText("ENGLISH"); helloworld.setText(resources.getString(R.string.hello_world)); setTitle(resources.getString(R.string.app_name)); lang_selected = 0; }else if(LocaleHelper.getLanguage(MainActivity.this).equalsIgnoreCase("hi")){ context = LocaleHelper.setLocale(MainActivity.this,"hi"); resources =context.getResources(); dialog_language.setText("हिन्दी"); helloworld.setText(resources.getString(R.string.hello_world)); setTitle(resources.getString(R.string.app_name)); lang_selected =1; } else if(LocaleHelper.getLanguage(MainActivity.this).equalsIgnoreCase("kn")){ context = LocaleHelper.setLocale(MainActivity.this,"kn"); resources =context.getResources(); dialog_language.setText("ಕನ್ನಡ"); helloworld.setText(resources.getString(R.string.hello_world)); setTitle(resources.getString(R.string.app_name)); lang_selected =2; } show_lan_dialog.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { final String[] Language = {"ENGLISH","हिन्दी","ಕನ್ನಡ"}; final int checkItem; Log.d("Clicked","Clicked"); final AlertDialog.Builder dialogBuilder = new AlertDialog.Builder(MainActivity.this); dialogBuilder.setTitle("Select a Language") .setSingleChoiceItems(Language, lang_selected, new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { dialog_language.setText(Language[i]); if(Language[i].equals("ENGLISH")){ context = LocaleHelper.setLocale(MainActivity.this,"en"); resources =context.getResources(); lang_selected = 0; helloworld.setText(resources.getString(R.string.hello_world)); setTitle(resources.getString(R.string.app_name)); } if(Language[i].equals("हिन्दी")) { context = LocaleHelper.setLocale(MainActivity.this,"hi"); resources =context.getResources(); lang_selected = 1; helloworld.setText(resources.getString(R.string.hello_world)); setTitle(resources.getString(R.string.app_name)); } if(Language[i].equals("ಕನ್ನಡ")) { context = LocaleHelper.setLocale(MainActivity.this,"kn"); resources =context.getResources(); lang_selected = 2; helloworld.setText(resources.getString(R.string.hello_world)); setTitle(resources.getString(R.string.app_name)); } } }) .setPositiveButton("OK", new DialogInterface.OnClickListener() { @Override public void onClick(DialogInterface dialogInterface, int i) { dialogInterface.dismiss(); } }); dialogBuilder.create().show(); } }); } }
Here in above code we have a RelativeLayout with OnClickEvent, Then user will click the RelativeLayout a AlertDialog will popup asking for language selection, by using which user can change language of his application.
For Example: When user select language as Hindi, The strings.xml (hi) will get loaded and all the text in the application will turn it language string that come from strings.xml (hi), likewise if user select kannada as his app language then strings gets loaded from strings.xml(kn).
and if he select language as English all language will come back to default string.xml
Conclusion
In this tutorial we learnt how to change whole app language in android programmatically using strings.xml locale.