Hi Guy’s Welcome to Proto Coders Point. In this flutter Article let’s learn how to add border to images in flutter.
In this Article will cover 4 example:
- Flutter image with Border all side.
- Flutter Image with rounded/circular borders
- Flutter Image with one side border
- Different border color at each side
As you all know to display Image in flutter we make use of Image Widget, But Flutter Image widget do not provide any properties to set a border to a image. To achieve border to any widget we can use Container widget in which we use decoration properties to set border.
Video Tutorial
Giving Border to Image in flutter
Example:
Container( decoration: BoxDecoration( // add border border: Border.all(/* widget, color,..etc */), // add image child: Image.asset(/* ..path to image. */), ),
In Detail Complete Code Example below.
Flutter Image with Border on all side – Example 1
This Example will create a Border around Image widget by using container, Inside container widget I have used decoration properties with BoxDecoration which has border property and then set Border on all the sides on child widget.
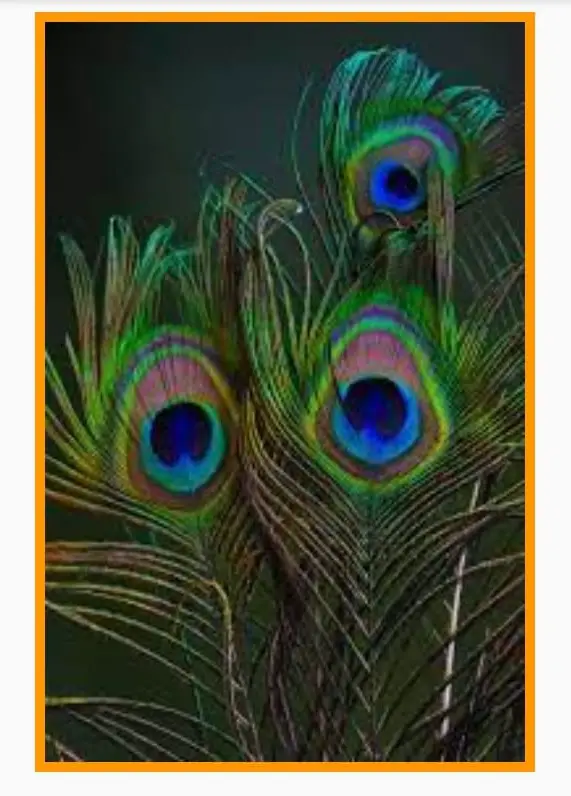
Source Code
body: SingleChildScrollView( child: Center( child: Column( children: [ SizedBox(height: 10,), Container( width: 150, height: 220, decoration: BoxDecoration( border: Border.all(width: 5,color: Colors.orange) ), child: Image.asset('assets/img1.jpg',fit: BoxFit.fill,) ), ], ), ), ),
Flutter image with rounded border or circular borders
The Below Example will clip the edge of a image, To do so we make use of ClipRRect widget with borderRadius property.
ClipRRect( borderRadius: BorderRadius.circular(/*..20.*/), child: Image.asset(/* ... */), ),
Then to add border color, Container widget is been used with decoration as BoxDecoration.
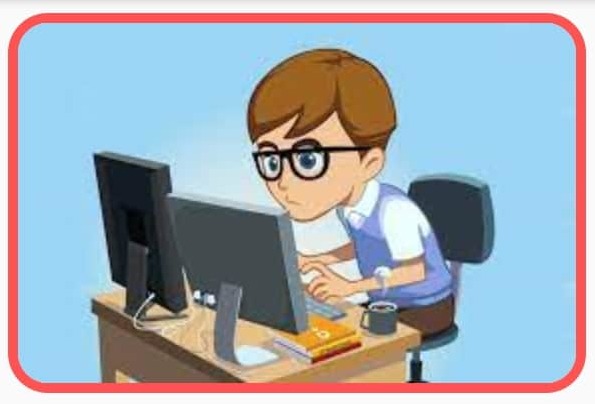
Source Code
body: SingleChildScrollView( child: Center( child: Column( children: [ SizedBox(height: 10,), Container( decoration: BoxDecoration( border: Border.all(width: 5,color: Colors.redAccent), borderRadius: BorderRadius.circular(20) ), child: ClipRRect( borderRadius: BorderRadius.circular(15), child: Image.asset('assets/img2.jpg') ), ), SizedBox(height: 10,), ], ), ), ),
Flutter Image with one side border
Some time in flutter ui designing you may need border to be set only on one side of a widget, In that case you can make border set like this:
Container( decoration: BoxDecoration( border: Border( left: BorderSide(width: 10,color: Colors.blue), // Here use border properties left, right, top, bottom ) ), child: Image.asset('assets/img3.jpg')),
Here in border use properties like left, right, top, bottom.
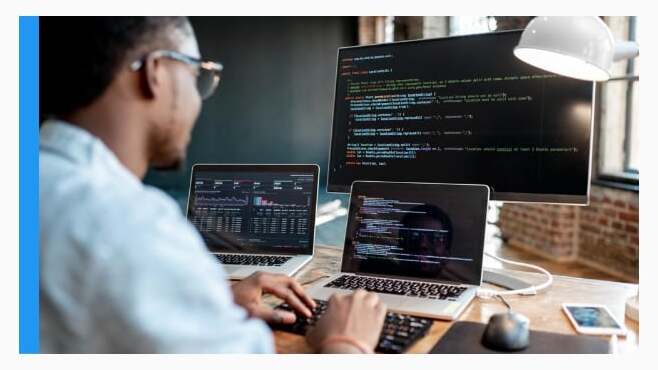
Source Code
body: SingleChildScrollView( child: Center( child: Column( children: [ Padding( padding: const EdgeInsets.all(25.0), child: Container( decoration: BoxDecoration( border: Border( left: BorderSide(width: 10,color: Colors.blue), // Here you can make use of border properties left, right, top, bottom ) ), child: Image.asset('assets/img3.jpg')), ), ], ), ), ),
Flutter Different color border on each side
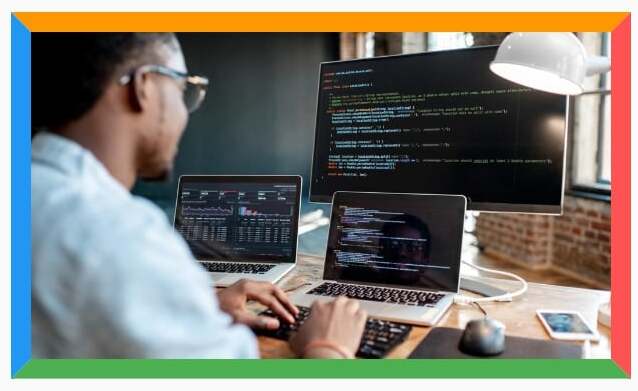
Source Code
body: SingleChildScrollView( child: Center( child: Column( children: [ SizedBox(height: 10,), Padding( padding: const EdgeInsets.all(25.0), child: Container( decoration: BoxDecoration( border: Border( left: BorderSide(width: 10,color: Colors.blue), right: BorderSide(width: 10,color: Colors.redAccent), bottom: BorderSide(width: 10,color: Colors.green), top: BorderSide(width: 10,color: Colors.orange), ) ), child: Image.asset('assets/img3.jpg')), ), ], ), ), ),