Flutter web: Removing hash symbol (#) from all URLs
Hi Guys, Welcome to Proto Coders Point, In this Flutter tutorial article will learn how to remove # (hash symbol) from Flutter web app URL’s.
Video Tutorial – To Remove # from URL
How to remove hash symbol from flutter web URL’s
So, you are building cross-platform application using flutter framework, By Default, When you run flutter as web app, it uses the hash URL fragment strategy. The url looks like this:
http://localhost:55379/#/
You can see, The URL has # in it. This kind of hashed URL is not much used in real life. So it’s good that we remove the hash (#) symbol from flutter URL.
To Remove hash from URL, we need to set URL strategy with PathUrlStrategy(), Immediately as soon as runApp(…) is called.
Add flutter_web_plugins with sdk: flutter under dependencies section.
Firstly you need to active flutter_web_plugins sdk, To do so open pubspec.yaml file & add it under dependencies as soon below, and hit pub get.
dependencies: flutter: sdk: flutter flutter_web_plugins: // add this. sdk: flutter // even this link.
Eg: To use flutter web plugins seturlStrategy and set PathUrlStretegy() to remove # symbol from url.
// main.dart import 'package:flutter/material.dart'; import 'package:flutter_web_plugins/flutter_web_plugins.dart'; void main() { runApp(const MyApp()); setUrlStrategy(PathUrlStrategy()); }
Note/Warning:
When you import flutter_web_plugins.dart
to make use of PathUrlStrategy
method then your flutter app now will only run on Web platform, and will cause error
while running on native android & iOS mobile devices. Solution is below.
The Right way to remove hashtag (#) from URL – Flutter Web
After setting PathUrlStrategy to remove hash # from url flutter app not working on mobile devices, Here is the Solution.
Steps
1. Create 2 Config file for native & Web
In lib directory of your flutter project, create two files named as url_strategy_webConfig.dart
and url_strategy_wativeConfig.dart
.
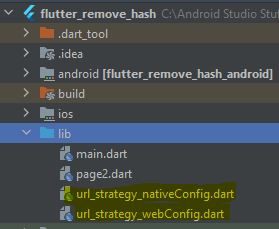
2. Code for url_strategy_webConfig.dart
import 'package:flutter_web_plugins/flutter_web_plugins.dart'; void urlConfig() { setUrlStrategy(PathUrlStrategy()); }
3. Code for url_strategy_wativeConfig.dart
void urlConfig() { // Do nothing on native platforms, As we don't need to set anything in native application }
4. Import the files in main.dart
Now import the above dart file depending on which platforms our flutter appilication.
if our flutter app in running as web application on browser then will import url_strategy_web.dart file and then apply setUrlStretegy(PathUrlStretegy()).
Code:
// main.dart import 'package:flutter/material.dart'; import './url_strategy_nativeCongif.dart' if (dart.library.html) './url_strategy_webConfig.dart'; void main() { runApp(const MyApp()); urlConfig(); }
Therefore we have successfully, learnt The right way to remove hash(#) symbol from url of flutter web application, and then solved the issuei.e. “flutter app don’t work on mobile devices after importing flutter_web_plugins.dart”.
My Complete Source Code to Remove Hash from url
main.dart
import 'package:flutter/material.dart'; import 'page2.dart'; import 'url_strategy_nativeConfig.dart' if(dart.library.html) 'url_strategy_webConfig.dart'; void main() { runApp(const MyApp()); isWebConfig(); } class MyApp extends StatelessWidget { const MyApp({Key? key}) : super(key: key); // This widget is the root of your application. @override Widget build(BuildContext context) { return MaterialApp( title: 'Flutter Demo', theme: ThemeData( primarySwatch: Colors.blue, ), routes: { '/':(context)=>MyHomePage(), '/page2': (context)=>Page2() }, ); } } class MyHomePage extends StatefulWidget { const MyHomePage({Key? key}) : super(key: key); @override State<MyHomePage> createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { @override Widget build(BuildContext context) { return Scaffold( body: Center( child: Column( mainAxisAlignment: MainAxisAlignment.center, children: [ Text("Page 1",style: TextStyle(fontSize: 18),), ElevatedButton(onPressed: (){ Navigator.of(context).pushNamed('/page2'); }, child: Text("Goto Page 2")), ], ), ), ); } }