Hi Guys, Welcome to Proto Coders Point. In this flutter tutorial, will learn how to implement range slider in bottom sheet of flutter app.
Flutter Slider
In fluttter a slider is a widget of material design that is used to set a range value, So basically slider widget in flutter is used as input widget by which user can simply drag the slider point to select the range value.
I have already wrote a complete article on Slider flutter where i have explained it in details
Range Slider in flutter Bottom Sheet
This article is only on “How to use range slider in bottom sheet flutter”.
Video Tutorial
Complete Code: main.dart
import 'package:flutter/material.dart'; void main() { runApp(const MyApp()); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', debugShowCheckedModeBanner: false, theme: ThemeData( primarySwatch: Colors.blue, ), home: const MyHomePage(), ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { double height = 180; @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Bottom Sheet with slider"), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text("Height: ${height}", style: TextStyle(fontSize: 30),), ElevatedButton( onPressed: (){ showModalBottomSheet(context: context, builder: (BuildContext context){ return Container( height: 200, child: Center(child: StatefulBuilder( builder: (context,state){ return Center( child: Slider( value: height.toDouble(), max: 200, min: 50, onChanged: (double value) { state(() { height = value.round() as double; }); setState(() { }); }, ), ); }, ), ), ); } ); }, child: Text(" Select Height "), ), ], ), ), ); } }
Output
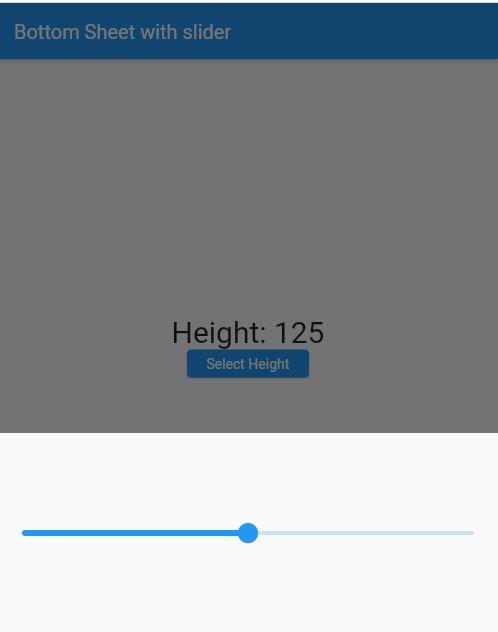
In above code, we have 2 widget Text & Elevated.
Text Widget will simple show there ranger value from range slider.
Elevated Button when pressed, will show model bottom sheet flutter.
Inside showModelBottomSheet popup, we are showing a slider where user can select the range. The same selected range value is show in Text Widget.
Flutter slider not moving / updating
Flutter show model bottom sheet is not a stateful widget that is the reason the widget inside it will not update, In our case slider is not moving even after setState() is called from bottom sheet will not rebuild.
Here is the solution
As you can see in above code, Slider widget is been wrapped with StatefulBuilder Widegt, that will have it own setState() to update/rebuild it child on change.
StatefulBuilder( builder: (context,state){ return Center( child: Slider( value: height.toDouble(), max: 200, min: 50, onChanged: (double value) { // rebuild this statefulbuilder state(() { height = value.round() as double; }); setState(() { // this will rebuild the main }); }, ), ); }, ),