Hi Guys, Welcome to Proto Coders Point. In this flutter tutorial, will learn how to create a search bar in fluttter appbar.
A search field i.e. a search bar is the must required feature to be provided to your flutter app user.
If you are android developer you might be knowing about searchview, searchbar in React Native & UISearchBar in iOS.
In flutter we have a searchDelegate class to implement searchfield in flutter.
How to add search bar in appbar flutter
In this tutorial, will add a search bar in flutter appbar, where a user can enter their search query & depending on query entered we can suggest quick search list is search bar.
Will make use of flutter showSearch + searchDelegate class
Let’s get started
After creating or opening flutter project, let’s add a search bar field in flutter appbar.
Scaffold( appBar: AppBar( title: Text("Search Bar"), actions: [ IconButton(onPressed: (){ // class search delegate here }, icon: const Icon(Icons.search)) ], ), );
Here in AppBar, I make use of actions property with has IconButton to show a search magnifying glass icon in appbar.
Flutter show search in Appbar
Now, When user click on search Icon, we must show a search TextField where user can enter his query to make a quick search.
Will use showSearch Delegate class.
showSearch is a widget that shows full screen search page and returns the search query result to the user
AppBar( title: Text("Search Bar"), actions: [ IconButton(onPressed: (){ showSearch(context: context, delegate: MySearch()); }, icon: const Icon(Icons.search)) ], ),
Here MySearch is a class name that we will called to perform search operation in flutter.
Create class that extends SearchDelegate
Search Delegate class extends 4 override methods, create then by clicking on warning suggestion.
The override widget methods are:-
1. buildLeading:-
@override Widget? buildLeading(BuildContext context) => IconButton( icon: const Icon(Icons.arrow_back), onPressed: () { close(context, null) ; }, );
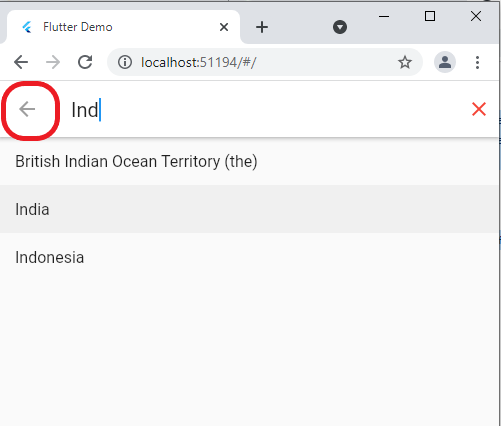
buildLeading is used to show a backbutton in search bar, so that user can click on it to close search box page, Here arrow_back icon is used as you can see in screenshot above.
2. buildAction:–
@override List<Widget>? buildActions(BuildContext context) => [ IconButton( icon: const Icon(Icons.clear,color: Colors.red,), onPressed: () { if(query.isEmpty){ close(context, null); }else{ query = ''; } }, ), ];
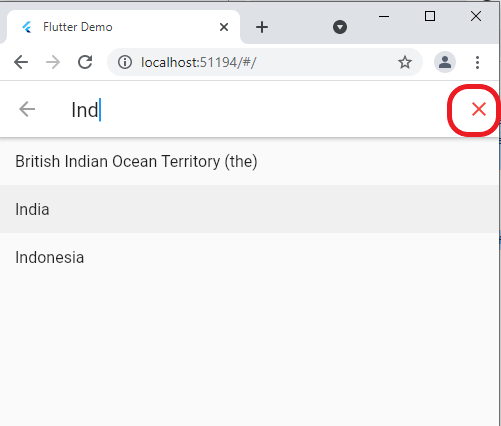
buildAction is used to add some Action widgte in search bar so that use can click on it to perform some action, In out case will use cancel icon so that user can click & cancel it and close search field.
3. buildSuggestions:- flutter search bar with listview
@override Widget buildSuggestions(BuildContext context) { List<String> suggesstions = data.where((element) { final result = element.toLowerCase(); final input = query.toLowerCase(); return result.contains(input); }).toList(); return ListView.builder( itemCount: suggesstions.length, itemBuilder: (context,index){ final suggestion = suggesstions[index]; return ListTile( title: Text(suggestion), onTap: (){ query = suggestion; showResults(context); }, ); } ); }
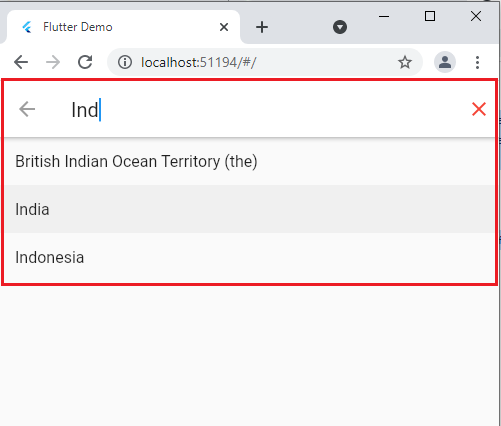
buildSuggestion is used to give a quick suggestion for a search, Here as the user goes on typing user will get hints for his search query, so user can directly select from search box suggestion list as you can see in above screenshot.
buildResults:–
@override Widget buildResults(BuildContext context) => Center( child: Text( query, // query will hold user selected search query ), );
The result that is finalized after the user make his search query final submission & will be shown in search page as search result.
Complete Code of Search Delegate class
class MySearch extends SearchDelegate{ List<String> data = [ 'A','B','C','D','E','F','G','H','I','J' ]; @override List<Widget>? buildActions(BuildContext context) => [ IconButton( icon: const Icon(Icons.clear,color: Colors.red,), onPressed: () { if(query.isEmpty){ close(context, null); }else{ query = ''; } }, ), ]; @override Widget? buildLeading(BuildContext context) => IconButton( icon: const Icon(Icons.arrow_back), onPressed: () { close(context, null) ; }, ); @override Widget buildResults(BuildContext context) => Center( child: Text( query, ), ); //flutter search bar with listview @override Widget buildSuggestions(BuildContext context) { List<String> suggesstions = data.where((element) { final result = element.toLowerCase(); final input = query.toLowerCase(); return result.contains(input); }).toList(); return ListView.builder( itemCount: suggesstions.length, itemBuilder: (context,index){ final suggestion = suggesstions[index]; return ListTile( title: Text(suggestion), onTap: (){ query = suggestion; showResults(context); }, ); } ); } }
Complete source – Flutter add search bar in appbar
main.dart
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', debugShowCheckedModeBanner: false, theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Search Bar"), actions: [ IconButton(onPressed: (){ showSearch(context: context, delegate: MySearch()); }, icon: const Icon(Icons.search)) ], ), ); } } class MySearch extends SearchDelegate{ // this is manual list of query for suggesting user. List<String> data = [ 'A','B','C','D','E','F','G','H','I','J' ]; @override List<Widget>? buildActions(BuildContext context) => [ IconButton( icon: const Icon(Icons.clear,color: Colors.red,), onPressed: () { if(query.isEmpty){ close(context, null); }else{ query = ''; } }, ), ]; @override Widget? buildLeading(BuildContext context) => IconButton( icon: const Icon(Icons.arrow_back), onPressed: () { close(context, null) ; }, ); @override Widget buildResults(BuildContext context) => Center( child: Text( query, ), ); @override Widget buildSuggestions(BuildContext context) { // will show a query hint suggestion "flutter search bar with listview" List<String> suggesstions = data.where((element) { final result = element.toLowerCase(); final input = query.toLowerCase(); return result.contains(input); }).toList(); return ListView.builder( itemCount: suggesstions.length, itemBuilder: (context,index){ final suggestion = suggesstions[index]; return ListTile( title: Text(suggestion), onTap: (){ query = suggestion; showResults(context); }, ); } ); } }