Hi Guys, Welcome to Proto Coders Point, In this Flutter Tutorial Article we will learn how to open app settings in flutter.
Flutter App_Settings Package
A Flutter package by which you can easily open Android & iOS devices settings pages such as: Open App Settings, Location Settings, device, Bluetooth Settings, WiFi settings, NFC, Battery optimization settings, Display setting, sound settings by just one button click using flutter app_settings package in your flutter project.
Video Tutorial on App Settings flutter plugin
Getting Started
App_Settings flutter package Installation
In your flutter project, open pubspec.yaml file and under dependencies section you need to add app_settings flutter library
dependencies:
app_settings: ^4.1.1
after adding the library hit pub get button on pubspec.yaml file top right or else run command in IDE terminal ‘flutter pub get’
Import it
Once you have successfully added the required package to open flutter app settings, now you need to import app_settings.dart file.
import 'package:app_settings/app_settings.dart';
Platform Configuration
Android
You can directly use system settings screen like: WIFI Settings, Security, Device Setting, date Setting etc, but in some cases, to access bluetooth setting page or Location settings page, you need to add access permission in your AndroidManifest.xml file
android/app/src/main/AndroidManifest.xml
<manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.yourname.yourapp"> <uses-permission android:name="android.permission.BLUETOOTH" /> <uses-permission android:name="android.permission.BLUETOOTH_ADMIN" /> <uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION"/> <application
iOS
TIP: If using Objective-C for iOS in your project, you will need to add use_frameworks!
to your Runner project podfile
in order to use this Swift plugin:
- target 'Runner' do use_frameworks!
Flutter App_ Setting package usage
Below are code to directly open setting page such as eg: Bluetooth Settings
Flutter Open App Setting
ElevatedButton(onPressed: (){ AppSettings.openAppSettings(); }, child: Text('Open App Setting'))
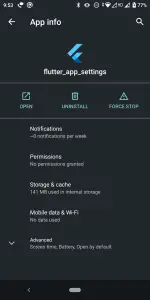
Flutter Open WiFI Setting
ElevatedButton(onPressed: (){ AppSettings.openWIFISettings(); }, child: Text('WIFI SETTING')),
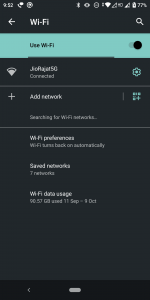
Flutter Open Location Setting
ElevatedButton(onPressed: (){ AppSettings.openLocationSettings(); }, child: Text('Location Settting')),
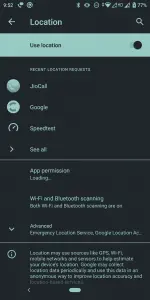
Flutter Open Bluetooth Settings
ElevatedButton(onPressed: (){ AppSettings.openBluetoothSettings(); }, child: Text('BlueTooth')),
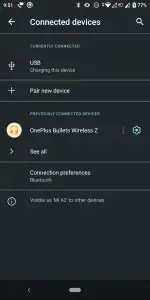
Flutter Open Device Settings
ElevatedButton(onPressed: (){ AppSettings.openDeviceSettings(); }, child: Text('Device Setting')),
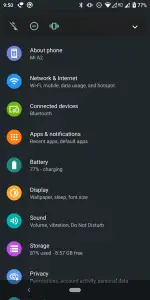
Flutter Open Notification Settings
ElevatedButton(onPressed: (){ AppSettings.openNotificationSettings(); }, child: Text('Notification Setting')),
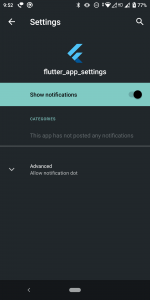
Flutter Open Battery Optimization Settings
ElevatedButton(onPressed: (){ AppSettings.openBatteryOptimizationSettings(); }, child: Text('Battery Optimization Setting'))
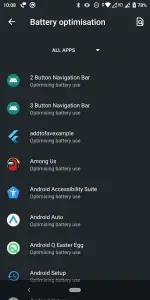
Flutter Open Internal Storage Settings
ElevatedButton(onPressed: (){ AppSettings.openInternalStorageSettings(); }, child: Text('Open Storage Setting'))
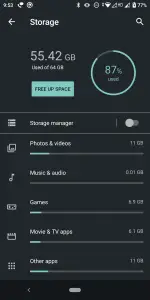