Hi Guys, Welcome to Proto Coders Point, In this flutter tutorial we will learn how to monetize flutter app with Google Admob ads.
So, you have an flutter app published on App Store or you have completed building your flutter app & want to integrate ads into your flutter app?
Lucky, you are in a right place, I have explained the step by step process on how to get ads on your app. So let’s begin.
You need to create an Admob Account
Here is a article step by step process to create account and add app in your admob account.
- Goto Admob.com
- SignIn/SignUp with your Google Account.
- Goto App > Add App
– select, YES, if you have already published App on Appstore or NO. - if Published, then give the package name/URL of your app.
- A unique App ID will be generated for your Admob ads app.
<!-- Sample AdMob App ID: ca-app-pub-3940256099942544~3347511713 -->
- Then, Click on “create Ad Unit”.
- An Admob ad unit will be generated.
adUnitId: 'ca-app-pub-3940256099942544/6300978111'
Use this ad unit id in your flutter app to display ads in your apps.
Now, Let’s move to flutter project where you want to integrate admob ads and display ads to your app users.
How to integrate admob ads in flutter apps
1. Add dependency: Google_Mobile_Ads Package
In your flutter project open pubspec.yaml and in dependencies section add the google mobile ads package dependencies
dependencies: google_mobile_ads: ^0.13.0 # version may change check it
Important Note: project configuration required to use above google mobile ads flutter ads package.
Flutter: 1.22.0 or higher.
- Android:
Android Studio version 3.2 or higher.
Target SDK version or minSDKVersion 19 and higher.
Set CompileSdkVersion 28 or higher.
Android Gradle Plugin 4.1 or high.
2. Android Platform Setup
Admob provided App Id should be added in AndroidManifest.xml as meta-data tag inside Application Tag.
<application android:label="flutter_app_simple" android:icon="@mipmap/ic_launcher"> <!-- Sample AdMob App ID: ca-app-pub-3940256099942544~3347511713 --> <meta-data android:name="com.google.android.gms.ads.APPLICATION_ID" android:value="ca-app-pub-3940256099942544~3347511713"/> // replace App id with your app id </application>
Note: you need to replace App ID in android:value=” your app id”;
3. iOS Platform Setup
Update Info.plist
In your flutter project, navigate to “ios/Runner/Info.plist” open it & at bottom before end of </dict> add the below key and string.
<key>GADApplicationIdentifier</key> <string>ca-app-pub-3940256099942544~1458002511</string> <key>SKAdNetworkItems</key> <array> <dict> <key>SKAdNetworkIdentifier</key> <string>cstr6suwn9.skadnetwork</string> </dict> </array>
A GADApplicationIdentifierkey with string value of your admob App ID.
A SKAdNetworkItems ey with google;’s SKANetwrkIdentifier value of skadnetwork.
4. Initialize theMobile Ads SDK
Before you actual load ads & display then to app users, you must call ‘MobileAds.instance’, which loads mobile ads SDK.
MobileAds.instance.initialize();
5. Create an instance object of Ad Eg: BannerAd
A BannerAd instance should be provided with adUnitID, AdSize, AdRequest and a BannerAdListener.
BannerAd bAd = new BannerAd(size: AdSize.banner, adUnitId: 'ca-app-pub-3940256099942544/6300978111', listener: BannerAdListener( onAdClosed: (Ad ad){ print("Ad Closed"); }, onAdFailedToLoad: (Ad ad,LoadAdError error){ ad.dispose(); }, onAdLoaded: (Ad ad){ print('Ad Loaded'); }, onAdOpened: (Ad ad){ print('Ad opened'); } ), request: AdRequest());
In above replace with your own adUnitID.
Different Banner AdSize
AdSize.banner | 320 x 50 |
AdSize.largeBanner | 320 x 100 |
AdSize.mediumRectangle | 320 x 250 |
AdSize.fullBanner | 468 x 60 |
AdSize.leaderboard | 728 x 90 |
6. Show ads – Banner ads to user
Then to load and display Admob Banner ads, you can make use of AdWidget.
AdWidget( ad: bAd..load(), key: UniqueKey(), ),
Here, In Ad property you need to pass the bannerAd we have created above.
Thus this will load banner ads in your flutter app, as shown in below screenshots.
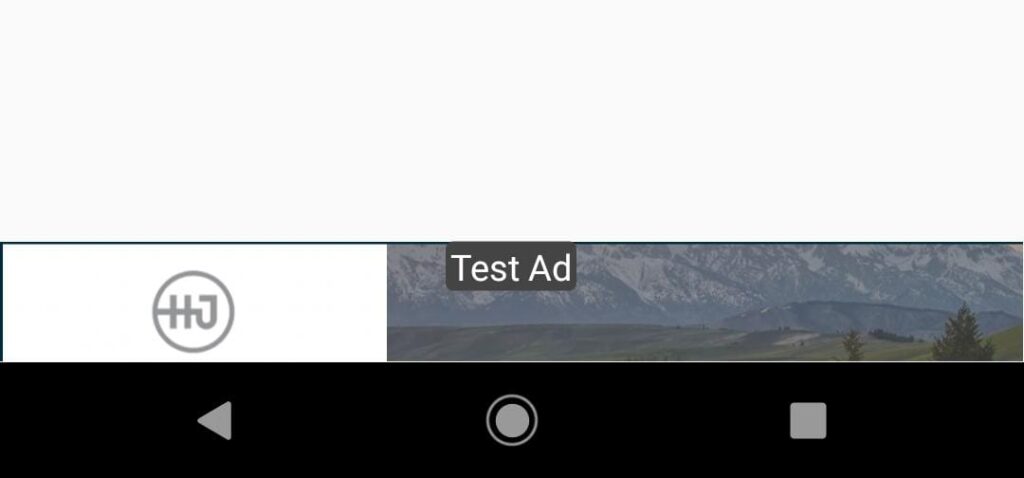
Complete Source Code – Flutter Admob Monetize – Banner Ad
AdmobHelper.dart
import 'dart:io'; import 'package:google_mobile_ads/google_mobile_ads.dart'; class AdmobHelper{ static String get bannerID => Platform.isAndroid ? 'ca-app-pub-3940256099942544/6300978111' : 'ca-app-pub-3940256099942544/6300978111'; static initialize(){ if(MobileAds.instance == null){ MobileAds.instance.initialize(); } } static BannerAd getBannerAd(){ BannerAd bAd = new BannerAd(size: AdSize.fullBanner, adUnitId: bannerID , listener: BannerAdListener( onAdClosed: (Ad ad){ print("Ad Closed"); }, onAdFailedToLoad: (Ad ad,LoadAdError error){ ad.dispose(); }, onAdLoaded: (Ad ad){ print('Ad Loaded'); }, onAdOpened: (Ad ad){ print('Ad opened'); } ), request: AdRequest()); return bAd; } }
main.dart — Only Banner ads at bottom of the screen
Video Tutorial on Monitizing flutter app with google admob — Banner Ad at bottom
import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'package:flutter_app_simple/AdmobHelper.dart'; import 'package:google_mobile_ads/google_mobile_ads.dart'; void main() { WidgetsFlutterBinding.ensureInitialized(); AdmobHelper.initialize(); runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Admob ad example', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomepage(), ); } } class MyHomepage extends StatefulWidget { @override _MyHomepageState createState() => _MyHomepageState(); } class _MyHomepageState extends State<MyHomepage> { @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Text('Admob Banner Test Ads'), ), bottomNavigationBar: Container( child: AdWidget( ad: AdmobHelper.getBannerAd()..load(), key: UniqueKey(), ), height: 50, ), ); } }
Hurry! We are all set, If the test ads are working, Real-Time abs will show into your flutter application once you publish the app into AppStore, you can sit back and relax.😎.
Showing admob banner ads randomly in between listview builder
Video tutorial on flutter listview ads
Source Code
AdmobHelper.dart
import 'package:google_mobile_ads/google_mobile_ads.dart'; class AdmobHelper { static String get bannerUnit => 'ca-app-pub-3940256099942544/6300978111'; static initialization(){ if(MobileAds.instance == null) { MobileAds.instance.initialize(); } } static BannerAd getBannerAd(){ BannerAd bAd = new BannerAd(size: AdSize.fullBanner, adUnitId: 'ca-app-pub-3940256099942544/6300978111' , listener: BannerAdListener( onAdClosed: (Ad ad){ print("Ad Closed"); }, onAdFailedToLoad: (Ad ad,LoadAdError error){ ad.dispose(); }, onAdLoaded: (Ad ad){ print('Ad Loaded'); }, onAdOpened: (Ad ad){ print('Ad opened'); } ), request: AdRequest()); return bAd; } }
main.dart
import 'dart:math'; import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'package:flutter_app_simple/AdmobHelper.dart'; import 'package:google_mobile_ads/google_mobile_ads.dart'; void main() { WidgetsFlutterBinding.ensureInitialized(); AdmobHelper.initialization(); runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Admob ad example', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomepage(), ); } } class MyHomepage extends StatefulWidget { @override _MyHomepageState createState() => _MyHomepageState(); } class _MyHomepageState extends State<MyHomepage> { late List<String> datas; // late for null safty late List<Object> dataads; // will store both data + banner ads @override void initState() { // TODO: implement initState super.initState(); datas = []; //generate array list of string for(int i = 1; i <= 20; i++){ datas.add("List Item $i"); } dataads = List.from(datas); // insert admob banner object in between the array list for(int i =0 ; i<=2; i ++){ var min = 1; var rm = new Random(); //generate a random number from 2 to 18 (+ 1) var rannumpos = min + rm.nextInt(18); //and add the banner ad to particular index of arraylist dataads.insert(rannumpos, AdmobHelper.getBannerAd()..load()); } } @override Widget build(BuildContext context) { return Scaffold( body: ListView.builder( itemCount: dataads.length, itemBuilder: (context,index){ //id dataads[index] is string show listtile with item in it if(dataads[index] is String) { return ListTile( title: Text(dataads[index].toString()), leading: Icon(Icons.exit_to_app), trailing: Icon(Icons.ice_skating), ); }else{ // if dataads[index] is object (ads) then show container with adWidget final Container adcontent = Container( child: AdWidget( ad: dataads[index] as BannerAd, key: UniqueKey(), ), height: 50, ); return adcontent; } }), bottomNavigationBar: Container( child: AdWidget( ad:AdmobHelper.getBannerAd()..load(), key: UniqueKey(), ), height: 50, ), ); } }
Output
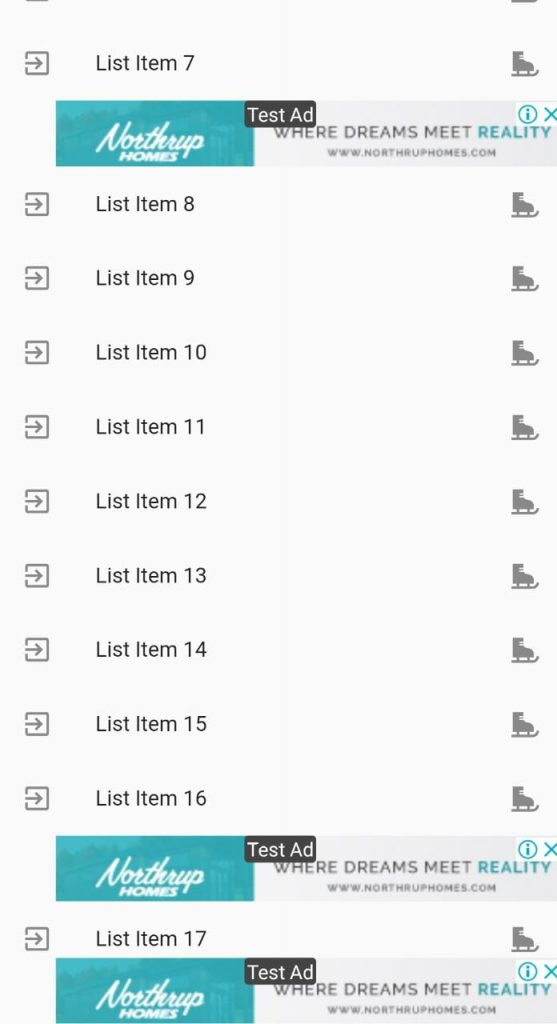
How to show Interstitial ads in flutter app
Video Tutorial
Load Interstitial ad
To Load an InterstitialAd you need to pass some parameters those are adUnitId, an AdRequest(), and an adLoadCallback:InterstitialAdLoadCallback(…..).
Below is an snippet code to load admob Interstitial Ads in flutter
InterstitialAd.load( adUnitId: 'ca-app-pub-3940256099942544/1033173712', // replace with your Admob Interstitial ad Unit ID request: AdRequest(), adLoadCallback:InterstitialAdLoadCallback( onAdLoaded: (InterstitialAd ad){ _interstitialAd = ad; }, onAdFailedToLoad: (LoadAdError error){ print('InterstitialAd failed to load: $error'); }), );
Ad Event Tracking
To track your ad unit, you can use FullScreenContentCallback, by using which you easily keep track of ads lifecycle, such as when the ad is shown or if user dismissed the ad that is shown.
_interstitialAd!.fullScreenContentCallback = FullScreenContentCallback( onAdShowedFullScreenContent: (InterstitialAd ad){ print("ad onAdshowedFullscreen"); }, onAdDismissedFullScreenContent: (InterstitialAd ad){ print("ad Disposed"); ad.dispose(); }, onAdFailedToShowFullScreenContent: (InterstitialAd ad, AdError aderror){ print('$ad OnAdFailed $aderror'); ad.dispose(); //call reload of the ads }, onAdImpression: (InterstitialAd ad) => print('$ad impression occurred.'), );
show interstitial ad to flutter app user
You can choose when to show the ad to the flutter app user, by just calling interstitial.show() method.
_interstitialAd.show();
Complete Code on how to show an interstitial ads
AdmobHelper.dart
import 'package:google_mobile_ads/google_mobile_ads.dart'; class AdmobHelper { static String get bannerUnit => 'ca-app-pub-3940256099942544/6300978111'; InterstitialAd? _interstitialAd; int num_of_attempt_load = 0; static initialization(){ if(MobileAds.instance == null) { MobileAds.instance.initialize(); } } static BannerAd getBannerAd(){ BannerAd bAd = new BannerAd(size: AdSize.fullBanner, adUnitId: 'ca-app-pub-3940256099942544/6300978111' , listener: BannerAdListener( onAdClosed: (Ad ad){ print("Ad Closed"); }, onAdFailedToLoad: (Ad ad,LoadAdError error){ ad.dispose(); }, onAdLoaded: (Ad ad){ print('Ad Loaded'); }, onAdOpened: (Ad ad){ print('Ad opened'); } ), request: AdRequest()); return bAd; } // create interstitial ads void createInterad(){ InterstitialAd.load( adUnitId: 'ca-app-pub-3940256099942544/1033173712', request: AdRequest(), adLoadCallback:InterstitialAdLoadCallback( onAdLoaded: (InterstitialAd ad){ _interstitialAd = ad; num_of_attempt_load =0; }, onAdFailedToLoad: (LoadAdError error){ num_of_attempt_load +1; _interstitialAd = null; if(num_of_attempt_load<=2){ createInterad(); } }), ); } // show interstitial ads to user void showInterad(){ if(_interstitialAd == null){ return; } _interstitialAd!.fullScreenContentCallback = FullScreenContentCallback( onAdShowedFullScreenContent: (InterstitialAd ad){ print("ad onAdshowedFullscreen"); }, onAdDismissedFullScreenContent: (InterstitialAd ad){ print("ad Disposed"); ad.dispose(); }, onAdFailedToShowFullScreenContent: (InterstitialAd ad, AdError aderror){ print('$ad OnAdFailed $aderror'); ad.dispose(); createInterad(); } ); _interstitialAd!.show(); _interstitialAd = null; } }
main.dart
import 'dart:math'; import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'package:flutter_app_simple/AdmobHelper.dart'; import 'package:google_mobile_ads/google_mobile_ads.dart'; void main() { WidgetsFlutterBinding.ensureInitialized(); AdmobHelper.initialization(); runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Admob ad example', theme: ThemeData( primarySwatch: Colors.blue, ), home: MyHomepage(), ); } } class MyHomepage extends StatefulWidget { @override _MyHomepageState createState() => _MyHomepageState(); } class _MyHomepageState extends State<MyHomepage> { late List<String> datas; // late for null safty late List<Object> dataads; // will store both data + banner ads AdmobHelper admobHelper = new AdmobHelper(); // object to access methods of AdmobHelper class @override void initState() { // TODO: implement initState super.initState(); datas = []; //generate array list of string for(int i = 1; i <= 20; i++){ datas.add("List Item $i"); } dataads = List.from(datas); // insert admob banner object in between the array list for(int i =0 ; i<=2; i ++){ var min = 1; var rm = new Random(); //generate a random number from 2 to 18 (+ 1) var rannumpos = min + rm.nextInt(18); //and add the banner ad to particular index of arraylist dataads.insert(rannumpos, AdmobHelper.getBannerAd()..load()); } } @override Widget build(BuildContext context) { return Scaffold( body: ListView.builder( itemCount: dataads.length, itemBuilder: (context,index){ //id dataads[index] is string show listtile with item in it if(dataads[index] is String) { return ListTile( title: Text(dataads[index].toString()), leading: Icon(Icons.exit_to_app), trailing: Icon(Icons.ice_skating), onTap: (){ admobHelper.createInterad(); // call create Interstitial ads }, onLongPress: (){ admobHelper.showInterad(); // call show Interstitial ads }, ); }else{ // if dataads[index] is object (ads) then show container with adWidget final Container adcontent = Container( child: AdWidget( ad: dataads[index] as BannerAd, key: UniqueKey(), ), height: 50, ); return adcontent; } }), bottomNavigationBar: Container( child: AdWidget( ad:AdmobHelper.getBannerAd()..load(), key: UniqueKey(), ), height: 50, ), ); } }
RewardedAds in Flutter
Load RewardedAd
To Load an RewardedAd you need to pass some parameters those are adUnitId, an AdRequest(), and an rewardedadLoadCallback:RewardedAdLoadCallback(…..).
Below is an snippet code to load admob Rewarded Ads in flutter
RewardedAd.load( adUnitId: 'ca-app-pub-3940256099942544/5224354917', request: AdRequest(), rewardedAdLoadCallback: RewardedAdLoadCallback( onAdLoaded: (RewardedAd ad) { print('$ad loaded.'); // Keep a reference to the ad so you can show it later. this._rewardedAd = ad; }, onAdFailedToLoad: (LoadAdError error) { print('RewardedAd failed to load: $error'); }, ));
RewardedAd Event Tracking
To track your ad unit, you can use FullScreenContentCallback, by using which you easily keep track of ads lifecycle, such as when the ad is shown or if user dismissed the ad that is shown.
_rewardedAd.fullScreenContentCallback = FullScreenContentCallback( onAdShowedFullScreenContent: (RewardedAd ad) => print('$ad onAdShowedFullScreenContent.'), onAdDismissedFullScreenContent: (RewardedAd ad) { print('$ad onAdDismissedFullScreenContent.'); ad.dispose(); }, onAdFailedToShowFullScreenContent: (RewardedAd ad, AdError error) { print('$ad onAdFailedToShowFullScreenContent: $error'); ad.dispose(); }, onAdImpression: (RewardedAd ad) => print('$ad impression occurred.'), );
show Rewarded ad to flutter app user
You can choose when to show the ad to the flutter app user, by just calling _rewardedAd.show().
_rewardedAd.show( onUserEarnedReward: (RewardedAd ad, RewardItem rewardItem) { // action to reward the app user });
Complete Code on How to show an Rewarded ads in flutter app
AdmobHelper.dart
import 'package:google_mobile_ads/google_mobile_ads.dart'; import 'package:provider/provider.dart'; import 'package:flutter/foundation.dart'; class AdmobHelper { RewardedAd _rewardedAd; static initialization() { if (MobileAds.instance == null) { MobileAds.instance.initialize(); } } void createRewardAd() { RewardedAd.load( adUnitId: 'ca-app-pub-3940256099942544/5224354917', request: AdRequest(), rewardedAdLoadCallback: RewardedAdLoadCallback( onAdLoaded: (RewardedAd ad) { print('$ad loaded.'); // Keep a reference to the ad so you can show it later. this._rewardedAd = ad; }, onAdFailedToLoad: (LoadAdError error) { print('RewardedAd failed to load: $error'); }, )); } void showRewardAd() { _rewardedAd.show( onUserEarnedReward: (RewardedAd ad, RewardItem rewardItem) { print("Adds Reward is ${rewardItem.amount}"); }); _rewardedAd.fullScreenContentCallback = FullScreenContentCallback( onAdShowedFullScreenContent: (RewardedAd ad) => print('$ad onAdShowedFullScreenContent.'), onAdDismissedFullScreenContent: (RewardedAd ad) { print('$ad onAdDismissedFullScreenContent.'); ad.dispose(); }, onAdFailedToShowFullScreenContent: (RewardedAd ad, AdError error) { print('$ad onAdFailedToShowFullScreenContent: $error'); ad.dispose(); }, onAdImpression: (RewardedAd ad) => print('$ad impression occurred.'), ); } }
RewardedAdsExample.dart
import 'package:flutter/material.dart'; import 'package:google_mobile_ads/google_mobile_ads.dart'; import 'AdmobHelper.dart'; class RewardAdsExample extends StatelessWidget { AdmobHelper admobHelper = new AdmobHelper(); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text("Reward Ads Example"), ), body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ ElevatedButton(onPressed: (){admobHelper .createRewardAd();}, child: Text("Load Reward Ads")), ElevatedButton(onPressed: (){admobHelper.showRewardAd();}, child: Text("Show Reward ads")) ], ), ), ); } }
main.dart
import 'dart:math'; import 'package:flutter/cupertino.dart'; import 'package:flutter/material.dart'; import 'package:flutter_app_simple/AdmobHelper.dart'; import 'package:flutter_app_simple/RewardAdsExample.dart'; import 'package:google_mobile_ads/google_mobile_ads.dart'; void main() { WidgetsFlutterBinding.ensureInitialized(); AdmobHelper.initialization(); runApp(MyApp()); } class MyApp extends StatelessWidget { // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( debugShowCheckedModeBanner: false, title: 'Flutter Admob ad example', theme: ThemeData( primarySwatch: Colors.blue, ), home: RewardAdsExample(), ); } }
How much does AdMob pay per 1000 impression?
Banner ads will give you very little revenue per 1000 impressions you can only earn about $0.50, but as you all know that google Admob pays only when ads are been clicked. some time with 1000 impression a day you can earn more than $2.00 with almost 5 -10 click( all depends on the location of the ads we clicked), if an app user from the USA clicks on your ads then you can easily earn around $0.30 – $1.00 per ad click, but with another country, it will be less most of the time.
while with native big ads will pay you around $1 dollor per 1000 impression.