Hi Guys, Welcome to Proto Coders Point, In this Flutter Tutorial we will implement Image Slider flutter using Carousel Pro a image Slider widget library for Flutter.
DEMO
How to make Image Slider in flutter?
To show an Sliding image in flutter you need to add a library called Carousel Pro using which you can easily add image slider widget in your project, to do so follow below steps.
Step 1: Add Image Slider( carousel pro ) dependencies in your project
Open pubspec.yaml file and add the following depencencies.
dependencies: carousel_pro: ^1.0.0 // add this line
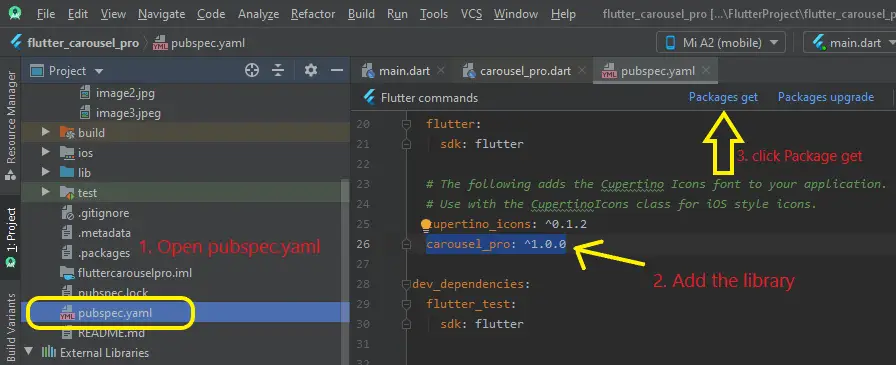
Library may come with latest feature in future so better visit official site to know about the latest version.
Step 2: Importing the carousel pro package
Then once you have added the required library depencencies in you flutter project now you can make use of the library just by importing the package of carousel pro package wherever required.
import 'package:carousel_pro/carousel_pro.dart';
Step 3: Snipped code of image slider flutter example
Carousel( images: [ AssetImage('assets/image1.jpg'), NetworkImage( 'imageurl'), AssetImage("assets/image2.jpg"), NetworkImage( "https://image.shutterstock.com/image-photo/micro-peacock-feather-hd-imagebest-260nw-1127238584.jpg"), AssetImage("assets/image3.jpeg"), NetworkImage( 'https://i.pinimg.com/originals/94/dd/57/94dd573e4b4de604ea7f33548da99fd6.jpg'), ], )
Then to show image slider we can make use of carousel widget that has a images[] properties where you can show any number of slidling images into the widget.
In the above snipped example i have make use of NetworkImage() widget to show images from internet, and AssetImage() to show images that are locally stored in Flutter project.
How to add assets and images in flutter Project
then to add image or assets under you flutter project you need to create a directory and name it as anything(in my case it assets).
Right click on your flutter project > New > Directory
give a name to you directory and there you go.
once assets directory is ready now you specify the assets directory in pubspec.yaml file so that our flutter project can easily get to know where assets image are been loacted
Now once you have added the path then you can add some images into assets folder to use then in your dart code.
Let’s come back to our main motive about this project to show image slider in flutter app.
Properties of Carousel Pro Flutter image slider library
dotSize: 4.0
dotSpacing: 15.0
dotColor: Colors.lightGreenAccent
dotIncreaseSize:2.0
dotColor: Colors.red
Here are list of the properties you can customize in Carousel Pro widget
Complete Code of Image Carousel slider in flutter using Carousel Pro Library
Just Copy paste the below code in main.dart file and here you go flutter app is ready with carousel image slider
main.dart
import 'package:flutter/material.dart'; import 'package:carousel_pro/carousel_pro.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomePage(), ); } } class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( backgroundColor: Colors.black45, appBar: AppBar( title: Text("Image Slider in FLutter "), ), body: Center( child: Column( crossAxisAlignment: CrossAxisAlignment.center, children: <Widget>[ Padding( padding: const EdgeInsets.all(15.0), child: Text( "Image Slider Example in Flutter using Carousel_Pro Library", style: TextStyle( color: Colors.blue, fontSize: 25.0, letterSpacing: 2, fontStyle: FontStyle.italic, fontWeight: FontWeight.bold), ), ), SizedBox( height: 300.0, width: double.infinity, child: Carousel( images: [ AssetImage('assets/image1.jpg'), NetworkImage( 'https://lh3.googleusercontent.com/proxy/BUNdJ4Jn2u00bBtQ_qNRLUoSGaaHsBqQzSsGnd3lRwQm6GSU8IAGlpAnRL5SWy1bx27ZZoSuOPUKA3Etyg1ND4PPO24fj5Y6a9IRHACrkbbMNf9k8fCnr3aOQJ_iWoEHcF4nwrCUDnzhKbywlPgXYamSdQ'), AssetImage("assets/image2.jpg"), NetworkImage( "https://image.shutterstock.com/image-photo/micro-peacock-feather-hd-imagebest-260nw-1127238584.jpg"), AssetImage("assets/image3.jpeg"), NetworkImage( 'https://i.pinimg.com/originals/94/dd/57/94dd573e4b4de604ea7f33548da99fd6.jpg'), ], dotColor: Colors.red, )), ], ), ), ); } }
Comments are closed.